SQL AND Operator
The SQL AND
operator is used to filter records based on multiple conditions. When using AND
in a query, each specified condition must be true for the row to be included in the result set. The AND
operator is often used in the WHERE
clause to apply more than one condition to refine search results.
In this tutorial, we will go through AND Operator in SQL, its syntax, and how to use this operator in forming conditions in SQL statements, with well detailed examples.
Syntax of SQL AND Operator
The basic syntax of the SQL AND
operator in a WHERE
clause is as follows:
SELECT column1, column2, ...
FROM table_name
WHERE condition1 AND condition2 AND ...;
Each part of this syntax has a specific purpose:
- SELECT: Specifies the columns to retrieve from the table.
- FROM: Specifies the table from which to retrieve data.
- WHERE: Introduces the conditions used to filter the data.
- AND: Combines multiple conditions. Each condition separated by
AND
must be true for a row to be included in the results.
Step-by-Step Examples with MySQL
We’ll go through various examples demonstrating the AND
operator in MySQL. Using MySQL 8.0 with MySQL Workbench, we’ll use a sample students
table with fields id
, name
, age
, grade
, and locality
.
Setup for Examples: Creating the Database and Table
1. Open MySQL Workbench and create a new database:
CREATE DATABASE school;
2. Select the school
database:
USE school;
3. Create a table named students
:
CREATE TABLE students (
id INT PRIMARY KEY AUTO_INCREMENT,
name VARCHAR(50),
age INT,
grade VARCHAR(10),
locality VARCHAR(50)
);
4. Insert some sample records to use in our examples:
INSERT INTO students (name, age, grade, locality)
VALUES
('Alice', 14, '8th', 'Northside'),
('Bob', 15, '9th', 'Westend'),
('Charlie', 14, '8th', 'Northside'),
('David', 16, '10th', 'Southend'),
('Eva', 15, '9th', 'Westend');
Examples: Using AND Operator in Queries
Now, let’s explore different scenarios of using the AND
operator with this table.
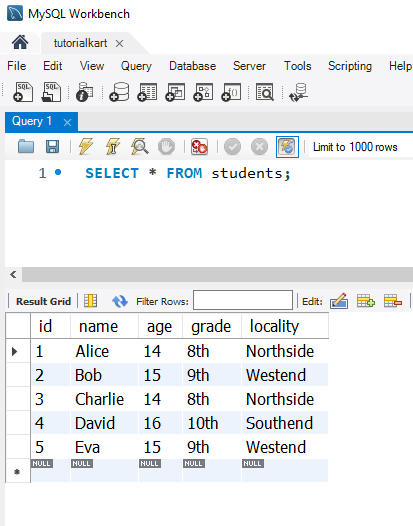
1 Selecting Rows with Two Conditions
To select students who are in the 9th grade and live in “Westend”:
SELECT * FROM students
WHERE grade = '9th' AND locality = 'Westend';
This query returns rows where both conditions are true: students who are in 9th grade and live in Westend. It displays records for Bob and Eva.
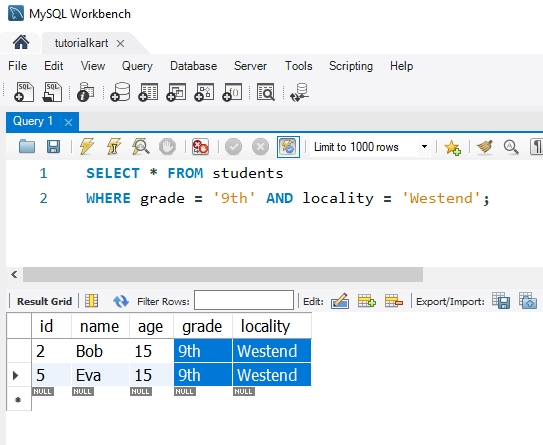
2 Using AND with Numeric Comparison
To select students who are older than 14 and in the 9th grade:
SELECT * FROM students
WHERE age > 14 AND grade = '9th';
This query returns students who are older than 14 and in the 9th grade. It displays records for Bob and Eva.
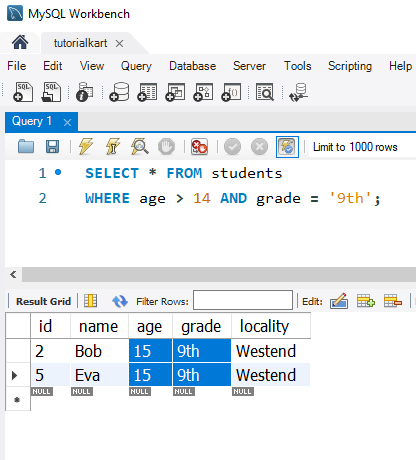
3 Using AND with Multiple Conditions
To select students who are 14 years old, in the 8th grade, and live in “Northside”:
SELECT * FROM students
WHERE age = 14 AND grade = '8th' AND locality = 'Northside';
This query only returns students who meet all three conditions, displaying records for Alice and Charlie.
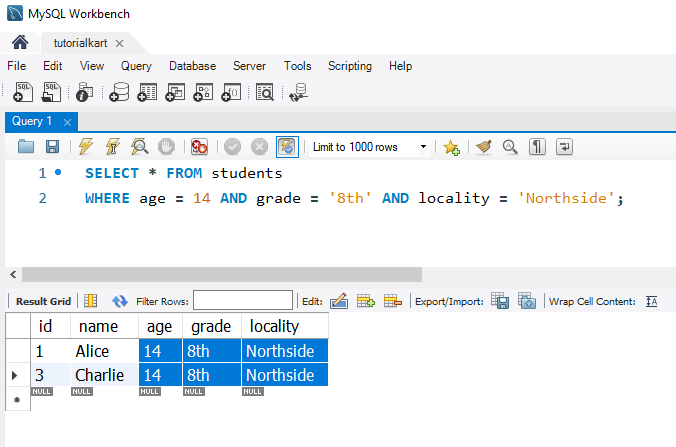
4 Using AND with Different Data Types
To select students who are named “Alice”, in the 8th grade, and live in “Northside”:
SELECT * FROM students
WHERE name = 'Alice' AND grade = '8th' AND locality = 'Northside';
This query uses a mix of text and numeric conditions, retrieving only records where all conditions are true. It will display the record for Alice only.
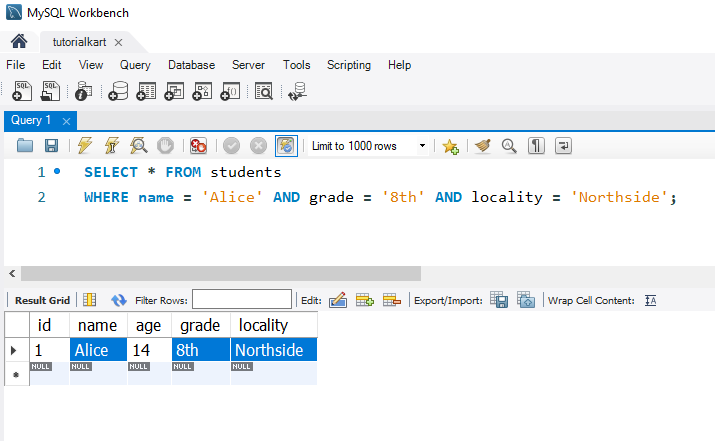
5 Using AND with Complex Conditions
To select students who are either in the 9th grade or 10th grade but also live in “Westend”:
SELECT * FROM students
WHERE (grade = '9th' OR grade = '10th') AND locality = 'Westend';
This query uses AND
with a combined OR
condition in parentheses to retrieve students who meet the specified criteria. It returns the records for Bob and Eva.
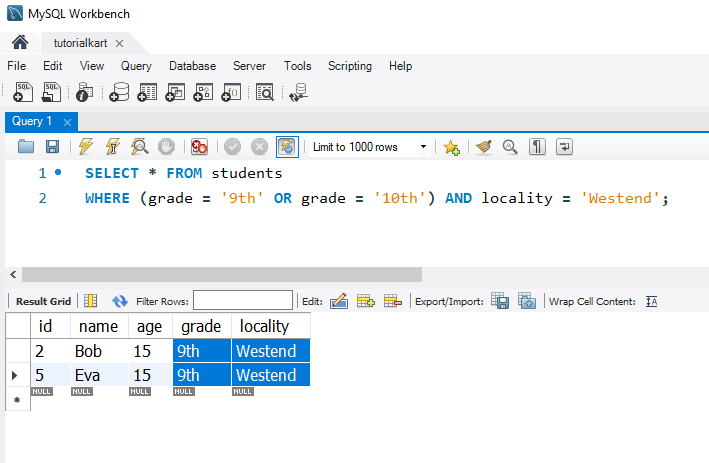
FAQs for SQL AND
1 What does the SQL AND operator do?
The AND
operator allows you to combine multiple conditions in a SQL query. All conditions separated by AND
must be true for a row to be included in the result set.
2 Can I use AND with other operators in SQL?
Yes, you can use AND
with other operators such as OR
, NOT
, and comparison operators to create complex conditions in a query.
3 How many conditions can I combine with AND?
There is no strict limit to the number of conditions you can combine with AND
, but readability may become an issue with too many conditions. Aim to keep conditions manageable.
4 What happens if one condition in an AND statement is false?
If any condition in an AND
expression is false, the entire condition evaluates to false, and the row is excluded from the result set.
5 Can AND be used with different data types in conditions?
Yes, you can use AND
with conditions involving different data types, such as numbers, strings, and dates, as long as each condition evaluates to true or false.