SQL Comparison Operators
SQL comparison operators are used to compare values in SQL queries, typically within the WHERE
clause. These operators are essential for filtering data by comparing column values against specific criteria.
In this tutorial, we will explore SQL comparison operators, their syntax, and practical examples.
List of SQL Comparison Operators
- Equal Operator
- Not Equal Operator
- Greater-than Operator
- Less-than Operator
- Greater-than or Equal Operator
- Less-than or Equal Operator
Operator | Description | Example |
---|---|---|
= | Checks if values are equal | SELECT * FROM students WHERE name = 'Arjun'; |
!= or <> | Checks if values are not equal | SELECT * FROM students WHERE name != 'Ram'; |
> | Checks if the left value is greater than the right | SELECT * FROM students WHERE age > 18; |
< | Checks if the left value is less than the right | SELECT * FROM students WHERE age < 18; |
>= | Checks if the left value is greater than or equal to the right | SELECT * FROM students WHERE age >= 18; |
<= | Checks if the left value is less than or equal to the right | SELECT * FROM students WHERE age <= 18; |
Syntax of SQL Comparison Operators
Comparison operators are used within a SQL query as follows:
</>
Copy
SELECT column_name
FROM table_name
WHERE column_name comparison_operator value;
For example, to select students older than 18:
</>
Copy
SELECT * FROM students WHERE age > 18;
Step-by-Step Examples Using SQL Comparison Operators
1 Filtering Students Based on Age and Name
We will create a students
table to demonstrate SQL comparison operators.
</>
Copy
CREATE TABLE students (
id INT PRIMARY KEY AUTO_INCREMENT,
name VARCHAR(50),
age INT,
grade VARCHAR(10)
);
Insert sample records:
</>
Copy
INSERT INTO students (name, age, grade)
VALUES
('Arjun', 17, '11th'),
('Ram', 19, '12th'),
('John', 18, '12th'),
('Roy', 16, '10th');
Now, let’s select students who are 18 years or older and have a grade of ’12th’:
</>
Copy
SELECT * FROM students
WHERE age >= 18 AND grade = '12th';
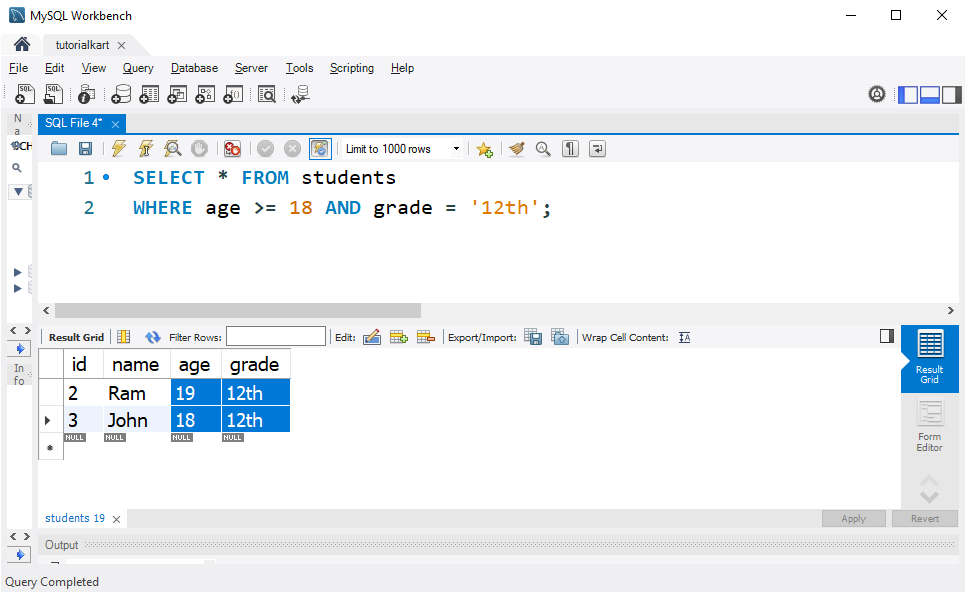
Explanation:
- The
age >= 18
condition ensures only students 18 or older are selected. - The
grade = '12th'
condition filters only students in grade 12. - This returns records for ‘Ram’ and ‘John’.