SQL Division Operator
The SQL /
(division) operator is used to divide one numeric value by another. It is commonly used in mathematical calculations within SQL queries, particularly in calculating ratios, averages, and proportions.
In this tutorial, we will explore the SQL division operator, its syntax, and practical examples demonstrating its usage.
Syntax of SQL Division Operator
The basic syntax of the SQL division operator is as follows:
SELECT column1 / column2 AS result
FROM table_name;
Alternatively, division can be performed directly on numeric values:
SELECT 20 / 4 AS result;
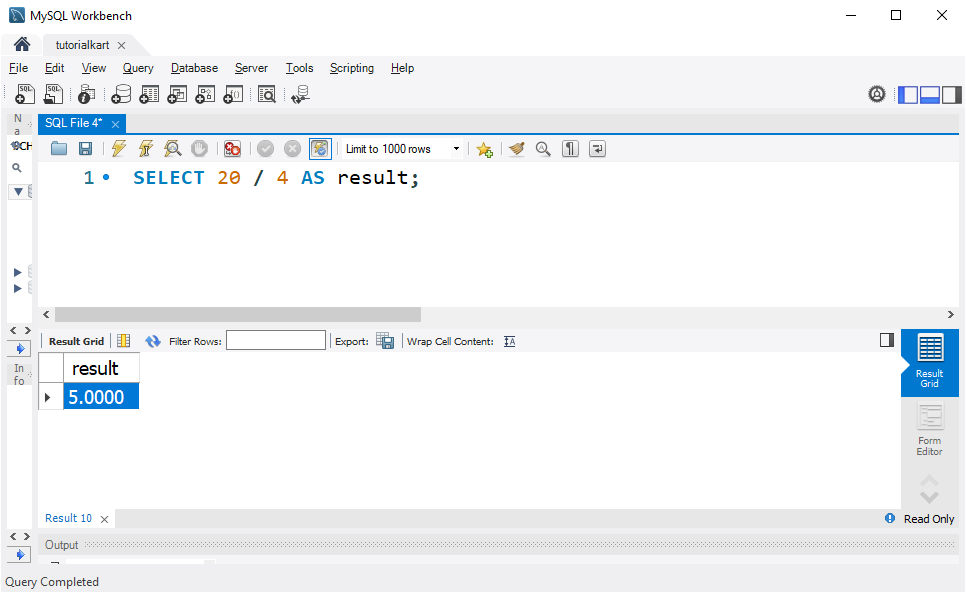
Step-by-Step Examples Using SQL Division Operator
1 Calculating Average Sales per Item
Let’s consider a sales
table that stores information about total sales and the number of items sold. We will use the division operator to calculate the average sale price per item.
CREATE TABLE sales (
id INT PRIMARY KEY AUTO_INCREMENT,
salesperson VARCHAR(50),
total_sales DECIMAL(10,2),
items_sold INT
);
Insert some sample data:
INSERT INTO sales (salesperson, total_sales, items_sold)
VALUES
('Arjun', 5000.00, 25),
('Ram', 7200.50, 36),
('John', 3400.75, 17),
('Roy', 8100.00, 45);
Now, let’s calculate the average price per item for each salesperson:
SELECT salesperson, total_sales, items_sold,
total_sales / items_sold AS avg_price_per_item
FROM sales;
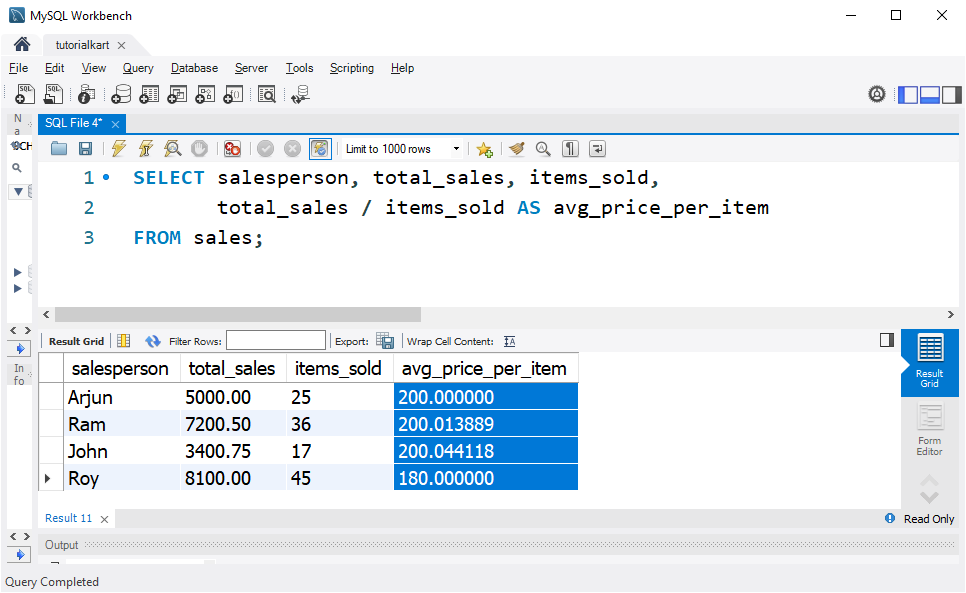
Explanation:
total_sales / items_sold
calculates the average price per item for each salesperson.- The result is displayed under the alias
avg_price_per_item
. - This helps in analyzing sales performance based on price per unit.
2 Calculating Student Score Percentage
Let’s consider a students
table where we store the total marks obtained and the maximum marks possible for each student. We will use the division operator to calculate the percentage.
CREATE TABLE students (
id INT PRIMARY KEY AUTO_INCREMENT,
name VARCHAR(50),
marks_obtained INT,
total_marks INT
);
Insert some sample data:
INSERT INTO students (name, marks_obtained, total_marks)
VALUES
('Arjun', 450, 500),
('Ram', 390, 500),
('John', 420, 500),
('Roy', 460, 500);
Now, let’s calculate the percentage of marks obtained:
SELECT name, marks_obtained, total_marks,
(marks_obtained / total_marks) * 100 AS percentage
FROM students;
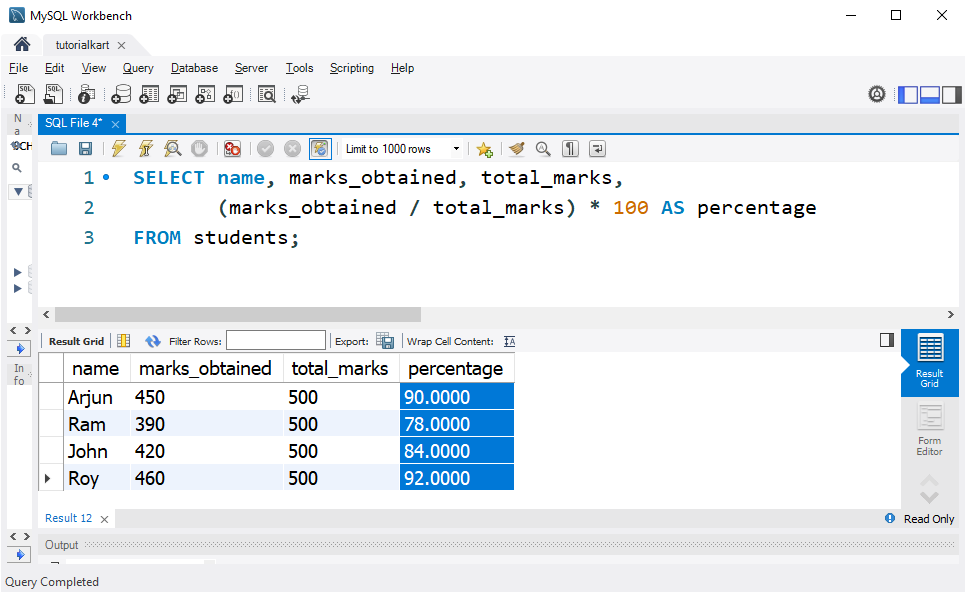
Explanation:
marks_obtained / total_marks
computes the fraction of marks obtained.- Multiplying by 100 converts the fraction into a percentage.
- This result helps in evaluating student performance in percentage format.
Handling Division by Zero
In SQL, division by zero leads to an error. To handle this, we can use the NULLIF
function, which returns NULL
if the divisor is zero:
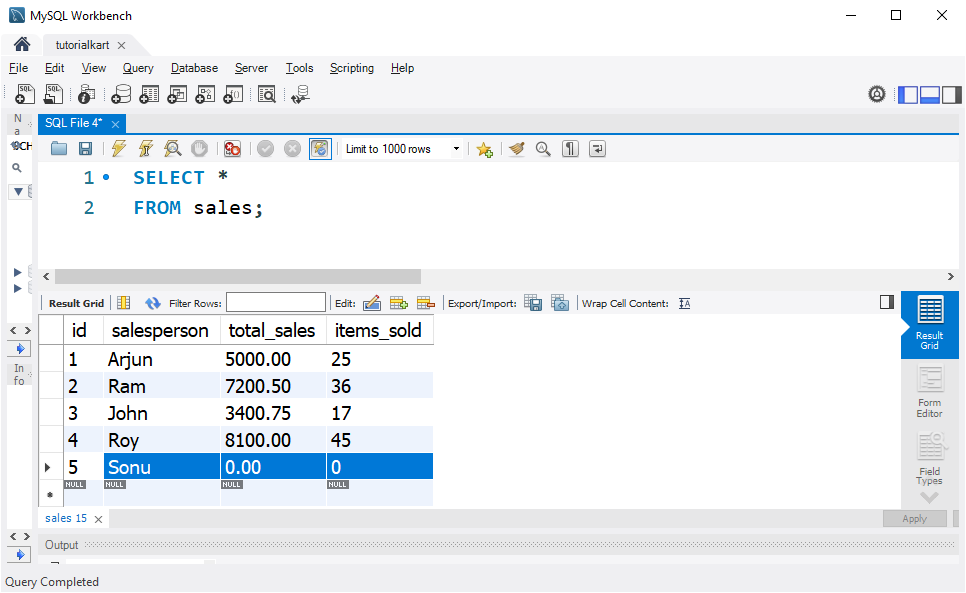
SELECT total_sales, items_sold,
total_sales / NULLIF(items_sold, 0) AS avg_price
FROM sales;
This ensures that if items_sold
is zero, the division does not cause an error but instead returns NULL
.
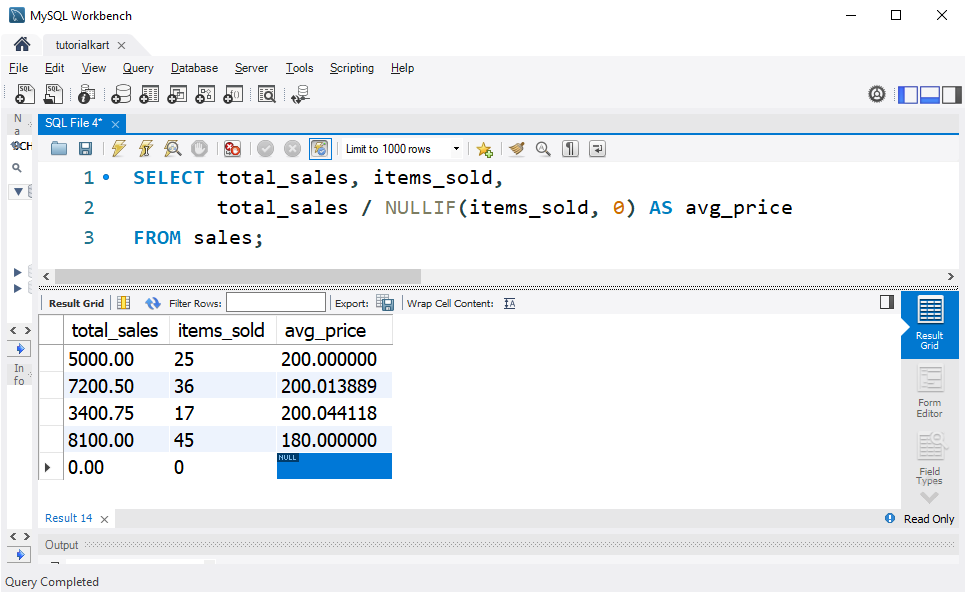
Conclusion
In this tutorial for SQL division operator (/
), we covered:
- Syntax of the SQL division operator.
- Example 1: Calculating the average sales price per item.
- Example 2: Computing student score percentages.
- Handling division by zero using
NULLIF
.