SQL Greater Than Operator
The SQL >
(greater than) operator is used to compare two values. It returns TRUE
when the left operand is greater than the right operand. This operator is commonly used in the WHERE
clause to filter results based on numerical or date-based conditions.
In this tutorial, we will explore the SQL greater than operator with syntax and practical examples.
Syntax of SQL Greater Than Operator
The basic syntax of the SQL >
operator in a WHERE
clause is:
SELECT column1, column2, ...
FROM table_name
WHERE column_name > value;
Explanation:
- SELECT: Specifies the columns to retrieve.
- FROM: Defines the table from which data is retrieved.
- WHERE: Introduces the condition for filtering.
- >: Ensures only rows where
column_name
is greater than the given value are included.
Step-by-Step Examples Using SQL Greater Than Operator
1 Filtering Students Older Than 18
Let’s create a students
table to demonstrate the >
operator.
CREATE TABLE students (
id INT PRIMARY KEY AUTO_INCREMENT,
name VARCHAR(50),
age INT
);
Insert some sample data:
INSERT INTO students (name, age)
VALUES
('Arjun', 20),
('Ram', 18),
('John', 22),
('Priya', 17);
Now, we retrieve students older than 18:
SELECT * FROM students
WHERE age > 18;
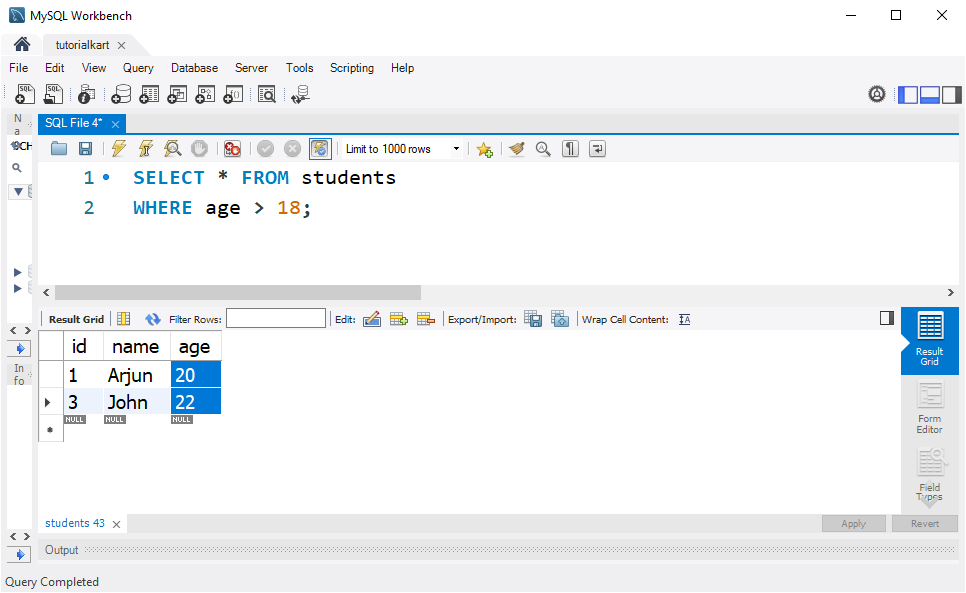
Explanation: The query returns students whose age
is greater than 18. The records for Arjun (20) and John (22) are included, while Ram (18) and Priya (17) are excluded.
2 Finding Products with Price Greater Than 500
Let’s create a products
table to filter expensive products.
CREATE TABLE products (
id INT PRIMARY KEY AUTO_INCREMENT,
name VARCHAR(50),
price DECIMAL(10,2)
);
Insert some sample data:
INSERT INTO products (name, price)
VALUES
('Laptop', 1200.00),
('Smartphone', 700.00),
('Headphones', 150.00),
('Tablet', 450.00);
Now, we retrieve products priced above 500:
SELECT * FROM products
WHERE price > 500;
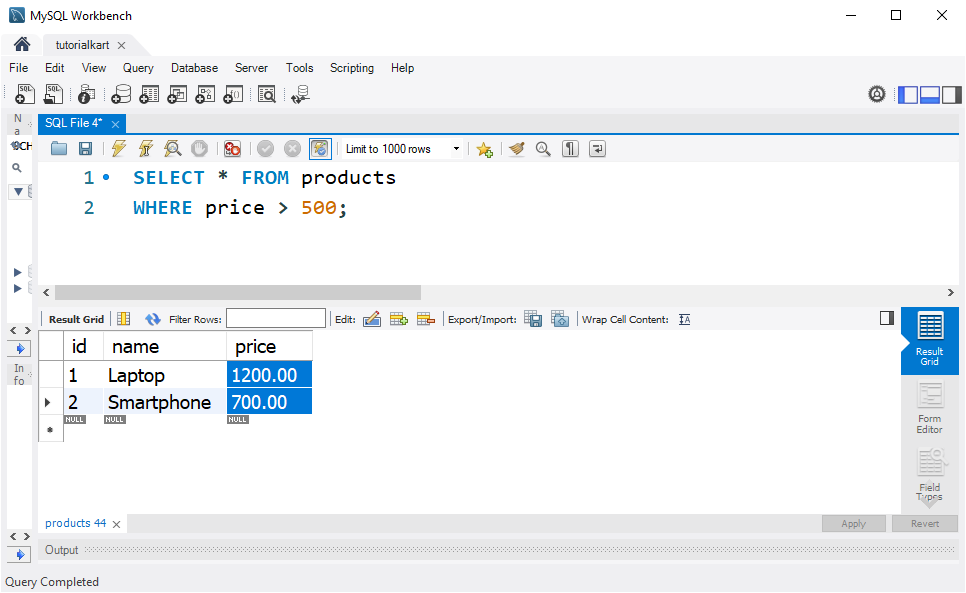
Explanation: The query filters out products where the price
is greater than 500. The Laptop (1200) and Smartphone (700) are included, while Headphones (150) and Tablet (450) are excluded.
Conclusion
In this SQL Greater than operator tutorial, we covered:
- How the
>
operator works in SQL. - The syntax and usage in queries.
- Practical examples for filtering students based on age and products based on price.