SQL Greater Than or Equal To Operator
The SQL >=
(Greater Than or Equal To) operator is used to filter records where a column value is either greater than or exactly equal to a specified value. It is commonly used in the WHERE
clause to retrieve data that meets or exceeds a certain threshold.
In this tutorial, we will cover the syntax and examples of using the >=
operator in SQL queries.
Syntax of SQL >= Operator
The basic syntax for using the >=
operator in an SQL WHERE
clause is:
SELECT column1, column2, ...
FROM table_name
WHERE column_name >= value;
Explanation:
- SELECT: Specifies the columns to retrieve.
- FROM: Defines the table from which data is fetched.
- WHERE: Filters the records based on a condition.
- >=: Ensures only records where the column value is greater than or equal to the specified value are returned.
Step-by-Step Examples Using SQL >= Operator
1 Selecting Students Aged 18 or Older
Let’s create a students
table to demonstrate the >=
operator:
CREATE TABLE students (
id INT PRIMARY KEY AUTO_INCREMENT,
name VARCHAR(50),
age INT,
grade VARCHAR(10)
);
Insert some sample data:
INSERT INTO students (name, age, grade)
VALUES
('Arjun', 17, '11th'),
('Ram', 18, '12th'),
('John', 19, '12th'),
('Priya', 16, '10th');
Now, let’s use the >=
operator to select students who are 18 years old or older:
SELECT * FROM students
WHERE age >= 18;
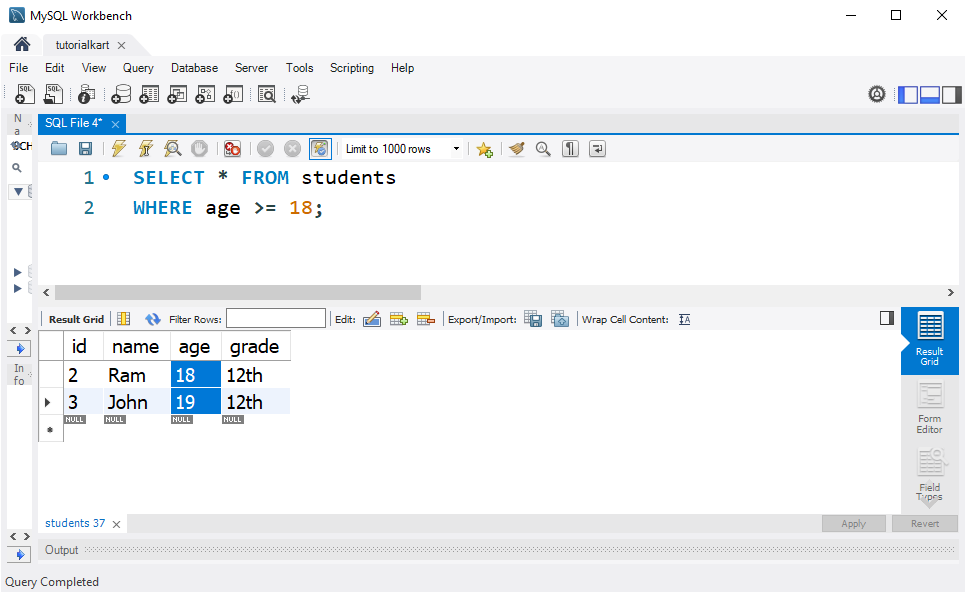
Explanation:
- This query selects students whose
age
is greater than or equal to 18. - The result will include
Ram
(18) andJohn
(19), but excludeArjun
(17) andPriya
(16).
2 Selecting Employees with Salary >= 50000
Consider an employees
table storing salary information:
CREATE TABLE employees (
id INT PRIMARY KEY AUTO_INCREMENT,
name VARCHAR(50),
salary DECIMAL(10,2),
department VARCHAR(50)
);
Insert some sample records:
INSERT INTO employees (name, salary, department)
VALUES
('Arjun', 45000, 'Finance'),
('Ram', 55000, 'HR'),
('John', 60000, 'IT'),
('Priya', 50000, 'Marketing');
Now, let’s retrieve employees earning 50000
or more:
SELECT * FROM employees
WHERE salary >= 50000;
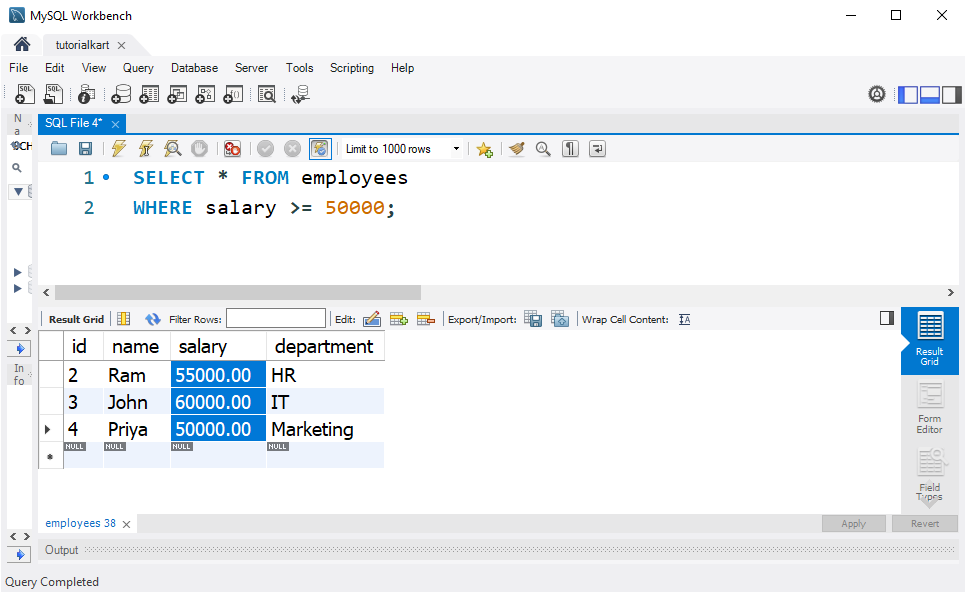
Explanation:
- The query retrieves employees with salaries greater than or equal to
50000
. - The result will include
Ram
(55000),John
(60000), andPriya
(50000), but excludeArjun
(45000).
Conclusion
In this SQL Greater Than or Equal To Operator tutorial, we covered:
- The syntax of the
>=
operator. - Example queries selecting students of age 18 or older.
- Example queries retrieving employees earning 50000 or more.