SQL Less Than or Equal To Operator
The SQL <=
(Less Than or Equal To) operator is used to compare values in a database query. It returns records where a specified column’s value is less than or equal to a given value. This operator is commonly used in the WHERE
clause to filter data based on numerical values, dates, or other comparable data types.
In this tutorial, we will explore the SQL <=
operator, its syntax, and practical use cases with examples.
Syntax of SQL Less Than or Equal To Operator
The basic syntax of the SQL <=
operator in a WHERE
clause is as follows:
SELECT column1, column2, ...
FROM table_name
WHERE column_name <= value;
Explanation:
- SELECT: Specifies the columns to retrieve from the table.
- FROM: Specifies the table from which to retrieve data.
- WHERE: Filters records based on a condition.
<=
: Ensures that only records where the column’s value is less than or equal to the specified value are retrieved.
Step-by-Step Examples Using SQL <= Operator
1 Using <= Operator on Numeric Values
Let’s create a students
table and apply the <=
operator to filter students based on age.
Step 1: Creating the Table
CREATE TABLE students (
id INT PRIMARY KEY AUTO_INCREMENT,
name VARCHAR(50),
age INT,
grade VARCHAR(10)
);
Insert some sample data:
INSERT INTO students (name, age, grade)
VALUES
('Arjun', 14, '8th'),
('Ram', 15, '9th'),
('John', 16, '10th'),
('Priya', 13, '7th');
Step 2: Selecting Students Aged 14 or Below
SELECT * FROM students
WHERE age <= 14;
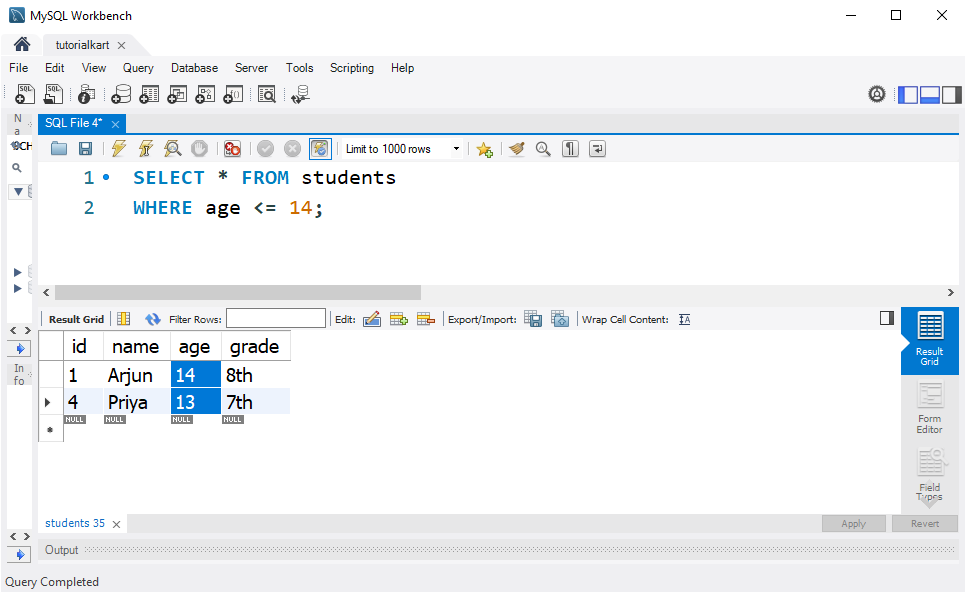
Explanation:
- The query retrieves students with
age
values that are less than or equal to 14. - It will return the records for Arjun (14) and Priya (13).
2 Using <= Operator on Date Values
Let’s create an orders
table and filter orders placed before or on a specific date.
Step 1: Creating the Table
CREATE TABLE orders (
id INT PRIMARY KEY AUTO_INCREMENT,
customer_name VARCHAR(50),
order_date DATE,
amount DECIMAL(10,2)
);
Insert some sample data:
INSERT INTO orders (customer_name, order_date, amount)
VALUES
('Arjun', '2024-02-05', 250.50),
('Ram', '2024-02-10', 100.00),
('John', '2024-02-15', 500.75),
('Priya', '2024-02-20', 150.25);
Step 2: Selecting Orders Placed on or Before February 10, 2024
SELECT * FROM orders
WHERE order_date <= '2024-02-10';
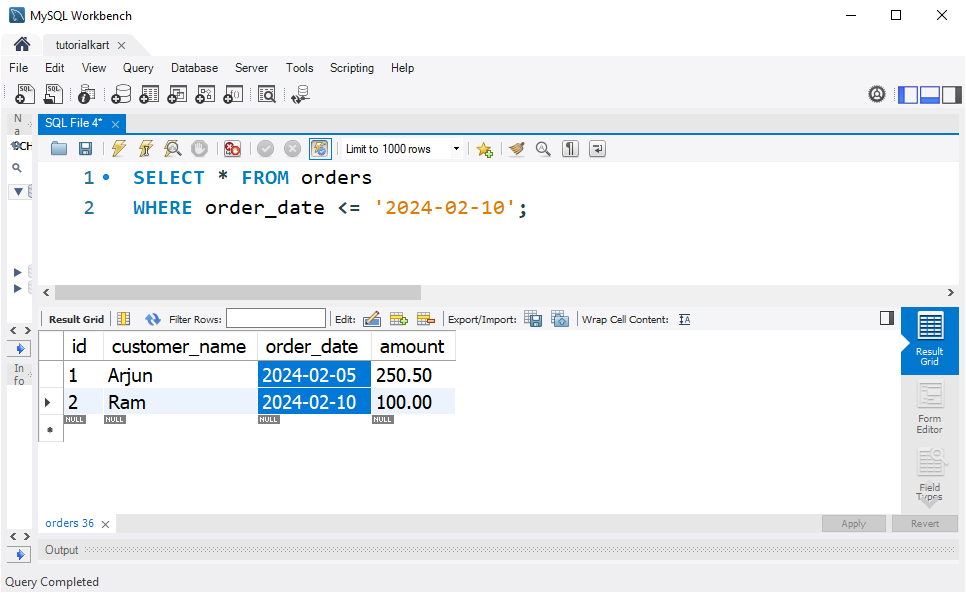
Explanation:
- The query filters records where
order_date
is on or before2024-02-10
. - It returns orders for Arjun (2024-02-05) and Ram (2024-02-10).
Conclusion
In this SQL Less Than or Equal To operator tutorial, we covered:
- The syntax of the
<=
operator. - How to use it with numerical values.
- How to apply it to date values.