SQL NOT EQUAL Operator
The SQL NOT EQUAL
operator is used to filter records that do not match a specified value. In SQL, the !=
or <>
operators can be used interchangeably to represent “not equal to”. This operator is commonly used in the WHERE
clause to exclude specific values from query results.
In this tutorial, we will explore the NOT EQUAL
operator in SQL with syntax explanations and practical examples.
Syntax of SQL NOT EQUAL != Operator
The basic syntax for using the NOT EQUAL
operator in a WHERE
clause is as follows:
SELECT column1, column2, ...
FROM table_name
WHERE column_name != value;
Alternatively, you can use the <>
operator:
SELECT column1, column2, ...
FROM table_name
WHERE column_name <> value;
Explanation:
- SELECT: Specifies the columns to retrieve from the table.
- FROM: Specifies the table from which data is selected.
- WHERE: Filters records based on conditions.
- != or <>: Ensures that the values in the column are not equal to the specified value.
Step-by-Step Examples Using SQL NOT EQUAL != Operator
1 Filtering Employees Who Are Not from a Specific Department
Let’s create an employees
table to demonstrate the NOT EQUAL operator.
CREATE TABLE employees (
id INT PRIMARY KEY AUTO_INCREMENT,
name VARCHAR(50),
department VARCHAR(50),
salary DECIMAL(10,2)
);
Insert some sample data:
INSERT INTO employees (name, department, salary)
VALUES
('Arjun', 'IT', 75000),
('Ram', 'HR', 60000),
('John', 'Finance', 80000),
('Priya', 'IT', 72000),
('David', 'Marketing', 65000);
Now, let’s fetch employees who are NOT in the IT department:
SELECT * FROM employees
WHERE department != 'IT';
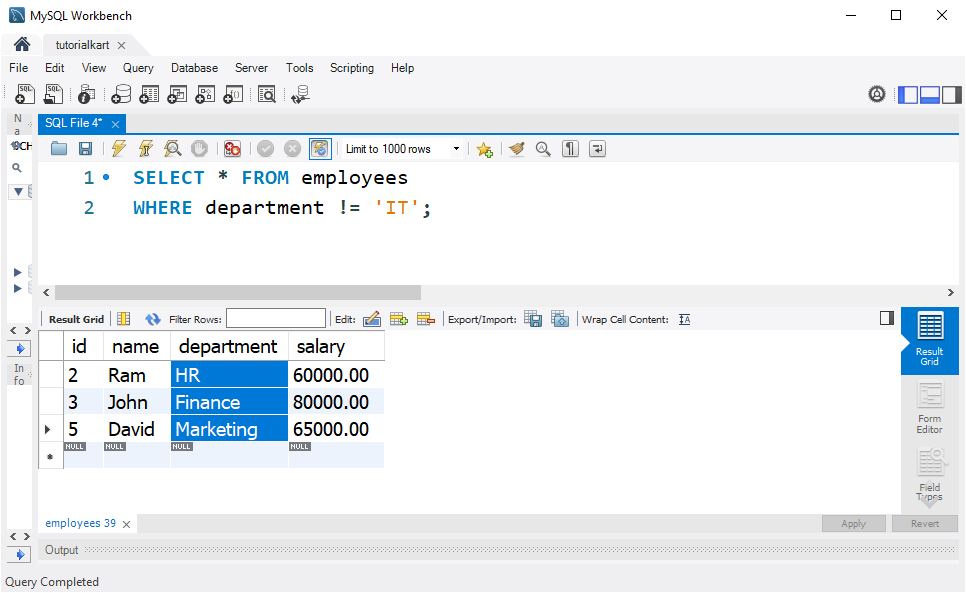
Explanation:
- The query selects all employees whose department is not “IT”.
- Arjun and Priya belong to IT, so they are excluded from the results.
- Only employees from HR, Finance, and Marketing are displayed.
2 Selecting Customers Who Are Not from a Specific City
Let’s create a customers
table to filter out customers who do not belong to a certain city.
CREATE TABLE customers (
id INT PRIMARY KEY AUTO_INCREMENT,
name VARCHAR(50),
city VARCHAR(50),
total_spent DECIMAL(10,2)
);
Insert some sample data:
INSERT INTO customers (name, city, total_spent)
VALUES
('Arjun', 'Mumbai', 1200.50),
('Ram', 'Delhi', 800.00),
('John', 'Bangalore', 1500.75),
('Priya', 'Mumbai', 950.25),
('David', 'Chennai', 1100.60);
Now, let’s fetch customers who are NOT from Mumbai:
SELECT * FROM customers
WHERE city <> 'Mumbai';
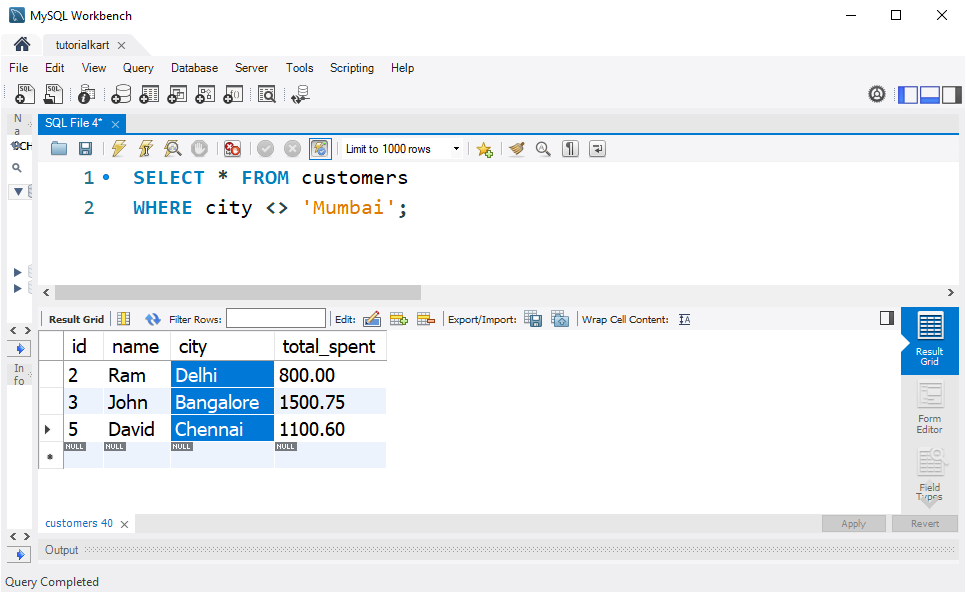
Explanation:
- The query selects all customers whose city is not “Mumbai”.
- Arjun and Priya are from Mumbai, so they are excluded from the results.
- The result will include customers from Delhi, Bangalore, and Chennai.
Conclusion
In this SQL Not Equal operator tutorial, we covered:
- The syntax for using the
!=
and<>
operators. - Using
NOT EQUAL
inWHERE
clauses to exclude certain records. - Examples of filtering employees and customers based on criteria.