SwiftUI Assets
In SwiftUI, assets are resources such as images, colors, and data files that are bundled with your app. You can manage these assets to ensure a consistent design, optimal performance, and seamless adaptation across different platforms like iOS, macOS, watchOS, and tvOS.
The Asset Catalog in Xcode allows you to organise and utilise these assets effortlessly.
In this tutorial, we’ll explore how to add, manage, and use assets in a SwiftUI project, including examples for images and colors.
What Are Assets?
Assets in SwiftUI projects include:
- Images: Used for icons, backgrounds, or UI elements.
- Colors: Custom colors for text, backgrounds, or components.
- Data Files: JSON, plist, or other files bundled with the app.
These resources are stored in the Assets.xcassets folder within your Xcode project, allowing for centralised management.
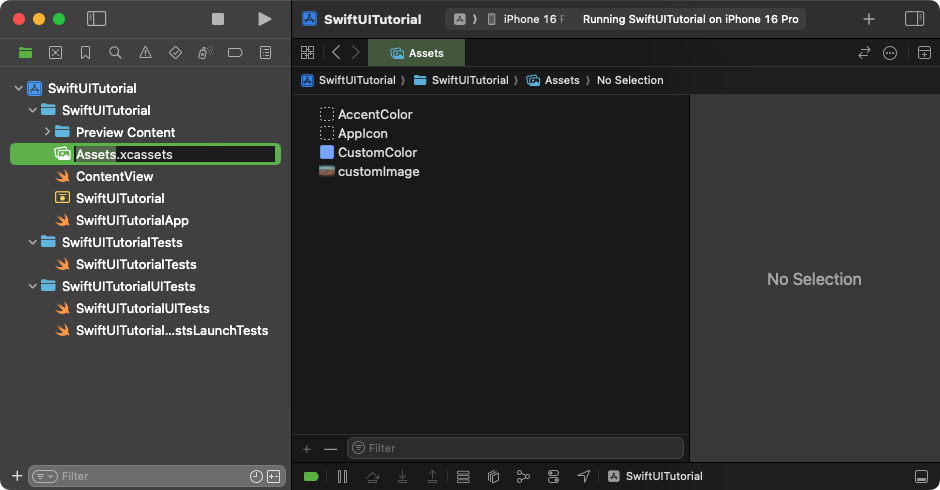
How to Add Assets in Xcode
Follow these steps to add assets to your SwiftUI project:
Step 1
Open your Xcode project.
Step 2
Navigate to the Assets.xcassets folder in the Project Navigator.
Step 3
Adding Image into Assets
To add an image, drag and drop your image file into the Assets folder.
Adding Color Set into Assets
To create a custom color, click the +
button at the bottom of the Asset Catalog, select Color Set, and define the color.
Once added, these assets can be referenced in your SwiftUI code.
Using Assets in SwiftUI
SwiftUI provides simple and declarative ways to use assets like images and colors. Let’s explore examples for both.
Example 1: Using Images
This example demonstrates how to display an image from the asset catalog:
Code Example:
import SwiftUI
struct ContentView: View {
var body: some View {
VStack {
Text("SwiftUI Assets")
.font(.title)
.padding()
Image("sampleImage") // Reference the image by its name in the Assets folder
.resizable()
.scaledToFit()
.frame(width: 200, height: 200)
}
}
}
Xcode Screenshot:
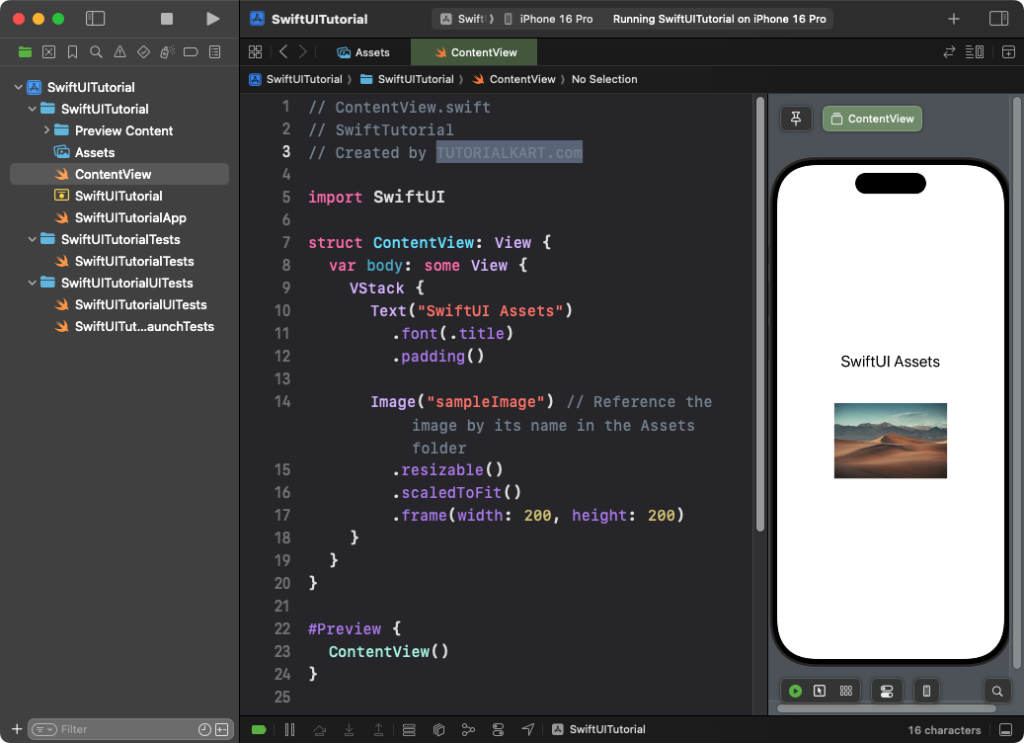
Explanation:
- The
Image("sampleImage")
loads an image named “SampleImage” from the asset catalog. - The
.resizable()
modifier makes the image scalable. - The
.scaledToFit()
modifier ensures the image maintains its aspect ratio.
Result: The image is displayed in a scalable and well-proportioned format.
Example 2: Using Custom Colors
This example shows how to use custom colors defined in the asset catalog:
Code Example:
import SwiftUI
struct ContentView: View {
var body: some View {
VStack {
Text("Hello, SwiftUI!")
.font(.headline)
.foregroundColor(Color("PrimaryColor")) // Use a custom color from Assets
.padding()
Rectangle()
.fill(Color("SecondaryColor")) // Apply the custom color to a shape
.frame(width: 200, height: 100)
}
}
}
Xcode Screenshot:
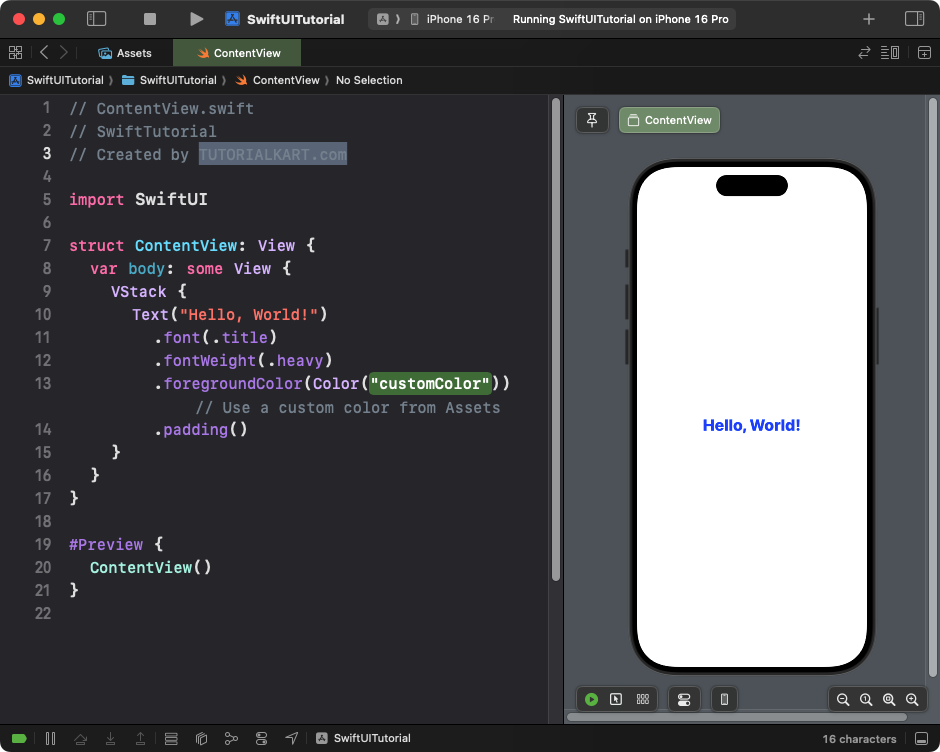
Explanation:
- The
Color("customColor")
references a custom color named “customColor” in the asset catalog. - Custom colors make it easy to maintain consistency across your app.
Result: The text uses colors defined in the asset catalog.
Example 3: Dynamic Image Assets
This example shows how to use dynamic image assets that adapt to light and dark mode:
Code Example:
import SwiftUI
struct ContentView: View {
var body: some View {
VStack {
Image("DynamicImage")
.resizable()
.scaledToFit()
.frame(width: 200, height: 200)
}
}
}
Explanation:
- Create an image set in the asset catalog and configure separate images for light and dark mode.
- The system automatically selects the appropriate image based on the current mode.
Result: The image adapts dynamically to light and dark mode, ensuring a consistent user experience.
Advantages of Using Assets in SwiftUI
Organizing and using assets in SwiftUI offers several benefits:
- Centralized Management: All resources are stored in a single location for easy access.
- Dynamic Adaptation: Images and colors adapt automatically to light and dark mode.
- Consistency: Custom colors ensure a uniform design language across the app.
- Performance Optimization: Assets are optimized for different device resolutions and platforms.
Assets streamline the development process while enhancing the visual appeal of your app.
Conclusion
SwiftUI assets are used for creating visually consistent and adaptable applications. By using images, colors, and other resources in the asset catalog, you can ensure a cohesive design that scales seamlessly across Apple’s ecosystem. With the examples in this tutorial, you can effectively use assets to enhance your app’s appearance and user experience.