SwiftUI Image Link
In SwiftUI, you can make an image clickable by wrapping it in a Link. This is particularly useful when you want to create interactive UI elements, such as clickable banners or thumbnails that open a URL in a browser.
In this tutorial, we will go through an example on how to create an image as a link, so that when user clicks on it, navigates to the specified link.
When to Use Clickable Image Links
Clickable image links are useful in scenarios like:
- Creating promotional banners that lead to external websites.
- Designing interactive galleries or navigation menus.
- Improving user experience by making content visually appealing and functional.
Syntax to Create Image Links
Here’s the basic syntax for making an image a clickable link:
Link(destination: URL(string: "https://example.com")!) {
Image("imageName")
.resizable()
.scaledToFit()
}
Examples to Create Image Links
In the following examples, we demonstrate how to create image links using Link
.
Example 1: Image Link to a Website
In this example, clicking the image opens a specified URL in the browser.
File: ContentView.swift
// ContentView.swift
// Created by TUTORIALKART.com
import SwiftUI
struct ContentView: View {
var body: some View {
VStack {
ImageLinkToWebsiteView()
}
}
}
struct ImageLinkToWebsiteView: View {
var body: some View {
Link(destination: URL(string: "https://example.com")!) {
Image("sampleImage")
.resizable()
.scaledToFit()
.frame(width: 250)
}
.padding()
}
}
#Preview {
ContentView()
}
Detailed Explanation
- Link: Wraps the image and specifies the URL to navigate to when clicked.
- Image: Displays the image named
sampleImage
from the app’s assets. - .resizable: Ensures the image can scale dynamically.
- .scaledToFit: Maintains the image’s aspect ratio within the frame.
- .frame: Sets the width of the image to 250 points for better visibility.
Output Screenshots
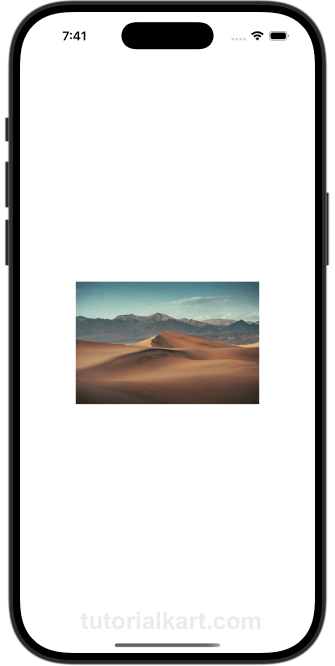
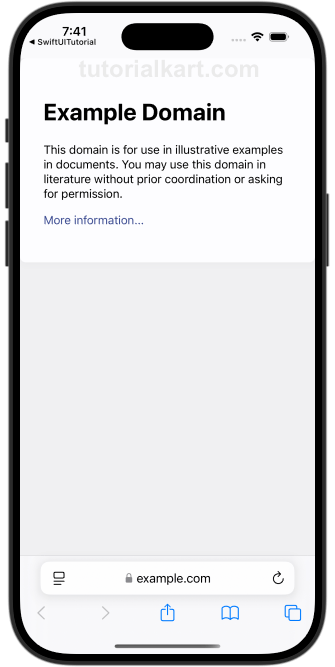
Output Video
Conclusion
Making images clickable in SwiftUI is straightforward and enhances user interaction. Whether linking to external websites or navigating within your app, the Link
and Button
modifiers provide flexible and powerful options.