SwiftUI Image Opacity
In SwiftUI, the Image view allows you to control the transparency of an image using the .opacity()
modifier. Adjusting opacity is a simple yet powerful technique for creating layered effects, emphasizing content, or adding subtle visual enhancements to your UI.
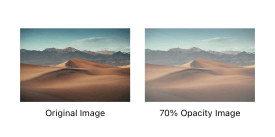
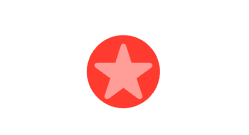
In this tutorial, we will guide you through applying opacity to images in SwiftUI with examples.
When to Use Image Opacity
Controlling image opacity is useful in scenarios like:
- Creating overlay effects in a user interface.
- Highlighting or de-emphasizing certain elements.
- Blending images with other visual components.
Syntax to Adjust Image Opacity
Here’s the basic syntax for adjusting an image’s opacity:
Image("imageName")
.opacity(opacityValue)
Here, opacityValue
is a Double
ranging from 0.0
(completely transparent) to 1.0
(fully opaque).
Examples to Adjust Image Opacity
In the following examples, we demonstrate how to apply opacity to a system image and a custom image.
Example 1: Layering Images with Opacity
In this example, we place one system image over another, with the top image partially transparent, creating a layered effect.
File: ContentView.swift
// ContentView.swift
// Created by TUTORIALKART.com
import SwiftUI
struct ContentView: View {
var body: some View {
VStack {
SystemImageOpacityView()
}
}
}
struct SystemImageOpacityView: View {
var body: some View {
ZStack {
Image(systemName: "circle.fill")
.resizable()
.scaledToFit()
.frame(width: 100, height: 100)
.foregroundColor(.red)
Image(systemName: "star.fill")
.resizable()
.scaledToFit()
.frame(width: 80, height: 80)
.foregroundColor(.white)
.opacity(0.5)
}
.padding()
}
}
#Preview {
ContentView()
}
Detailed Explanation
- ZStack: Layers the circle and star images on top of each other.
- Circle Image: Acts as the background, fully opaque and blue.
- Star Image: Positioned over the circle with a white tint and 50% opacity. Since the background circle image is red, the star image is 50% transparent, we get to see a light shade of red transparent through the star image.
Output

Example 2: Comparing Original and Transparent Images
In this example, we display both the original and the partially transparent versions of a custom image side by side.
File: ContentView.swift
// ContentView.swift
// Created by TUTORIALKART.com
import SwiftUI
struct ContentView: View {
var body: some View {
VStack {
CustomImageOpacityView()
}
}
}
struct CustomImageOpacityView: View {
var body: some View {
HStack(spacing: 20) {
VStack {
Image("sampleImage")
.resizable()
.scaledToFit()
.frame(width: 150)
Text("Original Image")
.font(.caption)
}
VStack {
Image("sampleImage")
.resizable()
.scaledToFit()
.frame(width: 150)
.opacity(0.7)
Text("70% Opacity Image")
.font(.caption)
}
}
.padding()
}
}
#Preview {
ContentView()
}
Detailed Explanation
- HStack: Aligns the original and transparent images horizontally.
- Original Image: Displays the image without any opacity modifier.
- Transparent Image: Displays the same image with 70% opacity.
Output

Conclusion
Adjusting the opacity of images in SwiftUI is a straightforward way to create subtle visual effects and improve design aesthetics. Using the .opacity()
modifier, you can easily control the transparency of system and custom images to fit your app’s requirements.