SwiftUI Image Rotate
In SwiftUI, the Image
view allows you to display and manipulate images in your app. Rotating an image is a simple yet powerful feature to add dynamic and visually appealing elements to your user interface. You can apply rotation transformations using the .rotationEffect()
modifier or animate the rotation for interactive effects.
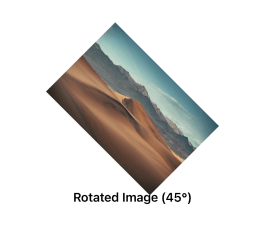
In this tutorial, we will guide you through rotating images in SwiftUI with two examples.
When to Rotate an Image
Rotating images is useful in scenarios like:
- Creating interactive animations for user engagement.
- Customizing the appearance of images to fit a specific design style.
- Building dynamic visual effects for gaming or creative applications.
Syntax to Rotate an Image
Here’s a basic syntax for rotating an image using the .rotationEffect()
modifier:
Image("imageName")
.rotationEffect(.degrees(angleInDegrees))
Examples to Rotate an Image
In the following examples, we take sampleImage.jpg and demonstrate two ways to rotate an image: using a fixed rotation angle and applying an animated rotation.
Example 1: Rotating an Image with a Fixed Angle
In this example, we apply a static rotation angle to an image using the .rotationEffect()
modifier. This is useful for simple transformations where the rotation does not change dynamically.
File: ContentView.swift
import SwiftUI
struct ContentView: View {
var body: some View {
VStack {
Image("sampleImage")
.resizable()
.scaledToFit()
.frame(width: 200, height: 200)
.rotationEffect(.degrees(45))
Text("Rotated Image (45°)")
.font(.headline)
}
.padding()
}
}
#Preview {
ContentView()
}
Detailed Explanation
- Image: The
Image
view loads an image namedsampleImage
from the app’s assets. - .resizable(): Makes the image scalable.
- .scaledToFit(): Ensures the image retains its aspect ratio within the specified dimensions.
- .rotationEffect: Rotates the image by 45 degrees around its center point.
Output
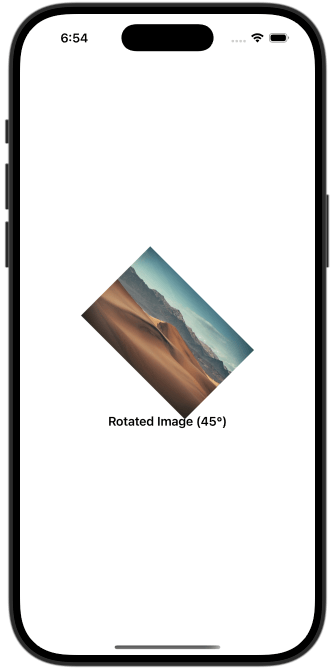
Example 2: Rotating an Image with Animation
In this example, we create an interactive animation where the image rotates continuously. This approach is ideal for creating dynamic and engaging visuals.
File: ContentView.swift
// ContentView.swift
// Created by TUTORIALKART.com
import SwiftUI
struct ContentView: View {
var body: some View {
VStack {
CustomImageOpacityView()
}
}
}
struct CustomImageOpacityView: View {
var body: some View {
HStack(spacing: 20) {
VStack {
Image("sampleImage")
.resizable()
.scaledToFit()
.frame(width: 150)
Text("Original Image")
.font(.caption)
}
VStack {
Image("sampleImage")
.resizable()
.scaledToFit()
.frame(width: 150)
.opacity(0.7)
Text("70% Opacity Image")
.font(.caption)
}
}
.padding()
}
}
#Preview {
ContentView()
}
Detailed Explanation
- Image: Displays an image named
sampleImage
from the app’s assets. - @State rotationAngle: Keeps track of the rotation angle for animation.
- .rotationEffect: Applies the current rotation angle to the image.
- .animation: Animates the rotation with a linear progression over 2 seconds, repeating indefinitely.
- .onAppear: Triggers the animation when the view appears by setting the rotation angle to 360 degrees.
Output
Conclusion
Rotating images in SwiftUI is a versatile technique that enhances user interfaces with dynamic visual effects. Whether applying a fixed rotation or animating the transformation, the .rotationEffect()
modifier provides the flexibility to achieve your design goals.