SwiftUI Image Rounded Border
In SwiftUI, the Image
view provides a way to display images and customize their appearance. Adding a rounded border to an image enhances its visual appeal and aligns it with modern design trends. Using the .clipShape()
and .overlay()
modifiers, you can easily create rounded borders around your images.
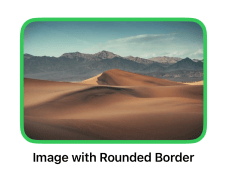
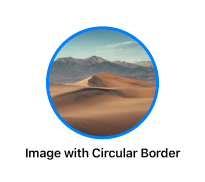
In this tutorial, we will demonstrate how to create simple and rounded borders with examples.
When to Use Rounded Borders
Rounded borders are useful in scenarios like:
- Improving the aesthetics of your app’s user interface.
- Creating a modern, clean, and soft appearance for images.
- Highlighting or emphasizing certain images in a visually appealing way.
Syntax to Add Rounded Borders to Images
Here’s a basic syntax for adding a rounded border using .clipShape()
and .overlay()
:
Image("imageName")
.clipShape(RoundedRectangle(cornerRadius: radius))
.overlay(
RoundedRectangle(cornerRadius: radius)
.stroke(Color.borderColor, lineWidth: borderWidth)
)
Examples to Add Rounded Borders
In the following examples, we demonstrate how to add simple and rounded borders to images.
Example 1: Adding a Rounded Border
In this example, we add a rounded border to an image by clipping it to a rounded rectangle and overlaying a border.
File: ContentView.swift
// ContentView.swift
// Created by TUTORIALKART.com
import SwiftUI
struct ContentView: View {
var body: some View {
VStack {
RoundedImageBorderView()
}
}
}
struct RoundedImageBorderView: View {
var body: some View {
VStack {
Image("sampleImage")
.resizable()
.scaledToFit()
.frame(width: 250)
.clipShape(RoundedRectangle(cornerRadius: 20))
.overlay(
RoundedRectangle(cornerRadius: 20)
.stroke(Color.green, lineWidth: 5)
)
Text("Image with Rounded Border")
.font(.headline)
}
.padding()
}
}
#Preview {
ContentView()
}
Detailed Explanation
- Image: Loads the
sampleImage
from the app’s assets and ensures it is resizable. - .clipShape: Clips the image to the shape of a rounded rectangle with a 20-point corner radius.
- .overlay: Adds a green stroke border of 5 points around the rounded rectangle.
- .frame: Sets the width of the image to 250 points while maintaining its aspect ratio.
Output
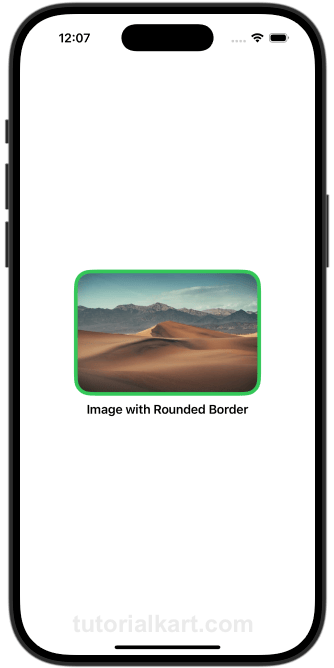
Example 2: Adding a Circular Border
In this example, we create a circular border around an image by clipping it to a circle and overlaying a border.
File: ContentView.swift
// ContentView.swift
// Created by TUTORIALKART.com
import SwiftUI
struct ContentView: View {
var body: some View {
VStack {
CircularImageBorderView()
}
}
}
struct CircularImageBorderView: View {
var body: some View {
VStack {
Image("sampleImage")
.resizable()
.scaledToFit()
.frame(height: 150)
.clipShape(Circle())
.overlay(
Circle()
.stroke(Color.blue, lineWidth: 4)
)
Text("Image with Circular Border")
.font(.headline)
}
.padding()
}
}
#Preview {
ContentView()
}
Detailed Explanation
- Image: Loads the
sampleImage
from the app’s assets and ensures it is resizable. - .clipShape: Clips the image to a circular shape.
- .overlay: Adds a blue stroke border of 4 points around the circular image.
- .frame: Sets the dimensions of the image to 150×150 points.
Output

Conclusion
Adding rounded borders to images in SwiftUI is a versatile way to enhance visual appeal. Whether using rounded rectangles or circular borders, these techniques help you create modern and polished designs for your app.