SwiftUI LazyHStack
In SwiftUI, the LazyHStack
is a horizontally scrolling container that efficiently arranges its child views in a horizontal stack. Unlike HStack, which loads all child views at once, the LazyHStack
only loads views that are currently visible, making it ideal for large data sets and optimised performance.
This tutorial will cover how to use LazyHStack
with examples to demonstrate its use cases, such as working with static and dynamic content.
Basic Syntax of LazyHStack
The basic syntax of LazyHStack
is as follows:
LazyHStack {
Content1()
Content2()
Content3()
}
Here:
LazyHStack
: A horizontal stack that lazily loads child views.Content1, Content2, Content3
: The views arranged inside the stack.
The LazyHStack
works well with large or dynamic content, as it loads only the views currently visible on the screen.
Examples of LazyHStack
Let’s explore practical examples of using LazyHStack
in SwiftUI.
Example 1: Basic LazyHStack with Static Content
This example demonstrates a simple LazyHStack
with static views arranged horizontally.
Code Example:
import SwiftUI
struct ContentView: View {
var body: some View {
ScrollView(.horizontal) {
LazyHStack(spacing: 20) {
ForEach(1...10, id: \.self) { index in
Text("Item \(index)")
.frame(width: 100, height: 100)
.background(Color.blue.opacity(0.3))
.cornerRadius(10)
}
}
.padding()
}
}
}
Xcode Screenshot:
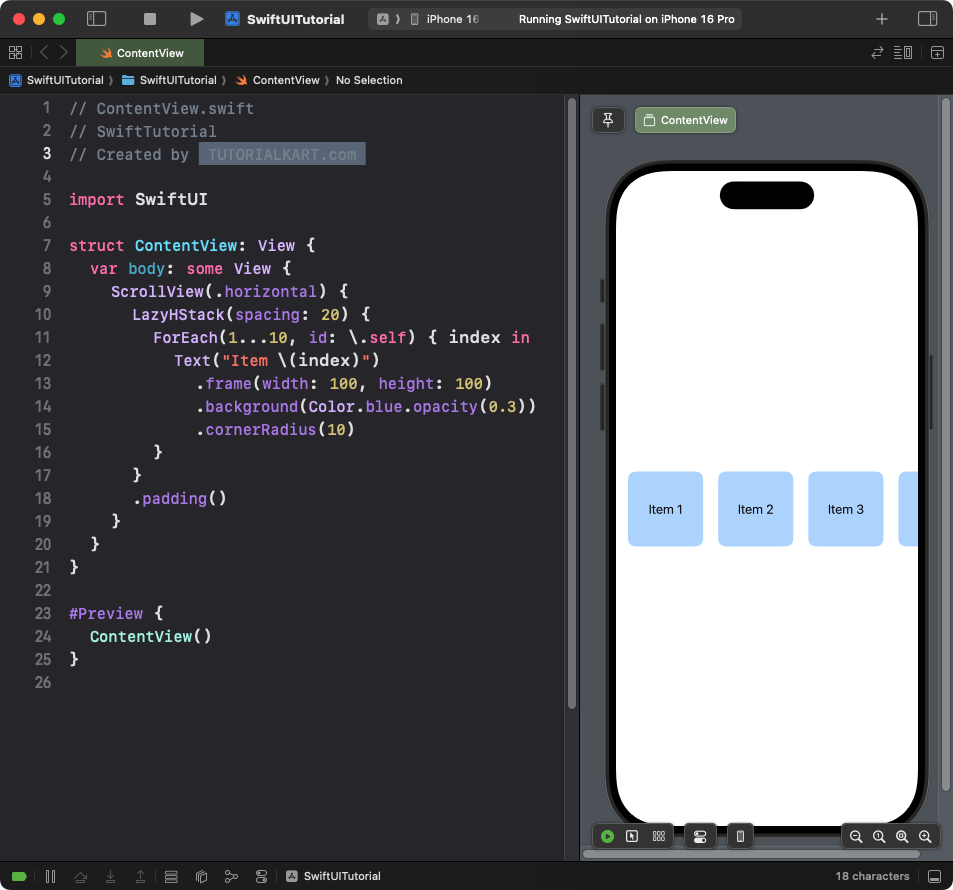
Explanation:
- The
ScrollView(.horizontal)
wraps theLazyHStack
to allow horizontal scrolling. Refer ScrollView. - The
ForEach
generates ten text items dynamically. - The items are styled with a blue background and rounded corners.
Result: A horizontal scrollable list with 10 items, arranged in a lazy manner.
Example 2: LazyHStack with Images
This example arranges images inside a horizontally scrollable LazyHStack
.
Code Example:
import SwiftUI
struct ContentView: View {
let imageNames = ["star", "heart", "circle", "square", "triangle"]
var body: some View {
ScrollView(.horizontal) {
LazyHStack(spacing: 15) {
ForEach(imageNames, id: \.self) { name in
Image(systemName: name)
.resizable()
.scaledToFit()
.frame(width: 80, height: 80)
.foregroundColor(.purple)
.background(Color.gray.opacity(0.2))
.cornerRadius(10)
}
}
.padding()
}
}
}
Xcode Screenshot:
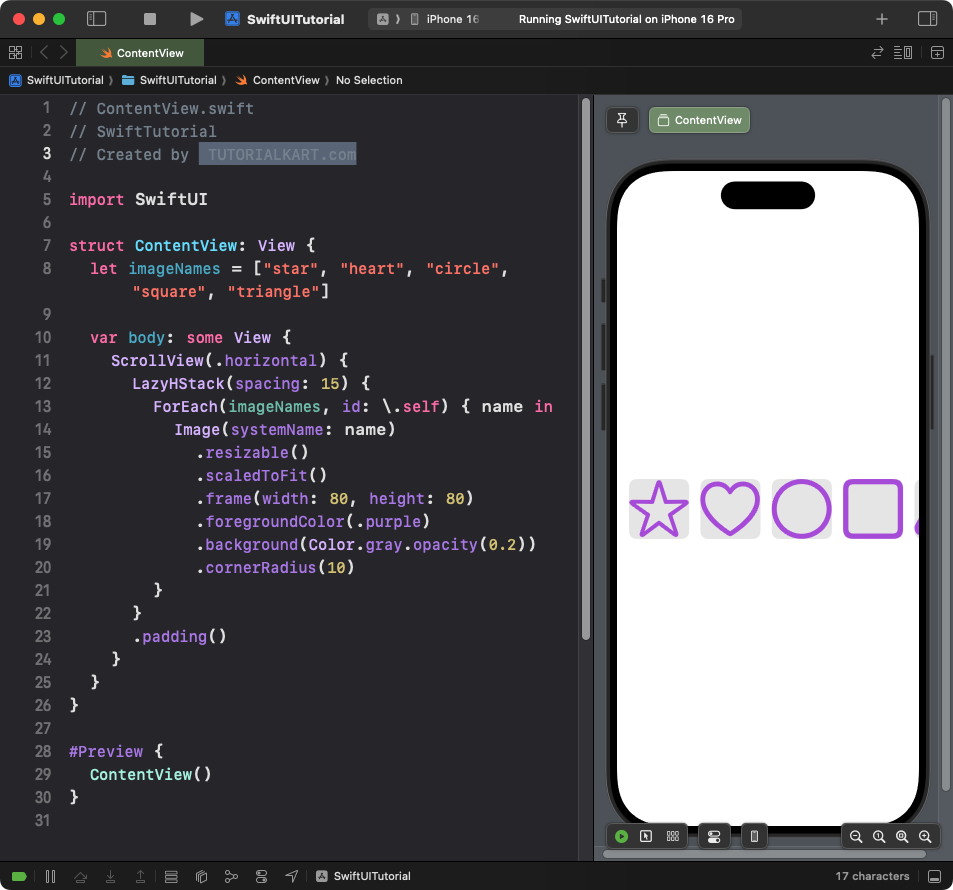
Explanation:
- The
ScrollView
enables horizontal scrolling. - The
LazyHStack
arranges the SF Symbols images horizontally. - Each image is styled with a background color, rounded corners, and a fixed frame size.
Result: A scrollable row of images styled with a gray background and purple icons.
Example 3: LazyHStack with Dynamic Content
This example demonstrates how to load a large number of dynamic items efficiently using LazyHStack
.
Code Example:
import SwiftUI
struct ContentView: View {
let items = Array(1...100)
var body: some View {
ScrollView(.horizontal) {
LazyHStack(spacing: 10) {
ForEach(items, id: \.self) { item in
Text("Item \(item)")
.frame(width: 80, height: 80)
.background(Color.orange.opacity(0.7))
.cornerRadius(8)
}
}
.padding()
}
}
}
Xcode Screenshot:
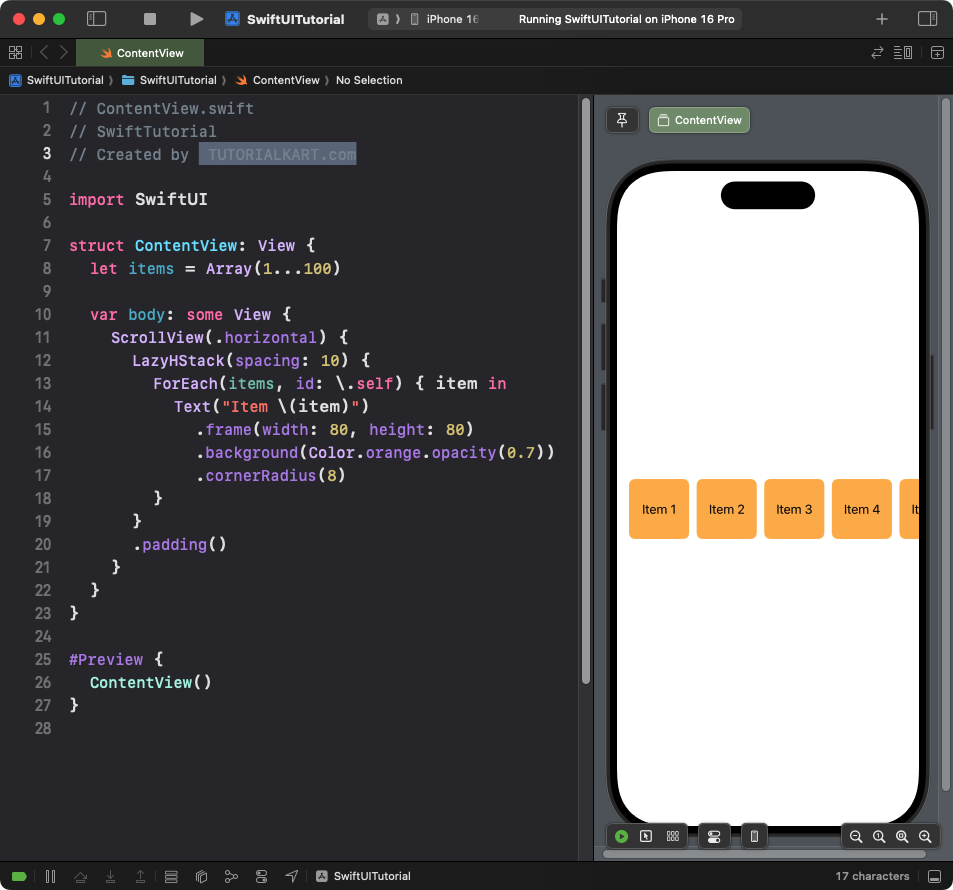
Explanation:
- The
items
array contains 100 numbers to simulate a large dataset. - The
LazyHStack
only loads the views that are visible on the screen, improving performance. - Each item is styled with a fixed frame and orange background.
Result: A scrollable list of 100 items arranged horizontally, but only visible items are loaded lazily.
Conclusion
SwiftUI’s LazyHStack
is a powerful tool for efficiently displaying horizontal content, especially for large or dynamic data sets. By combining it with ScrollView
, you can create scrollable, performant, and visually appealing layouts in your app.