SwiftUI LazyVGrid
In SwiftUI, the LazyVGrid
is a layout container that arranges views in a grid, organised vertically. The LazyVGrid
loads its child views lazily, meaning it only creates views that are currently visible on the screen. This makes it an efficient choice for large datasets.
In this tutorial, we’ll explore the basics of using LazyVGrid
, customise its layout, and provide examples for dynamic content.
Basic Syntax of LazyVGrid
The basic syntax of LazyVGrid
involves defining the grid layout using an array of GridItem
objects:
LazyVGrid(columns: [GridItem(.flexible()), GridItem(.flexible())]) {
// Add your views here
}
Here:
columns
: An array ofGridItem
objects defining the layout of columns in the grid.GridItem(.flexible())
: Configures the grid to distribute the available space equally among columns.
Examples of LazyVGrid
Let’s look at examples that demonstrate the usage and customization of LazyVGrid
.
Example 1: Basic LazyVGrid
This example creates a simple grid with two columns, displaying numbers:
Code Example:
import SwiftUI
struct ContentView: View {
let items = Array(1...20)
var body: some View {
ScrollView {
LazyVGrid(columns: [GridItem(.flexible()), GridItem(.flexible())], spacing: 20) {
ForEach(items, id: \.self) { item in
Text("Item \(item)")
.frame(width: 100, height: 100)
.background(Color.blue.opacity(0.5))
.cornerRadius(10)
}
}
.padding()
}
}
}
Xcode Screenshot:
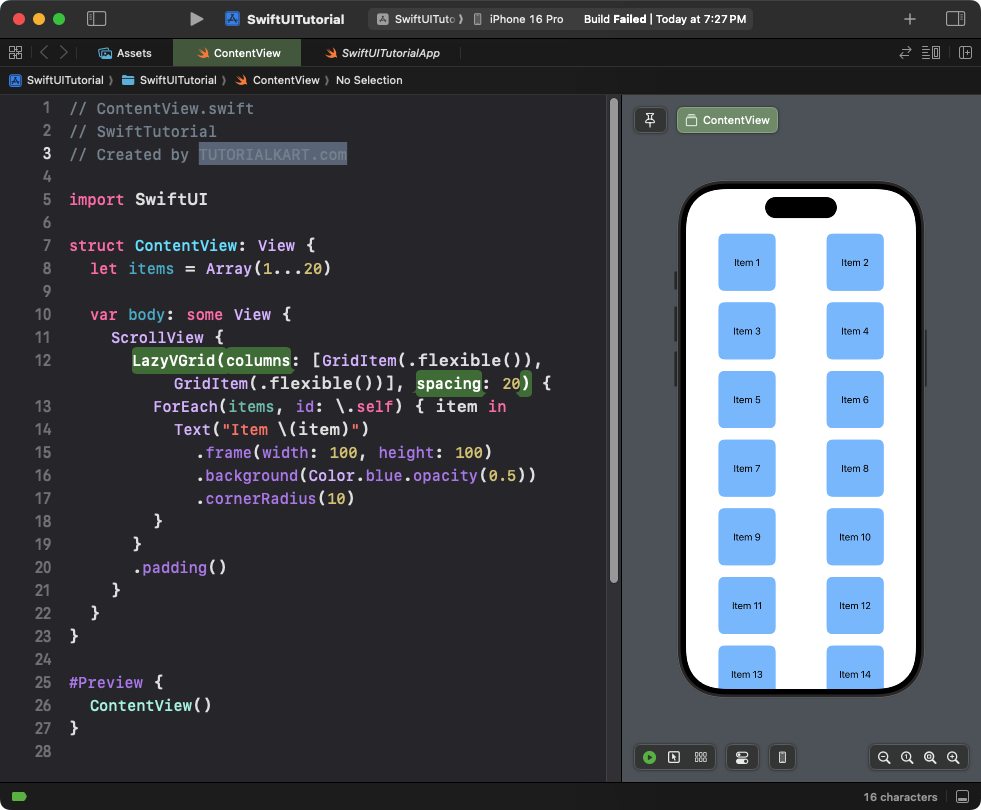
Explanation:
- The
LazyVGrid
arranges the items into two columns. - The
ForEach
loop generates 20 items dynamically. - Each item is styled with a blue background and rounded corners.
Result: A scrollable grid with 20 items, divided into two columns.
Example 2: LazyVGrid with Dynamic Content
This example shows how to populate the grid dynamically with user input:
Code Example:
import SwiftUI
struct ContentView: View {
@State private var items = ["Item 1", "Item 2", "Item 3"]
var body: some View {
VStack {
ScrollView {
LazyVGrid(columns: [GridItem(.flexible()), GridItem(.flexible())], spacing: 10) {
ForEach(items, id: \.self) { item in
Text(item)
.frame(height: 50)
.background(Color.pink.opacity(0.5))
.cornerRadius(8)
}
}
.padding()
}
Button("Add Item") {
items.append("Item \(items.count + 1)")
}
.padding()
.background(Color.blue)
.foregroundColor(.white)
.cornerRadius(8)
}
}
}
Xcode Screenshot:
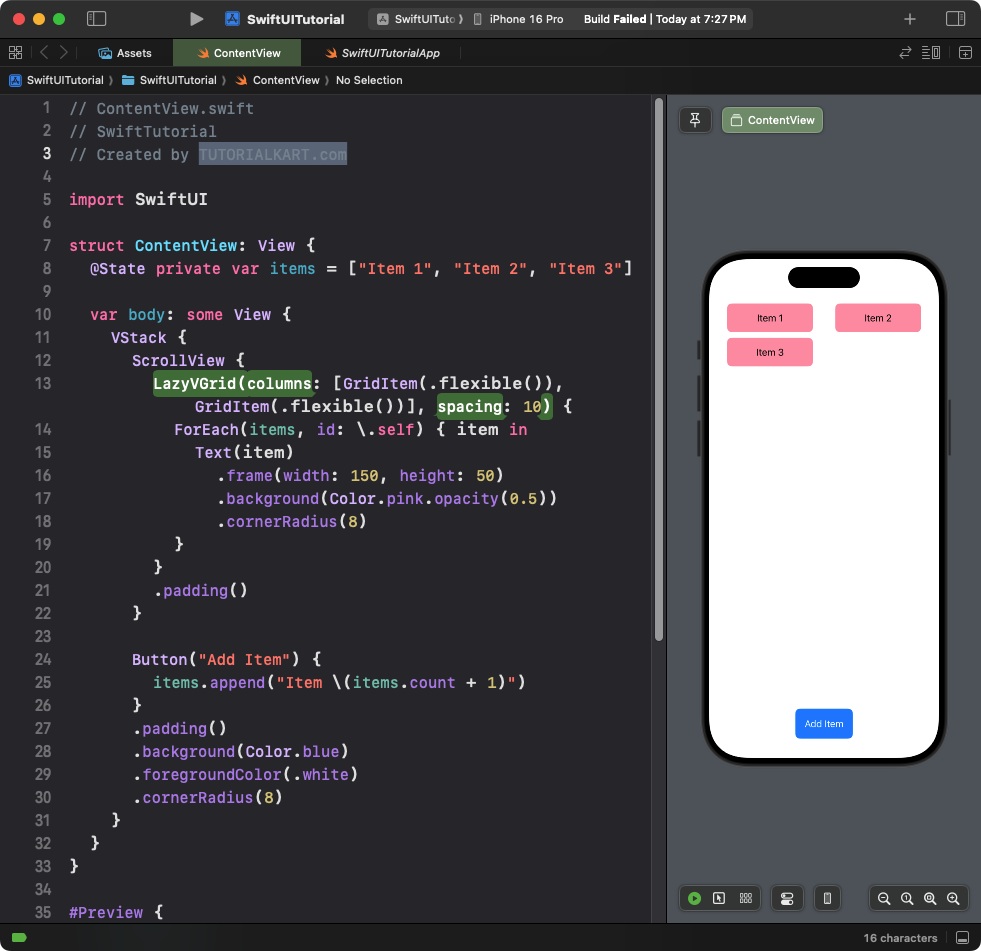
Explanation:
- The
@State
variableitems
holds the dynamic list of grid items. - A button appends a new item to the grid dynamically.
Result: A grid that dynamically updates when new items are added.
Conclusion
The LazyVGrid
in SwiftUI provides an efficient and flexible way to create vertical grids with dynamic content. By customizing column layouts and combining it with other SwiftUI components, you can create responsive and visually appealing grid-based designs for your app.