SwiftUI – MapView
In SwiftUI, the Map
view allows you to display and interact with maps in your app. With iOS 17, the Map
view uses the MapCameraPosition
to define the camera’s behavior, such as centering on the user’s location or a specific region. Additionally, the MapCameraPosition.userLocation(fallback:)
method provides a way to set a fallback position if the user’s location is unavailable.
In this SwiftUI tutorial, we will learn how to create a map with user location tracking and a fallback to a predefined region, along with examples.
Basic Syntax for Map with Fallback
The updated syntax for creating a Map
view with a fallback position is:
Map(position: $cameraPosition, interactionModes: .all)
Here:
position
: A binding to aMapCameraPosition
that controls the camera’s position and behavior, such as following the user’s location or focusing on a specific region.interactionModes
: Defines how users can interact with the map, such as zooming, panning, and rotating (.all
,.pan
, or.zoom
).
Using MapCameraPosition.userLocation(fallback:)
, you can specify a fallback region in case the user’s location is unavailable.
Examples
Let’s explore how to create a functional map with a fallback region and additional features.
Example 1: Map with User Location and Fallback
This example demonstrates how to create a map centered on the user’s location with a fallback region in case the location is unavailable:
Code Example:
import SwiftUI
import MapKit
struct ContentView: View {
@State private var cameraPosition: MapCameraPosition = .userLocation(fallback: .region(
MKCoordinateRegion(
center: CLLocationCoordinate2D(latitude: 37.7749, longitude: -122.4194), // San Francisco
span: MKCoordinateSpan(latitudeDelta: 0.05, longitudeDelta: 0.05)
)
))
var body: some View {
Map(position: $cameraPosition, interactionModes: .all)
.edgesIgnoringSafeArea(.all)
}
}
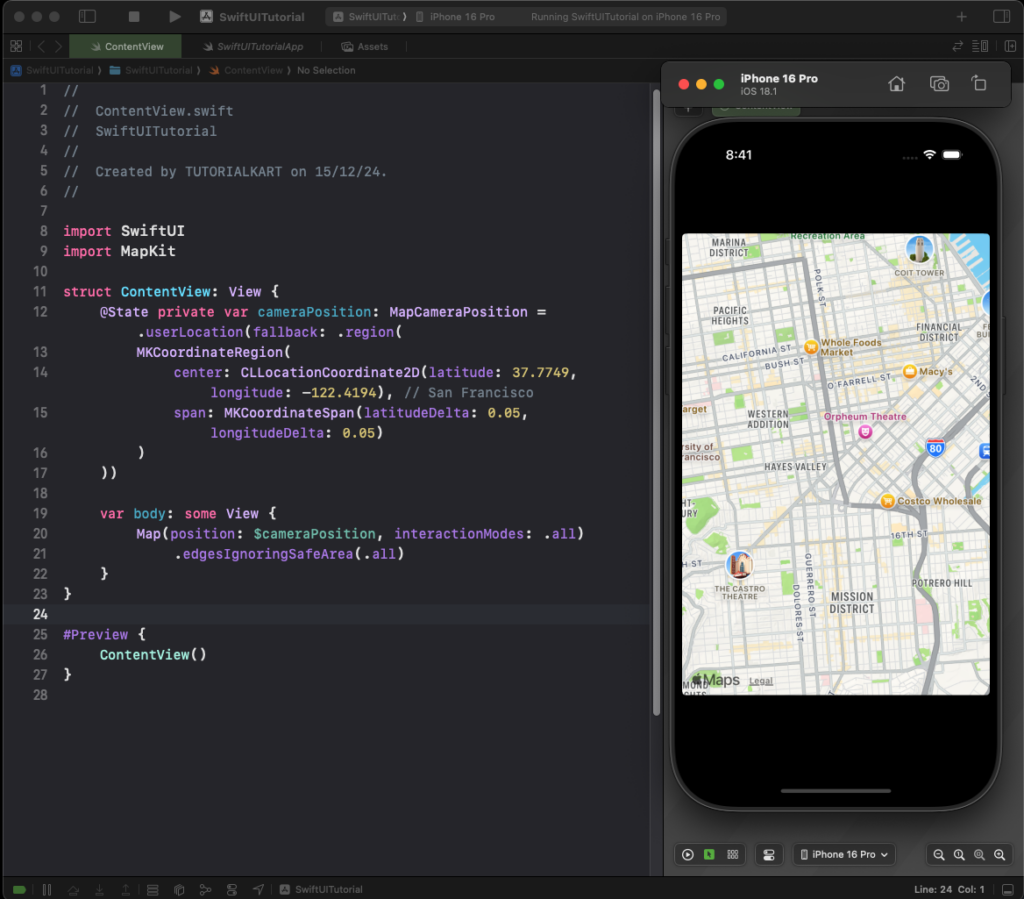
Explanation:
cameraPosition
is initialized with.userLocation(fallback:)
. If the user’s location is unavailable, the map centers on the fallback region (San Francisco in this example).- The
interactionModes: .all
parameter allows users to pan, zoom, and rotate the map. .edgesIgnoringSafeArea(.all)
makes the map fill the entire screen.
Result: The map centers on the user’s location if available, or defaults to San Francisco as the fallback region.
Conclusion
The Map
view in SwiftUI, combined with MapCameraPosition
and a fallback region, offers a flexible and user-friendly way to build location-based features. By customizing camera positions, adding interaction modes, and displaying annotations, you can create interactive and dynamic maps for your apps.