SwiftUI Round Image
In SwiftUI, creating round images enhances visual appeal and provides a clean, modern design. Using the .clipShape(Circle())
modifier, you can easily transform images into circular shapes. In this tutorial, we will demonstrate how to create round images with examples for both square and landscape images.
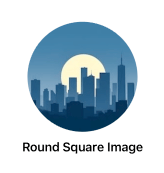
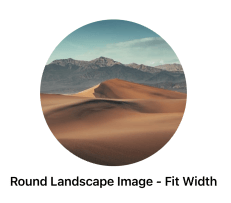
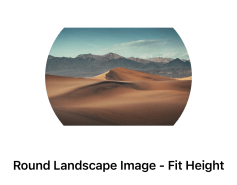
When to Use Round Images
Round images are useful in scenarios like:
- Displaying profile pictures or avatars.
- Creating modern UI elements for buttons or thumbnails.
- Highlighting key images in a design.
Syntax to Create Round Images
Here’s the basic syntax for creating a round image:
Image("imageName")
.resizable()
.scaledToFit()
.clipShape(Circle())
Examples to Create Round Images
In the following examples, we demonstrate creating round images for both square and landscape images.
Example 1: Round Image for a Square Image
In this example, we transform a square image into a circular shape using the .clipShape(Circle())
modifier.
File: ContentView.swift
// ContentView.swift
// Created by TUTORIALKART.com
import SwiftUI
struct ContentView: View {
var body: some View {
VStack {
RoundSquareImageView()
}
}
}
struct RoundSquareImageView: View {
var body: some View {
VStack {
Image("squareImage")
.resizable()
.scaledToFit()
.frame(width: 150, height: 150)
.clipShape(Circle())
Text("Round Square Image")
.font(.headline)
}
.padding()
}
}
#Preview {
ContentView()
}
Detailed Explanation
- Image: The
Image
view loads a square image namedsquareImage
from the app’s assets. - .resizable: Ensures the image can scale dynamically.
- .clipShape(Circle()): Clips the image to a circular shape.
- .frame: Restricts the image dimensions to 150×150 points.
Output
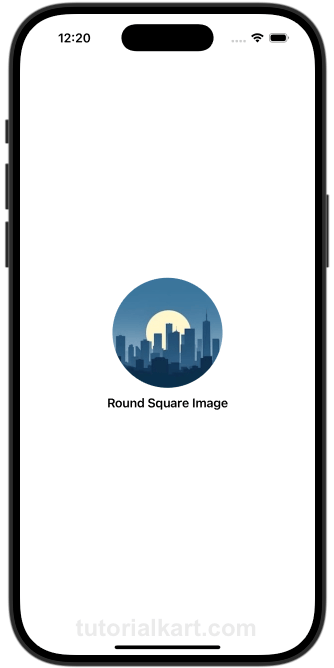
Example 2: Round Image for a Landscape Image (Fit to Width)
In this example, we create a round image from a landscape image, ensuring it fits within a circular frame while maintaining its width.
File: ContentView.swift
// ContentView.swift
// Created by TUTORIALKART.com
import SwiftUI
struct ContentView: View {
var body: some View {
VStack {
RoundLandscapeImageFitWidthView()
}
}
}
struct RoundLandscapeImageFitWidthView: View {
var body: some View {
VStack {
Image("sampleImage")
.resizable()
.scaledToFill()
.frame(width: 200, height: 200)
.clipShape(Circle())
Text("Round Landscape Image - Fit Width")
.font(.headline)
}
.padding()
}
}
#Preview {
ContentView()
}
Detailed Explanation
- Image: Loads a landscape image named
customImage
from the app’s assets. - .scaledToFill: Ensures the image fills the circular frame while maintaining its width.
- .clipShape(Circle()): Clips the image into a perfect circle.
- .frame: Sets the dimensions of the circular frame to 200×200 points.
Output
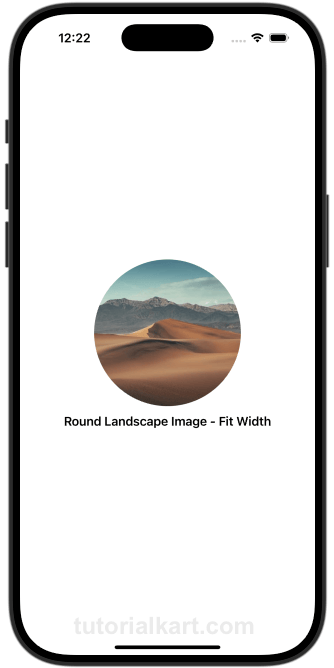
Example 3: Round Image for a Landscape Image (Fit to Height)
In this example, we create a round image from a landscape image, ensuring it fits within a circular frame while maintaining its height.
File: ContentView.swift
// ContentView.swift
// Created by TUTORIALKART.com
import SwiftUI
struct ContentView: View {
var body: some View {
VStack {
RoundLandscapeImageFitHeightView()
}
}
}
struct RoundLandscapeImageFitHeightView: View {
var body: some View {
VStack {
Image("sampleImage")
.resizable()
.scaledToFit()
.frame(width: 200, height: 200)
.clipShape(Circle())
Text("Round Landscape Image - Fit Height")
.font(.headline)
}
.padding()
}
}
#Preview {
ContentView()
}
Detailed Explanation
- Image: Loads a landscape image named
customImage
from the app’s assets. - .scaledToFit: Ensures the image fits within the circular frame without being cropped, maintaining its height.
- .clipShape(Circle()): Clips the image into a circular shape.
- .frame: Defines the circular frame dimensions as 200×200 points.
Output
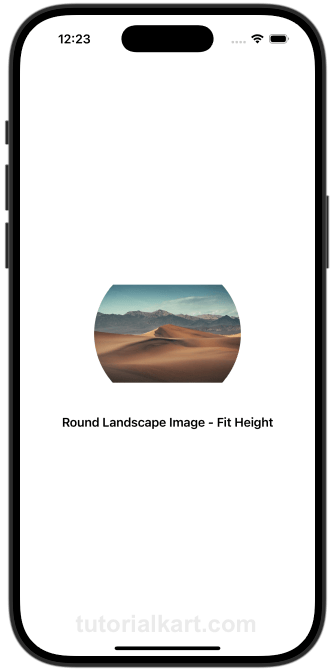
Conclusion
Creating round images in SwiftUI enhances visual appeal and is suitable for various use cases like avatars or thumbnails. By combining .clipShape(Circle())
with appropriate scaling modifiers, you can handle both square and landscape images effectively.