SwiftUI SecureField Focus
Managing focus in SwiftUI is essential when dealing with forms, especially when collecting sensitive information like passwords. With the SecureField
, you can programmatically control focus to improve user experience.
This tutorial will guide you through implementing focus management in a SecureField
, allowing you to move focus dynamically based on user actions.
When to Use Focus for SecureField
Focus management is particularly useful in the following scenarios:
- Improving the flow of form navigation.
- Highlighting the current input field for better usability.
- Triggering actions when the user moves away from a field.
Syntax
The FocusState
property wrapper in SwiftUI allows you to manage focus programmatically for input fields. Below is the syntax for using SecureField
with focus management:
@FocusState private var isFocused: Bool
SecureField("Enter your password", text: $password)
.focused($isFocused)
Example
This example demonstrates how to use SecureField
with FocusState
to manage focus dynamically. Users can toggle focus or move to the next field with programmatic control.
File: ContentView.swift
import SwiftUI
struct ContentView: View {
@State private var password: String = ""
@FocusState private var isPasswordFieldFocused: Bool
var body: some View {
VStack(spacing: 20) {
SecureField("Enter your password", text: $password)
.padding()
.textFieldStyle(RoundedBorderTextFieldStyle())
.focused($isPasswordFieldFocused)
Button("Toggle Focus") {
isPasswordFieldFocused.toggle()
}
.padding()
.buttonStyle(.borderedProminent)
Spacer()
}
.padding()
}
}
struct ContentView_Previews: PreviewProvider {
static var previews: some View {
ContentView()
}
}
Detailed Explanation
- SecureField: Captures the user’s password securely and binds it to the
@State
variablepassword
. It uses the.focused
modifier to control focus. - FocusState: The
@FocusState
property wrapper enables programmatic focus control. TheisPasswordFieldFocused
variable determines whether theSecureField
is focused. - Button: The “Toggle Focus” button demonstrates how to toggle the focus state dynamically.
- Layout: The
VStack
organizes the components vertically with padding and spacing for a clean layout.
Output
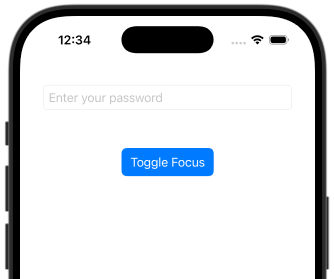
Working Video
Conclusion
Focus management in SwiftUI with SecureField
and FocusState
enhances user experience by allowing dynamic control of input fields.