SwiftUI SecureField onChange
In SwiftUI, the SecureField is a specialized input field designed for entering secure or sensitive information, such as passwords. The onChange
modifier allows you to react to changes in the value of the SecureField
. This makes it particularly useful for validating input or updating the UI dynamically based on user input.
In this tutorial, you will learn how to use SecureField onChange modifier, with a well formed example, and detailed explanation for the example.
When is SecureField onChange Used?
The onChange
modifier is typically used in scenarios where:
- You need to validate the password as the user types.
- Real-time feedback is required, such as updating a password strength indicator.
- You want to trigger some logic based on changes in the field, like enabling or disabling a button.
Example
In this example, we will use SecureField onChange modifier to display password strength in real time when user enters the value in SecureField.
File: ContentView.swift
import SwiftUI
struct ContentView: View {
@State private var password: String = ""
@State private var passwordStrength: String = "Weak"
var body: some View {
VStack(spacing: 20) {
SecureField("Enter your password", text: $password)
.padding()
.textFieldStyle(RoundedBorderTextFieldStyle())
.onChange(of: password) { newValue in
updatePasswordStrength(for: newValue)
}
Text("Password Strength: \(passwordStrength)")
.foregroundColor(passwordStrengthColor())
.font(.headline)
Spacer()
}
.padding()
}
private func updatePasswordStrength(for password: String) {
if password.count < 6 {
passwordStrength = "Weak"
} else if password.count < 12 {
passwordStrength = "Moderate"
} else {
passwordStrength = "Strong"
}
}
private func passwordStrengthColor() -> Color {
switch passwordStrength {
case "Weak":
return .red
case "Moderate":
return .orange
case "Strong":
return .green
default:
return .gray
}
}
}
struct ContentView_Previews: PreviewProvider {
static var previews: some View {
ContentView()
}
}
Detailed Explanation for the Example
- Importing SwiftUI: The code begins by importing the SwiftUI framework, which provides all the necessary tools for building the UI.
- State Variables: The
@State
propertiespassword
andpasswordStrength
store the user’s password and its strength, respectively. These properties enable the UI to react to changes in real-time. - SecureField: The
SecureField
is bound to thepassword
variable using the$password
binding. It has a placeholder text “Enter your password” and uses the.onChange
modifier to monitor changes to thepassword
. - Password Strength Logic: The
updatePasswordStrength
function evaluates the password’s length and assigns a strength value (Weak, Moderate, or Strong) accordingly. This function is called whenever the password changes. - Password Strength Indicator: A
Text
view displays the password strength. The text color dynamically updates based on the strength using thepasswordStrengthColor
function. - Layout: The
VStack
organizes the components vertically with padding and spacing for a clean layout. - Preview: The
ContentView_Previews
struct allows you to preview the UI in Xcode’s canvas during development.
Output
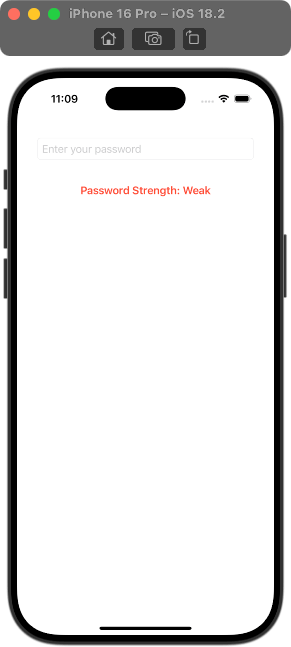
Conclusion
The SecureField
with onChange
in SwiftUI is a powerful combination for creating responsive and user-friendly forms. This example demonstrates how to provide real-time feedback to users, improving the overall experience.