SwiftUI – Text Character Spacing
In SwiftUI, you can adjust the spacing between characters in a Text
view using the kerning(_:)
and tracking(_:)
modifiers. These modifiers allow you to create visually appealing typography by customizing the distance between letters in your text.
While both kerning(_:)
and tracking(_:)
adjust character spacing, they differ slightly:
- kerning(_:): Adds spacing between individual characters.
- tracking(_:): Applies uniform spacing adjustment across the entire text, useful for consistent adjustments.
Syntax for Character Spacing
Here’s the basic syntax for adjusting character spacing:
Text("Your text here")
.kerning(value) // Adjust spacing between characters
.tracking(value) // Apply uniform spacing across the text
Here:
"Your text here"
: The content of theText
view.value
: The spacing value in points. Positive values increase spacing, while negative values decrease it.
Choose kerning(_:)
for fine-tuned adjustments between characters and tracking(_:)
for consistent adjustments across all characters.
Examples
Let’s explore how to use character spacing in SwiftUI with a few examples.
Example 1: Adjusting Character Spacing with Kerning
Here’s how you can increase the spacing between individual characters:
Code Example:
import SwiftUI
struct ContentView: View {
var body: some View {
Text("SwiftUI Kerning Example")
.kerning(5) // Increase spacing by 5 points
.padding()
.font(.title)
.background(Color.blue.opacity(0.2))
}
}
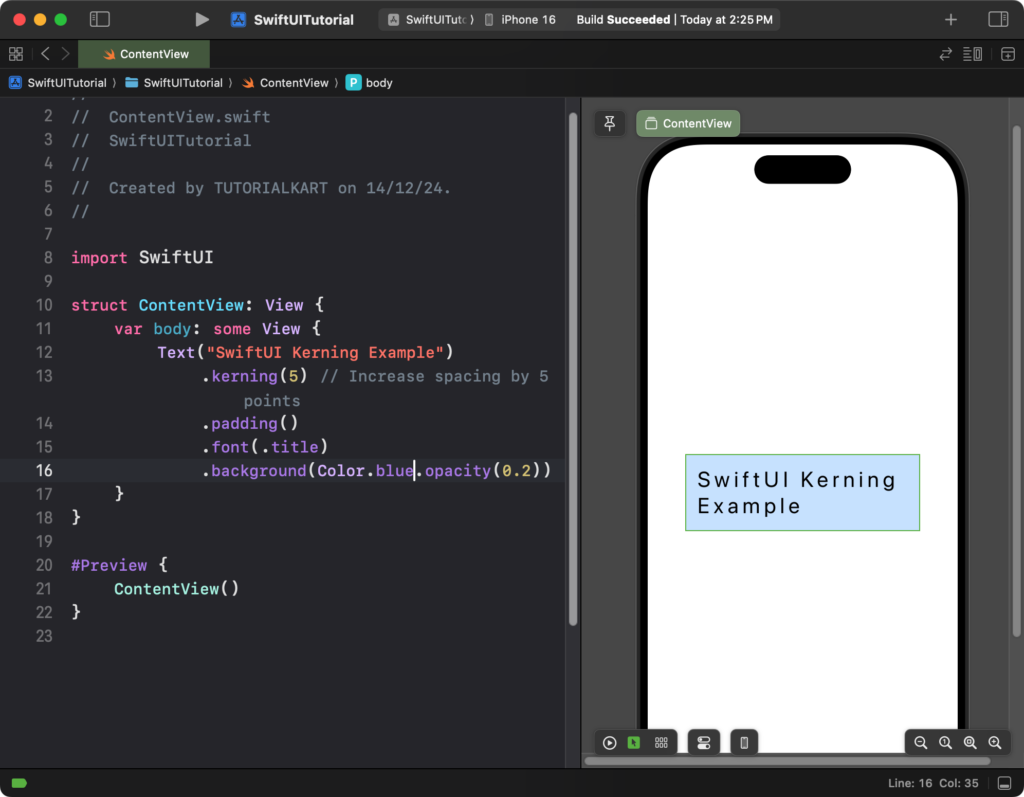
Explanation:
- The
.kerning(5)
modifier increases the spacing between individual characters by 5 points. - The
.font(.title)
makes the text larger, making the increased spacing more noticeable. - The
.background
adds a light blue background for visual emphasis.
Result: The characters in the text will have increased spacing, creating a more open appearance.
Example 2: Adjusting Character Spacing with Tracking
Here’s how you can apply consistent spacing across the entire text using tracking(_:)
:
Code Example:
import SwiftUI
struct ContentView: View {
var body: some View {
Text("SwiftUI Tracking Example")
.tracking(8) // Apply uniform spacing of 8 points
.padding()
.font(.headline)
.foregroundColor(.purple)
}
}
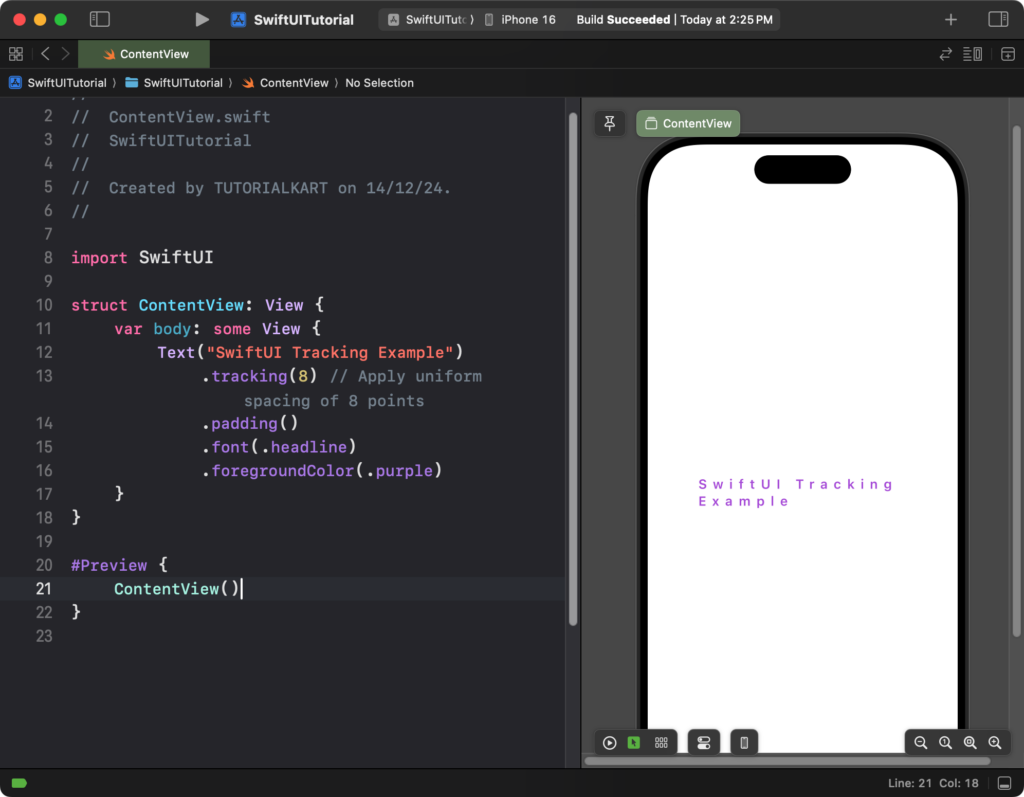
Explanation:
- The
.tracking(8)
modifier applies consistent spacing of 8 points across all characters. - The
.font(.headline)
adjusts the font size to highlight the spacing effect. - The
.foregroundColor(.purple)
sets the text color to purple for better visibility.
Result: The text will have uniform character spacing, making it appear more spaced out overall.
Example 3: Reducing Character Spacing
You can also reduce the spacing between characters by using negative values:
Code Example:
import SwiftUI
struct ContentView: View {
var body: some View {
Text("Reduced Spacing Example")
.kerning(-2) // Reduce spacing by 2 points
.padding()
.font(.largeTitle)
.foregroundColor(.red)
}
}
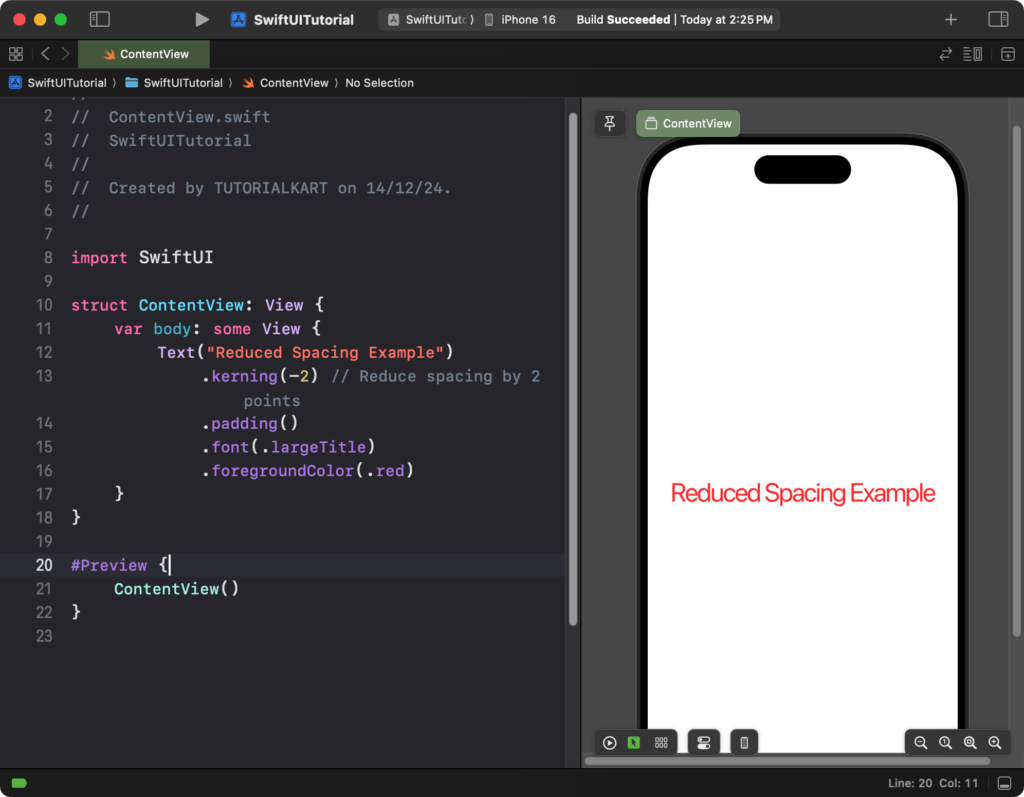
Explanation:
- The
.kerning(-2)
modifier reduces the spacing between characters by 2 points. - The
.font(.largeTitle)
makes the text larger for clarity. - The
.foregroundColor(.red)
changes the text color to red for emphasis.
Result: The characters in the text will appear closer together, creating a compact look.
Conclusion
In SwiftUI, the kerning(_:)
and tracking(_:)
modifiers allow you to fine-tune text character spacing to achieve the desired typography effects. Whether you want to create an open, spaced-out look or a compact, tight design, these modifiers provide the flexibility you need for your app’s text styling.