SwiftUI – Text Color
In SwiftUI, you can customize the text color of a Text
view using the .foregroundColor(_:)
modifier. This allows you to set the color of the text using predefined system colors or custom colors.
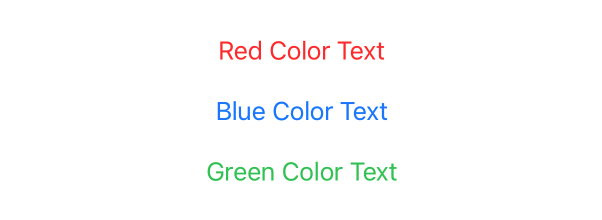
In this tutorial, we will look at the basic syntax and some examples to understand how to set text colors in SwiftUI.
Syntax for Setting Text Color
The syntax for changing the text color in SwiftUI is:
Text("Your Text Here")
.foregroundColor(Color.colorName)
Here:
"Your Text Here"
: The text content to display..foregroundColor
: A modifier to set the text color.Color.colorName
: A predefined or custom color. Examples of predefined colors includeColor.red
,Color.blue
,Color.green
, etc.
You can also use custom colors defined in your asset catalog or created using RGB/HEX values.
Examples
In the following examples, we will set the color for Text Views, using predefined colors, colors from asset catalog, or custom colors.
Example 1: Using Predefined Colors
Below is an example of how to use predefined system colors to change the text color:
Code Example:
import SwiftUI
struct ContentView: View {
var body: some View {
VStack(spacing: 20) {
Text("Red Color Text")
.foregroundColor(Color.red)
Text("Blue Color Text")
.foregroundColor(Color.blue)
Text("Green Color Text")
.foregroundColor(Color.green)
}
.padding()
}
}
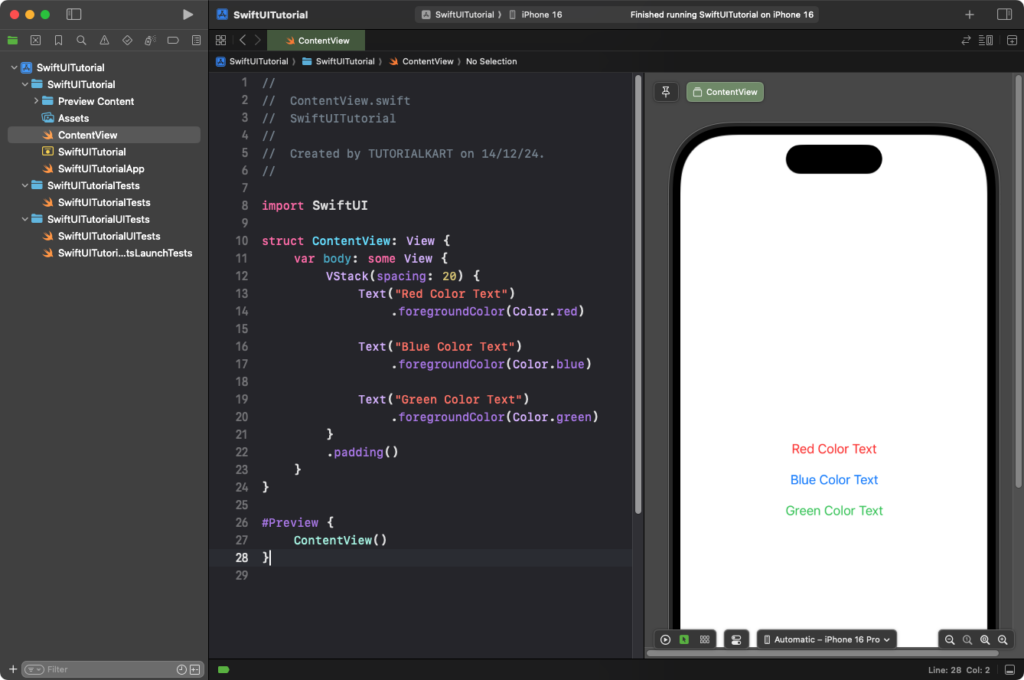
Explanation:
- The
Text("Hello, World!")
uses.foregroundColor(Color.red)
, making the text appear red. - The
Text("SwiftUI is amazing!")
is styled with.foregroundColor(Color.blue)
, making the text blue. - The
Text("Let's build great apps!")
is styled with.foregroundColor(Color.green)
, making the text green.
The VStack
stacks these text elements vertically, and spacing
adds space between them.
Example 2: Using Custom Colors
You can define custom colors in your asset catalog or use RGB/HEX values to set text colors. Here’s how:
Code Example:
import SwiftUI
struct ContentView: View {
var body: some View {
VStack(spacing: 20) {
Text("Custom Color Example")
.foregroundColor(Color("CustomColor")) // From asset catalog
Text("RGB Color Example")
.foregroundColor(Color(red: 0.5, green: 0.8, blue: 0.3)) // Custom RGB
Text("HEX Color Example")
.foregroundColor(Color(hex: "#FF5733")) // Custom HEX (requires extension)
}
.padding()
}
}
// Extension to support HEX colors
extension Color {
init(hex: String) {
let scanner = Scanner(string: hex)
scanner.scanLocation = hex.hasPrefix("#") ? 1 : 0
var rgbValue: UInt64 = 0
scanner.scanHexInt64(&rgbValue)
let red = Double((rgbValue & 0xFF0000) >> 16) / 255.0
let green = Double((rgbValue & 0x00FF00) >> 8) / 255.0
let blue = Double(rgbValue & 0x0000FF) / 255.0
self.init(red: red, green: green, blue: blue)
}
}
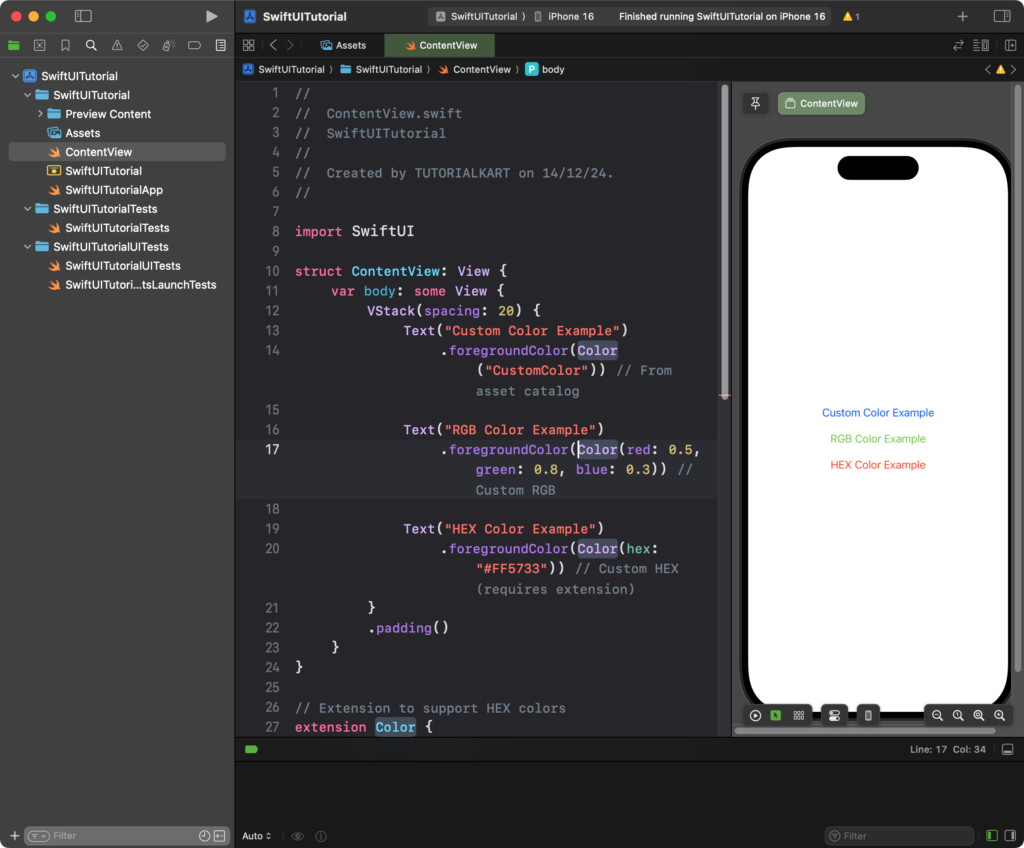
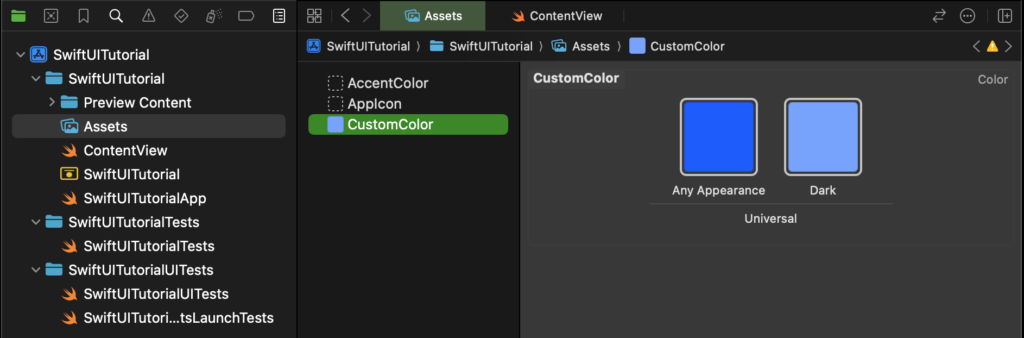
Explanation:
Color("CustomColor")
retrieves a custom color from the asset catalog.Color(red: 0.5, green: 0.8, blue: 0.3)
defines a custom RGB color.Color(hex: "#FF5733")
uses a HEX value for defining a custom color, which requires an extension for HEX support.
This flexibility allows you to maintain consistent branding and aesthetics in your app.
Conclusion
SwiftUI’s .foregroundColor
modifier is used to customise text colors using predefined system colors, asset catalog colors, or custom RGB/HEX values.