SwiftUI – Text Size
In SwiftUI, the Text
view allows you to display and style textual content. One of the most common customisations is adjusting the text size to match the design requirements. You can easily set the size of text using predefined font sizes, custom font styles, or scaling mechanisms for accessibility.
In this SwiftUI tutorial, we cover different ways to customise the size of text in SwiftUI, including examples for predefined styles, custom font sizes, and dynamic type scaling.
Setting Text Size in SwiftUI
The most common ways to set text size in SwiftUI include:
- Using predefined font styles: SwiftUI provides system-defined font styles like
.largeTitle
,.title
,.body
, etc. - Using custom font sizes: You can specify an exact font size in points using the
.font()
modifier withFont.system(size:)
. - Dynamic type scaling: Ensures your text scales appropriately based on the user’s accessibility settings.
Examples
Let’s explore how to adjust text size in SwiftUI with practical examples.
Example 1: Using Predefined Font Styles
SwiftUI provides a variety of predefined font styles that you can use to set the size of your text:
Code Example:
import SwiftUI
struct ContentView: View {
var body: some View {
VStack(alignment: .leading, spacing: 10) {
Text("Large Title").font(.largeTitle)
Text("Title").font(.title)
Text("Headline").font(.headline)
Text("Body").font(.body)
Text("Caption").font(.caption)
}
.padding()
}
}
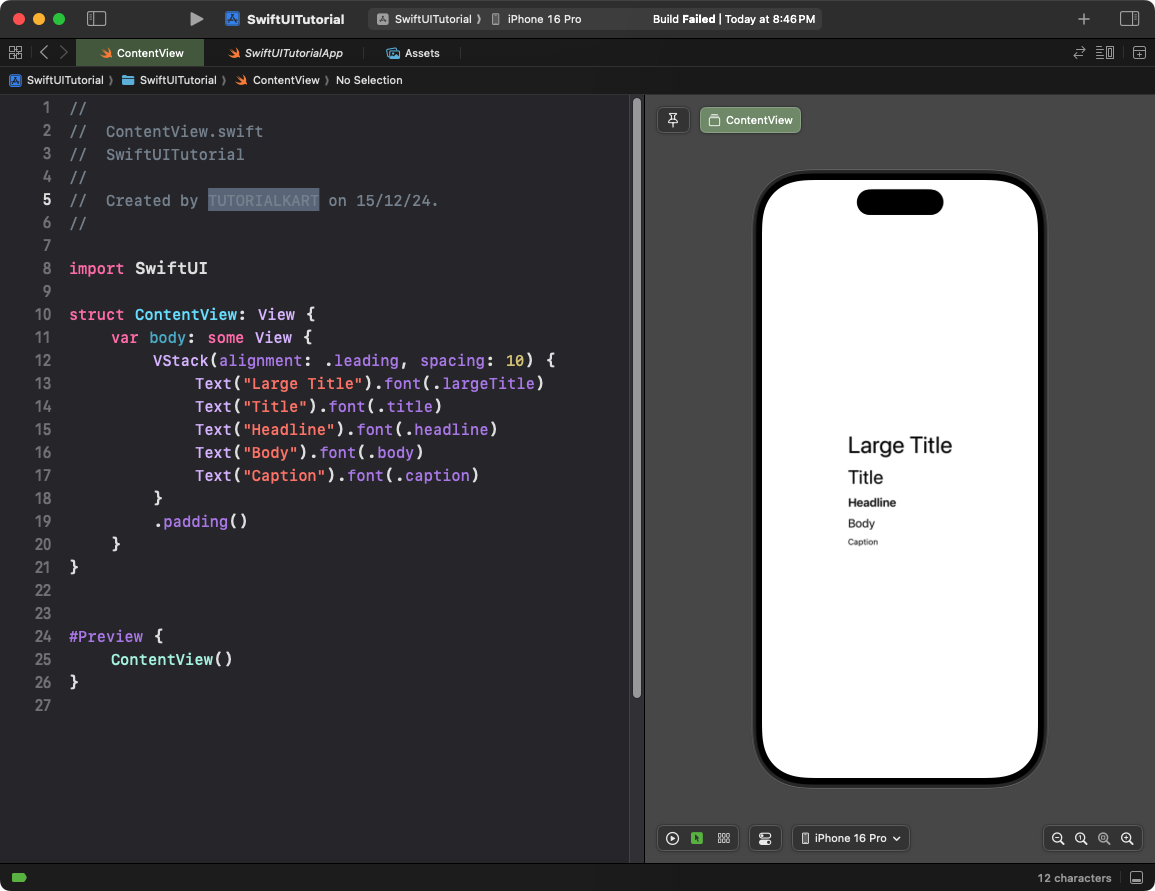
Explanation:
.font(.largeTitle)
: Sets the text to the largest predefined size..font(.title)
: Slightly smaller than.largeTitle
..font(.body)
: The default text size used for regular content..font(.caption)
: Small text, typically for supplementary information.
Result: Text with different predefined sizes is displayed in a vertical stack.
Example 2: Using Custom Font Sizes
You can specify an exact font size using Font.system(size:)
:
Code Example:
import SwiftUI
struct ContentView: View {
var body: some View {
VStack(alignment: .leading, spacing: 10) {
Text("Custom Font Size: 24").font(.system(size: 24))
Text("Custom Font Size: 18").font(.system(size: 18))
Text("Custom Font Size: 12").font(.system(size: 12))
}
.padding()
}
}
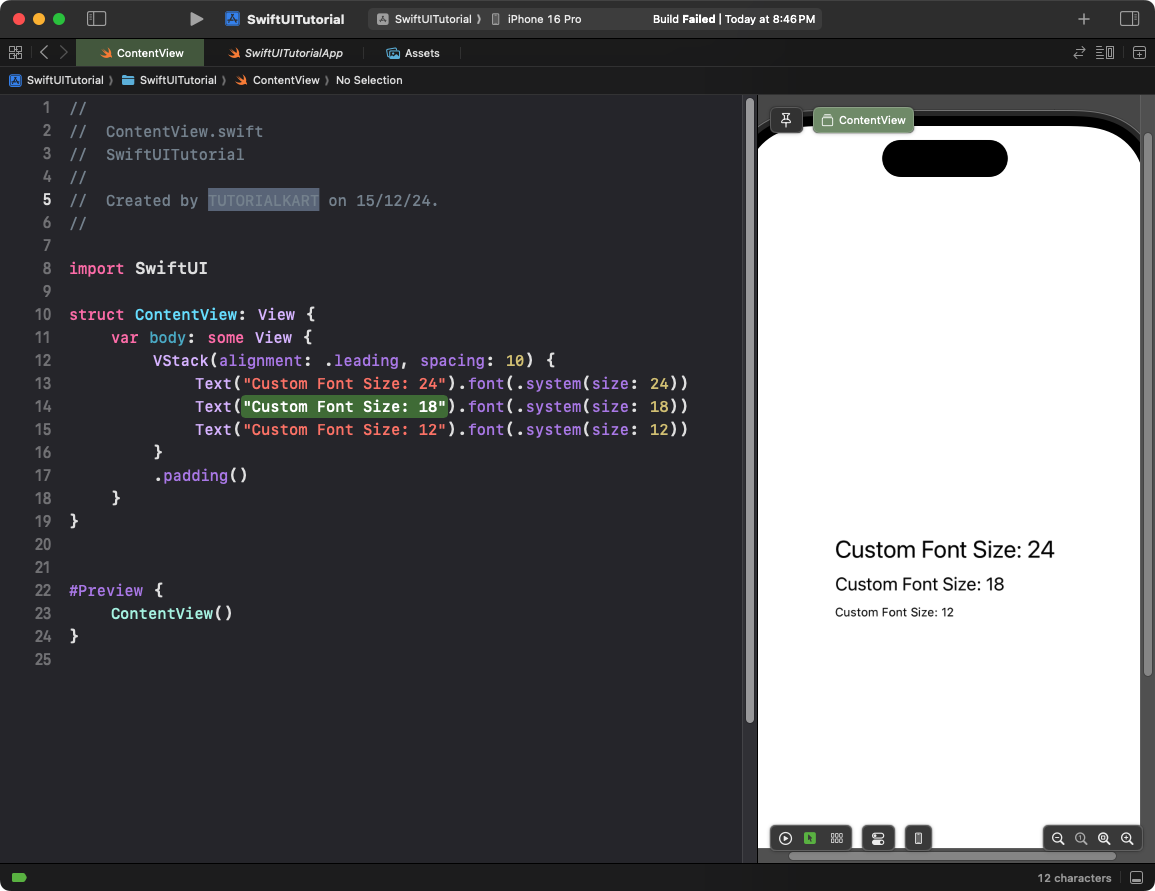
Explanation:
- The
.font(.system(size:))
modifier allows you to set the exact font size in points. - Each
Text
view has a different font size to demonstrate the effect.
Result: Text with custom font sizes is displayed in a vertical stack.
Example 3: Dynamic Type Scaling
Dynamic type ensures your text adjusts to the user’s accessibility settings. Use .dynamicTypeSize
to enable this behavior:
Code Example:
import SwiftUI
struct ContentView: View {
var body: some View {
Text("This text supports dynamic type scaling.")
.font(.body)
.dynamicTypeSize(.xSmall ... .accessibility3) // Supports a range of sizes
.padding()
}
}
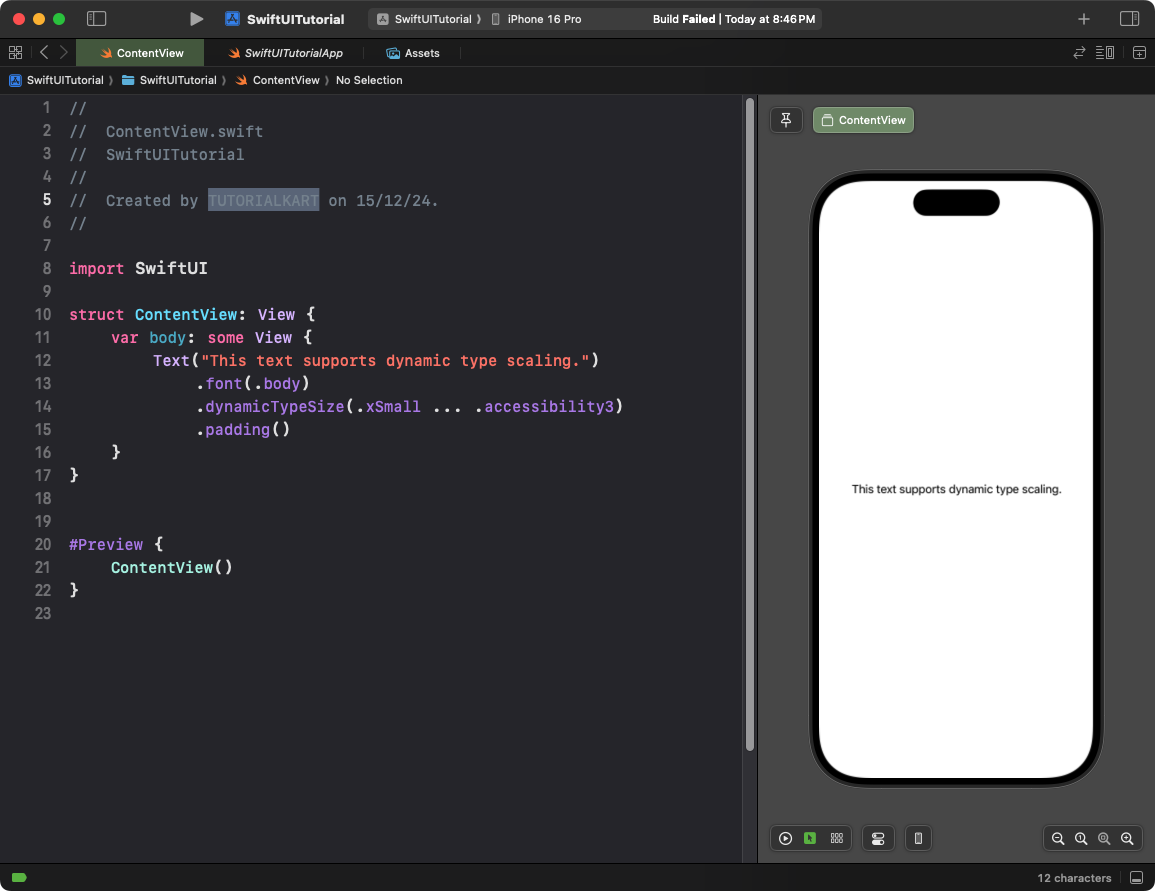
Explanation:
.dynamicTypeSize(.xSmall ... .accessibility3)
: Specifies the range of sizes supported for dynamic type.- The text automatically scales to fit the user’s accessibility settings.
Result: The text scales dynamically based on the user’s preferred font size settings in the device’s accessibility menu.
Conclusion
SwiftUI provides several ways to customize text size, from predefined font styles to custom sizes and dynamic type scaling for accessibility. By using these tools, you can create visually appealing and user-friendly interfaces tailored to your app’s design requirements.