SwiftUI – TextField Border Color
In SwiftUI, you can style a TextField to match your app’s design requirements, including customizing its border color.
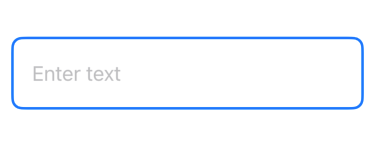
While SwiftUI does not directly provide a modifier for changing the border color, you can achieve this using the .overlay()
modifier or by wrapping the TextField
in a container with a custom border.
In this tutorial, we’ll explore how to customize the border color of a TextField
in SwiftUI with practical examples.
Customizing Border Color
To change the border color of a TextField
, you can use:
- The
.overlay()
Modifier: Add a custom border over theTextField
. - A Container View: Wrap the
TextField
in aZStack
orRoundedRectangle
with a border color.
Examples
Let’s look at examples of how to apply a custom border color to a TextField
in SwiftUI.
Example 1: Using the Overlay Modifier
This example demonstrates how to customize the border color of a TextField
using the .overlay()
modifier:
Code Example:
import SwiftUI
struct ContentView: View {
@State private var text: String = ""
var body: some View {
TextField("Enter text", text: $text)
.padding()
.overlay(
RoundedRectangle(cornerRadius: 8)
.stroke(Color.blue, lineWidth: 2) // Blue border with 2pt width
)
.padding()
}
}
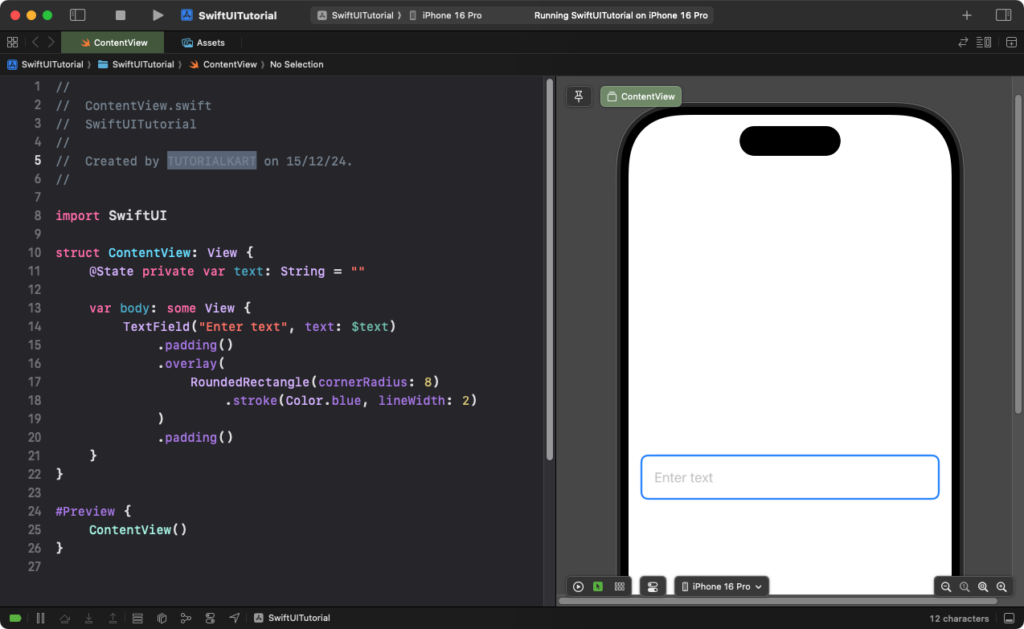
Explanation:
- The
.overlay()
modifier adds a blue border around theTextField
. - The
RoundedRectangle(cornerRadius: 8)
ensures the border has rounded corners. - The
.stroke(Color.blue, lineWidth: 2)
specifies the border color and thickness.
Result: The TextField
displays with a blue border and rounded corners.
Example 2: Dynamic Border Color
This example changes the border color dynamically based on user input:
Code Example:
import SwiftUI
struct ContentView: View {
@State private var text: String = ""
var borderColor: Color {
text.isEmpty ? .red : .green // Red for empty input, green for valid input
}
var body: some View {
TextField("Enter text", text: $text)
.padding()
.overlay(
RoundedRectangle(cornerRadius: 8)
.stroke(borderColor, lineWidth: 2)
)
.padding()
}
}
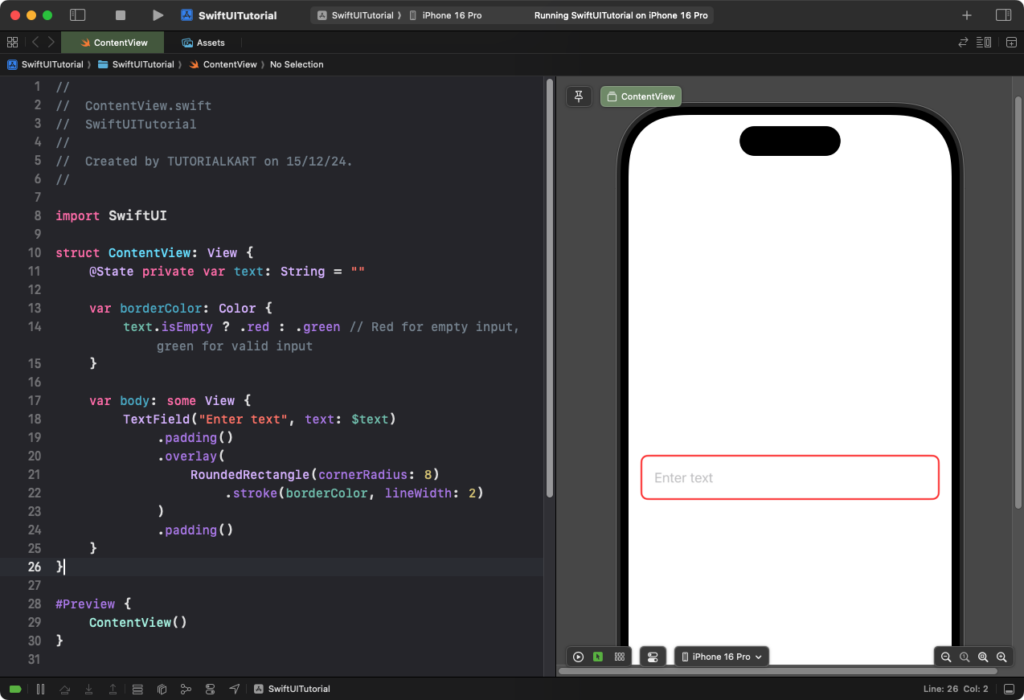
Explanation:
- The
borderColor
variable dynamically determines the border color based on the input. - If the input is empty, the border color is red; otherwise, it changes to green.
- The
.overlay()
modifier applies the dynamic border color to theTextField
.
Result: The border color changes dynamically based on the content of the TextField
.
Conclusion
By using the .overlay()
modifier or a container view, you can easily customize the border color of a TextField
in SwiftUI. Whether you want a static, dynamic, or multi-styled border, these techniques provide flexibility to match your app’s design requirements.