SwiftUI – Toggle as Checkbox
In SwiftUI, a Toggle is primarily designed to look like a switch. However, with customisation, you can make it appear and behave like a checkbox. Checkboxes are commonly used in forms or settings where users can select or deselect an option.
This tutorial demonstrates how to create a toggle that mimics the appearance and functionality of a checkbox in SwiftUI.
Creating a Toggle Checkbox
To make a toggle look like a checkbox, you can:
- Use the
Label
style to align a text description with the toggle. - Style the toggle using
.toggleStyle()
andButtonToggleStyle
to achieve a checkbox-like appearance. - Customize the toggle with images to enhance the visual feedback.
Examples
Here are examples of how to create a toggle that functions and looks like a checkbox.
Example 1: Simple Checkbox with Text
This example creates a basic checkbox-style toggle with a text label:
Code Example:
import SwiftUI
struct ContentView: View {
@State private var isChecked: Bool = false
var body: some View {
Toggle(isOn: $isChecked) {
Text("I agree to the terms and conditions")
.font(.body)
}
.toggleStyle(CheckboxToggleStyle()) // Custom checkbox style
.padding()
}
}
struct CheckboxToggleStyle: ToggleStyle {
func makeBody(configuration: Configuration) -> some View {
HStack {
Image(systemName: configuration.isOn ? "checkmark.square.fill" : "square")
.foregroundColor(configuration.isOn ? .blue : .gray)
.onTapGesture { configuration.isOn.toggle() } // Toggle the state
configuration.label
}
}
}
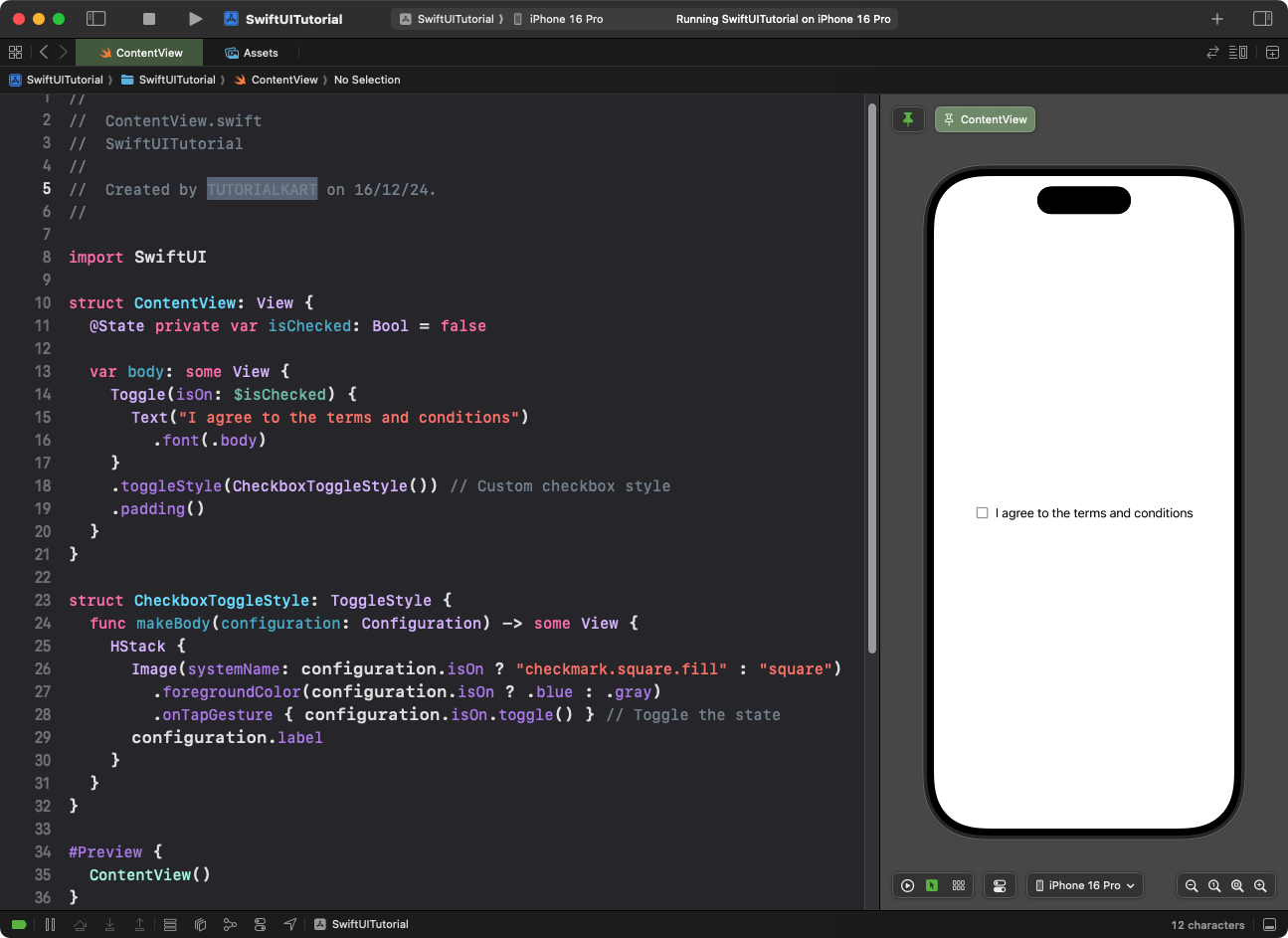
Explanation:
- The
CheckboxToggleStyle
customises the toggle to use a checkbox icon (square
for unchecked andcheckmark.square.fill
for checked). - The
onTapGesture
toggles the state when the checkbox is tapped. - The text label is displayed next to the checkbox.
Result: A checkbox-style toggle with a text label appears, allowing users to check or uncheck the box.
Example 2: Toggle as Checkbox with Dynamic State
This example uses a checkbox to enable or disable a setting:
Code Example:
import SwiftUI
struct ContentView: View {
@State private var isEmailEnabled: Bool = false
var body: some View {
VStack(alignment: .leading, spacing: 20) {
Toggle(isOn: $isEmailEnabled) {
Text("Enable Email Notifications")
}
.toggleStyle(CheckboxToggleStyle())
Text("Email notifications are \(isEmailEnabled ? "enabled" : "disabled").")
.foregroundColor(isEmailEnabled ? .green : .red)
}
.padding()
}
}
struct CheckboxToggleStyle: ToggleStyle {
func makeBody(configuration: Configuration) -> some View {
HStack {
Image(systemName: configuration.isOn ? "checkmark.square.fill" : "square")
.foregroundColor(configuration.isOn ? .blue : .gray)
.onTapGesture { configuration.isOn.toggle() }
configuration.label
}
}
}
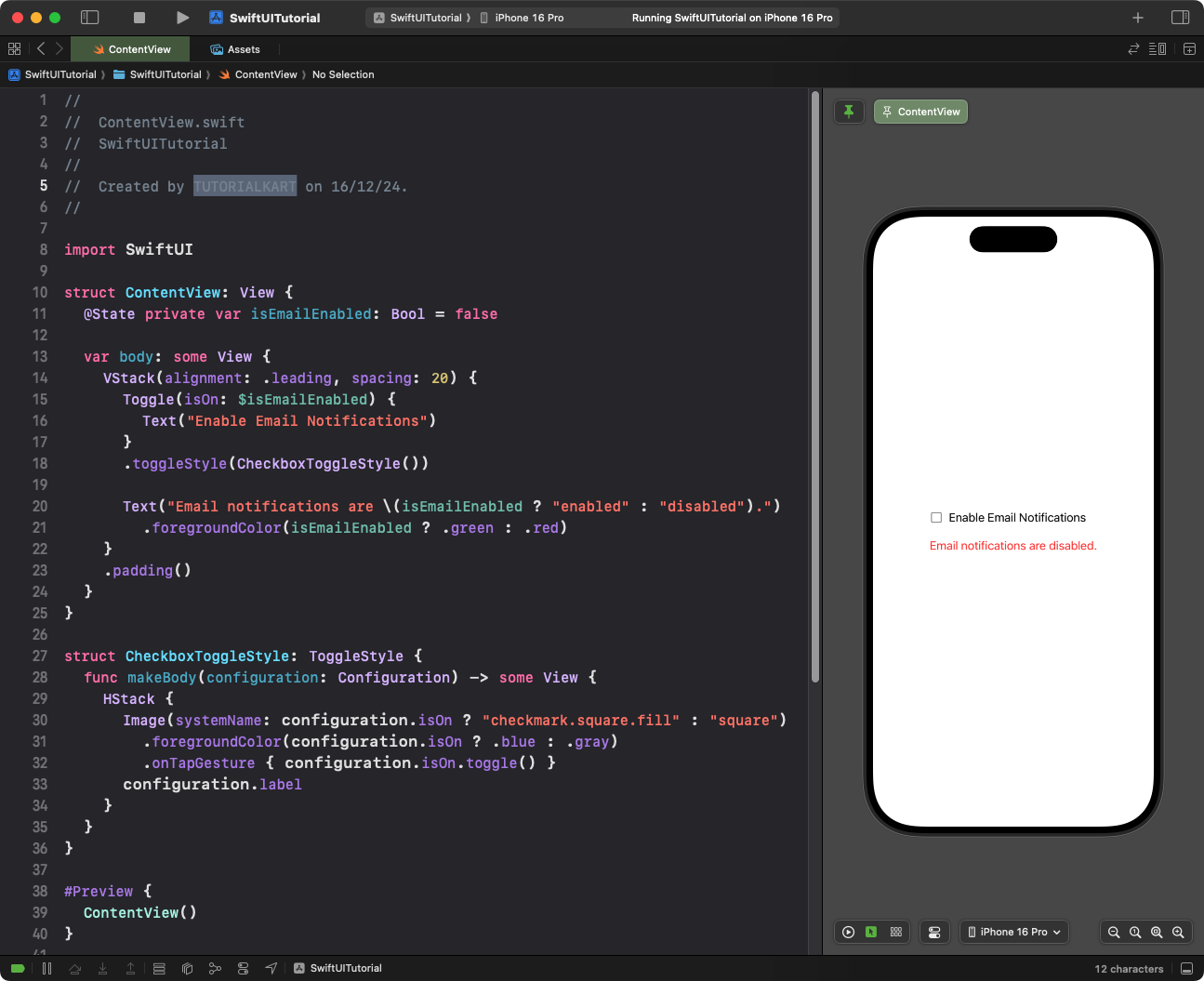
Explanation:
- The checkbox toggles the
isEmailEnabled
state. - The label dynamically reflects the toggle’s state, displaying “enabled” in green or “disabled” in red.
Result: A checkbox-style toggle controls email notifications and displays the current state to the user.
Example 3: Checkbox with Multiple Options
This example demonstrates a group of checkboxes for multiple options:
Code Example:
import SwiftUI
struct ContentView: View {
@State private var isOption1Selected: Bool = false
@State private var isOption2Selected: Bool = false
var body: some View {
VStack(alignment: .leading, spacing: 15) {
Toggle(isOn: $isOption1Selected) {
Text("Option 1")
}
.toggleStyle(CheckboxToggleStyle())
Toggle(isOn: $isOption2Selected) {
Text("Option 2")
}
.toggleStyle(CheckboxToggleStyle())
}
.padding()
}
}
struct CheckboxToggleStyle: ToggleStyle {
func makeBody(configuration: Configuration) -> some View {
HStack {
Image(systemName: configuration.isOn ? "checkmark.square.fill" : "square")
.foregroundColor(configuration.isOn ? .blue : .gray)
.onTapGesture { configuration.isOn.toggle() }
configuration.label
}
}
}
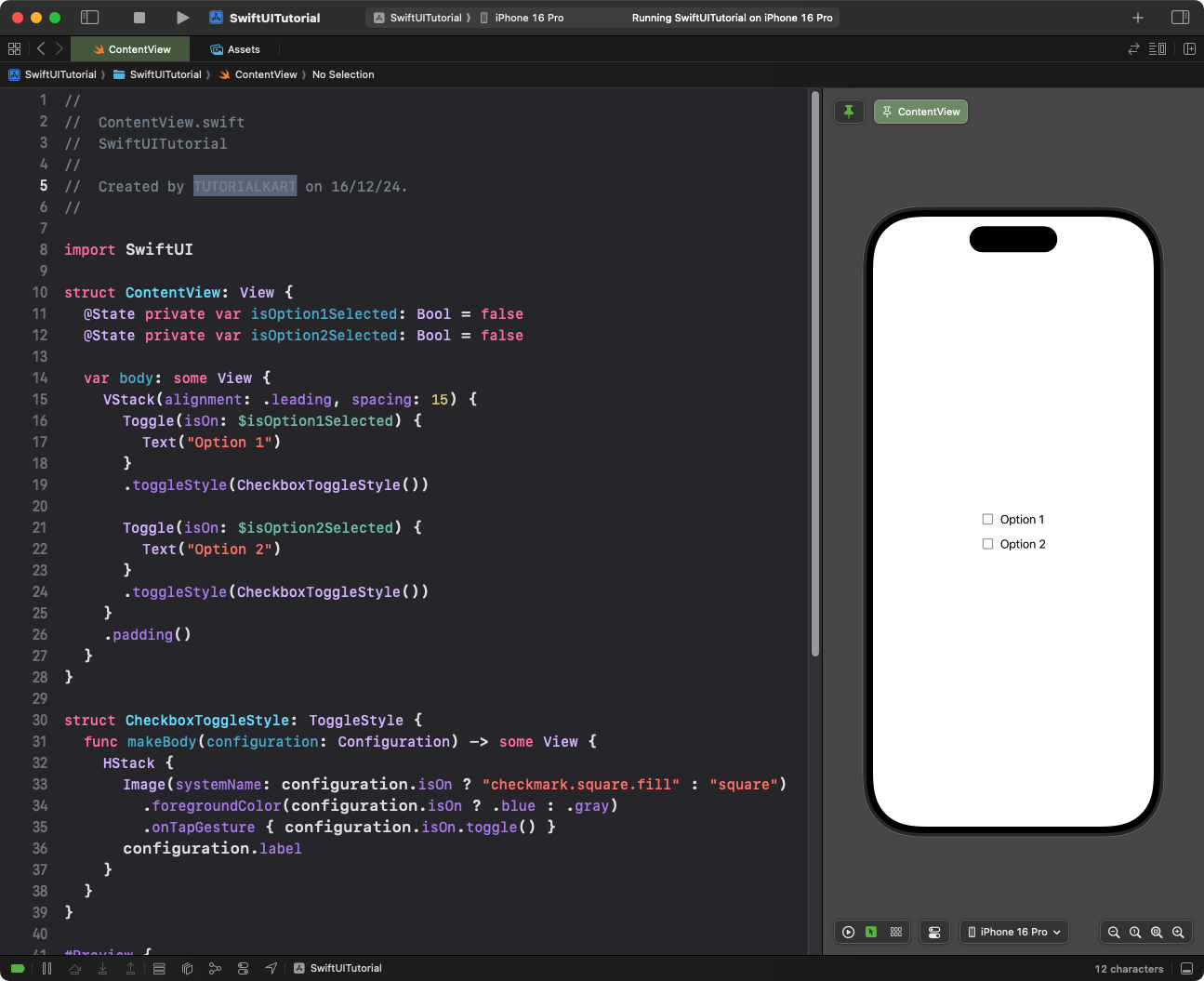
Explanation:
- Each toggle represents an independent checkbox with its own state variable.
- The
CheckboxToggleStyle
is reused to create the same checkbox appearance for each option.
Result: Multiple checkbox-style toggles allow users to select or deselect individual options.
Conclusion
By customizing the Toggle
control using a custom ToggleStyle
, you can easily create checkboxes in SwiftUI. These checkboxes provide familiar functionality for selecting and deselecting options, enhancing the user experience in forms and settings.