SwiftUI – VStack
In SwiftUI, the VStack
is a layout container that arranges its child views vertically in a column. It provides an easy way to create vertical layouts and automatically adapts to the available space, making it a core component for building user interfaces in SwiftUI.
In this SwiftUI tutorial, explains the basic usage of VStack
, its customisation options, and practical examples to help you build vertical layouts effectively.
Basic Syntax for VStack
The basic syntax for creating a VStack
is:
VStack(alignment: .center, spacing: 10) {
// Add views here
}
Here:
alignment
: Aligns the child views along the horizontal axis (.leading
,.center
, or.trailing
). The default is.center
.spacing
: Specifies the vertical space between the child views. The default is determined by the system.- The content block contains the views to be arranged vertically.
Each view inside the VStack
is placed in the order it’s defined.
Examples
Let’s explore how to use VStack
with practical examples.
Example 1: Simple VStack
This example demonstrates a basic VStack
with three text views:
Code Example:
import SwiftUI
struct ContentView: View {
var body: some View {
VStack {
Text("Item 1")
Text("Item 2")
Text("Item 3")
}
.padding()
}
}
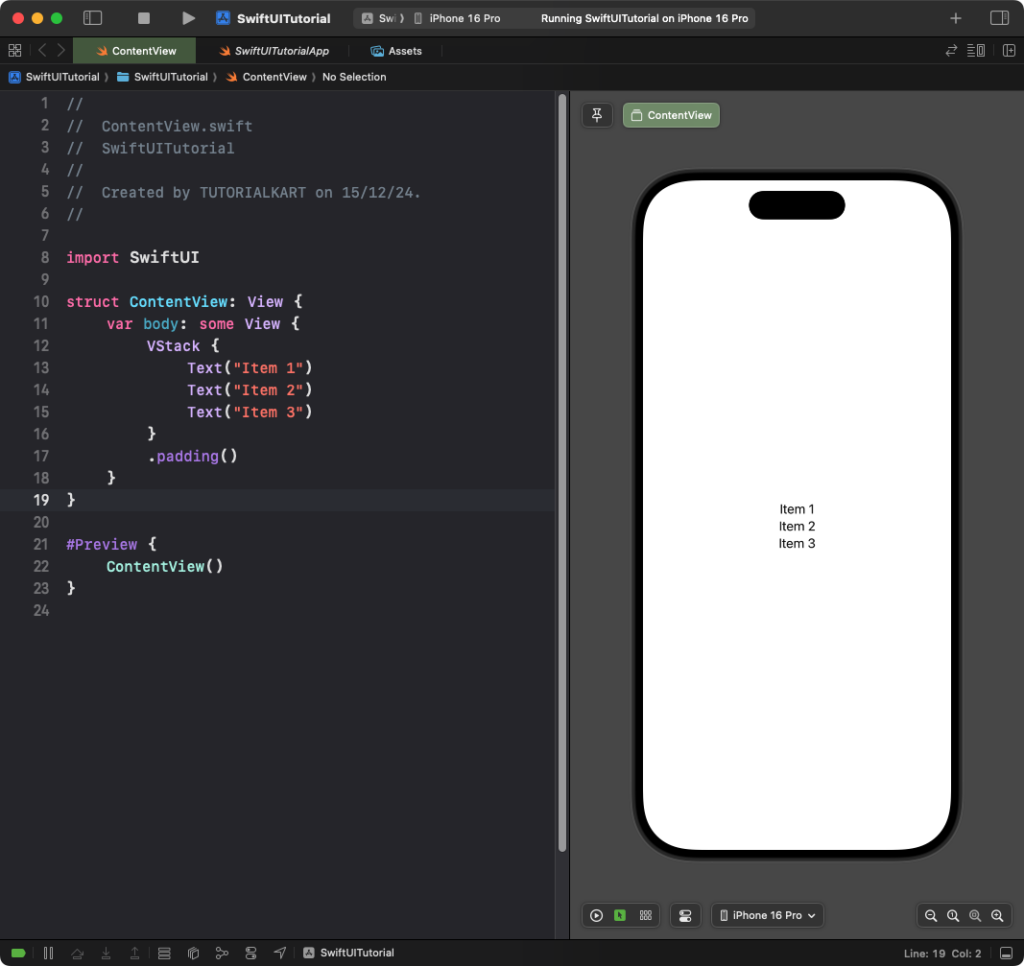
Explanation:
- The
VStack
arranges the text views vertically in the order they’re defined. - The
.padding()
modifier adds space around the entire stack.
Result: Three text items are displayed in a vertical column.
Example 2: Customising Alignment and Spacing
You can customize the alignment and spacing of the views in the VStack
:
Code Example:
import SwiftUI
struct ContentView: View {
var body: some View {
VStack(alignment: .leading, spacing: 20) {
Text("Item 1")
.padding()
.background(Color.blue.opacity(0.3))
.cornerRadius(8)
Text("Item 2")
.padding()
.background(Color.green.opacity(0.3))
.cornerRadius(8)
Text("Item 3")
.padding()
.background(Color.orange.opacity(0.3))
.cornerRadius(8)
}
.padding()
}
}
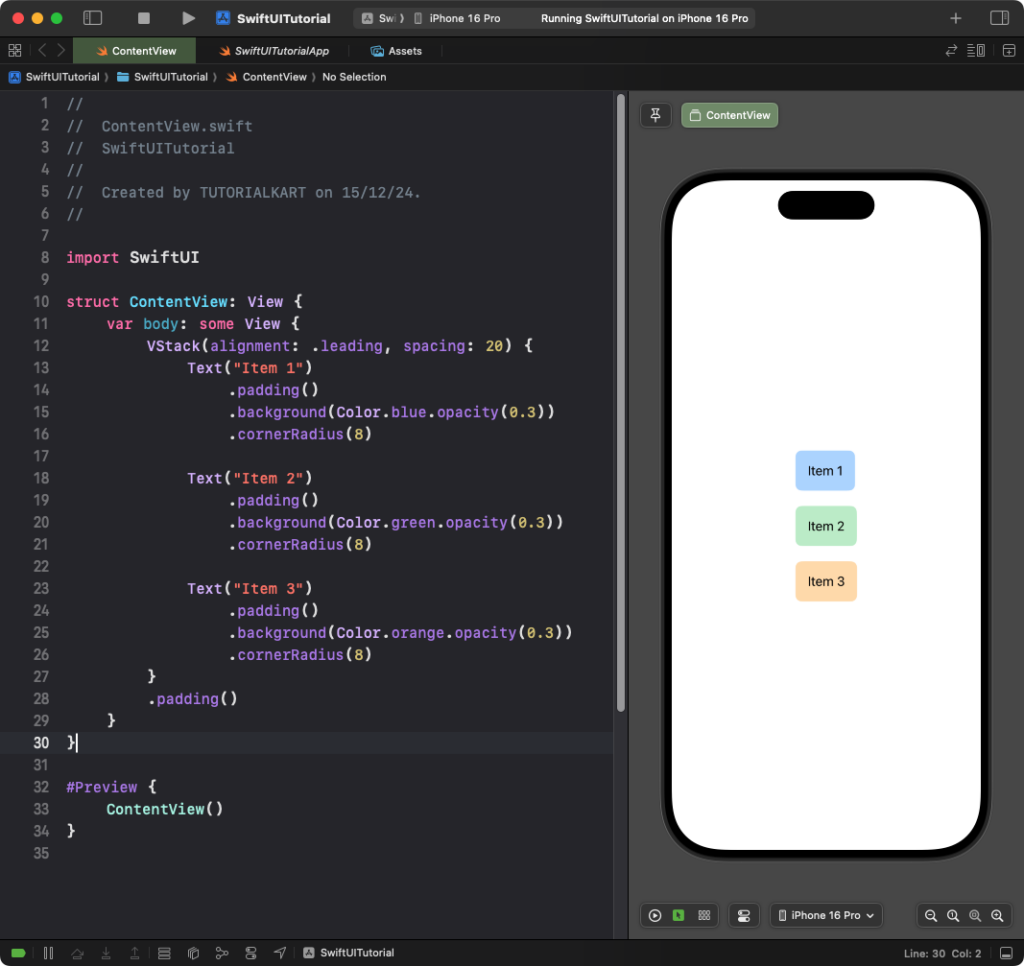
Explanation:
alignment: .leading
: Aligns the child views to the left.spacing: 20
: Adds 20 points of vertical space between each view.- Each item has a colored background and rounded corners for visual distinction.
Result: Three items are displayed in a vertical column, aligned to the left with equal spacing between them.
Example 3: VStack with Spacer
Use Spacer
to distribute content within the VStack
:
Code Example:
import SwiftUI
struct ContentView: View {
var body: some View {
VStack {
Text("Top")
Spacer()
Text("Bottom")
}
.padding()
}
}
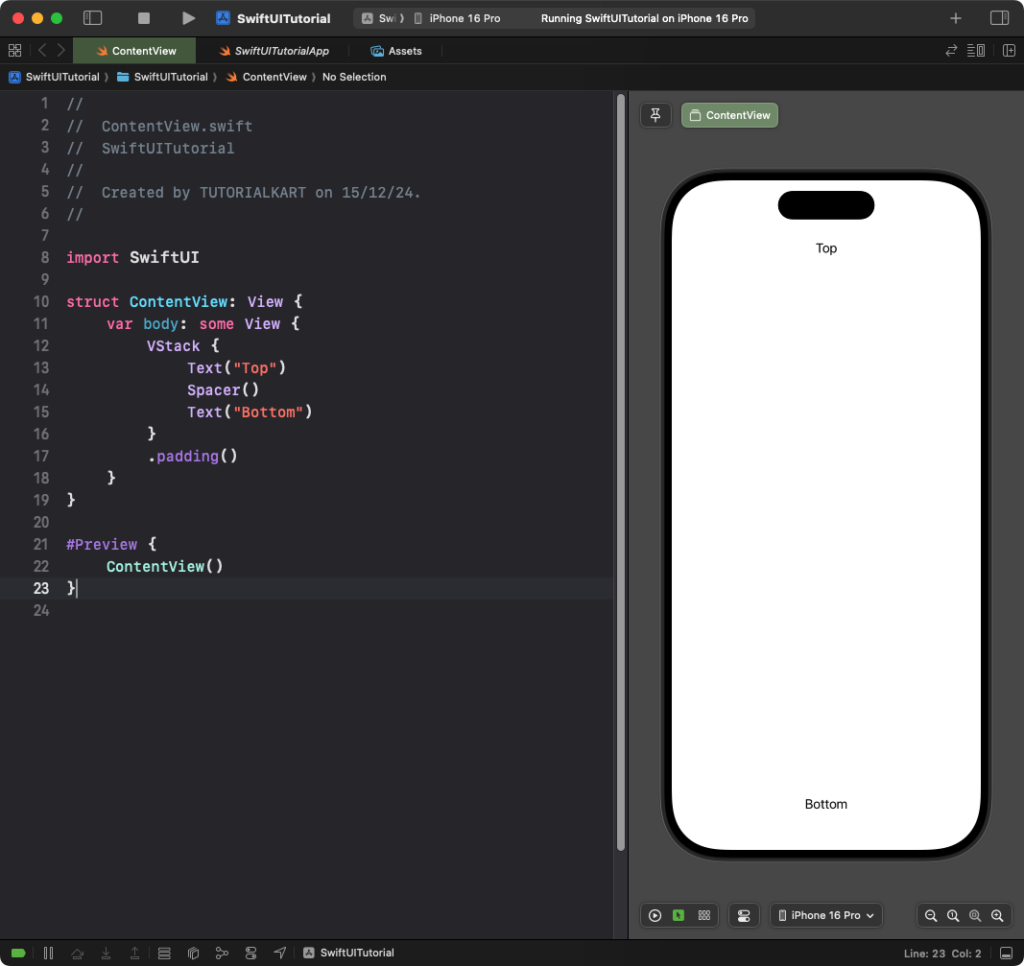
Explanation:
- The
Spacer
pushes the “Top” text to the top and the “Bottom” text to the bottom of the screen. - The
.padding()
modifier adds space around the entire stack.
Result: The “Top” text appears at the top, and the “Bottom” text appears at the bottom, with a spacer in between.
Conclusion
The VStack
is a versatile and essential layout container in SwiftUI for arranging views vertically. By using alignment, spacing, and other layout modifiers like Spacer
, you can create dynamic and visually appealing vertical layouts for your app.