C break
C break statement makes the execution to come out of the loop or break the loop even before the loop condition becomes false.
You can write a C break statement only inside a looping statement like while, do-while or for loop and switch statement.
In this tutorial, we will learn how to use break statement inside C looping statements and switch statement.
C break statement inside while loop
break statement when executed inside C While Loop, makes the execution come out of the while loop. Following flow diagram illustrates the execution flow with break statement inside a while loop.
Usually, break statement inside any looping statement is surrounded by if condition. Otherwise, it would break the loop in the first iteration itself.
Now, let write a C program, with a break statement in while loop. In the following program, we will write a while loop to print numbers from 0 to 10. Also, we will include a conditional statement where when the i
equals 5
, we will execute break statement.
C Program
#include <stdio.h>
void main() {
int i = 0;
while (i<=10) {
if(i == 5) {
break;
}
printf("%d\n", i);
i++;
}
}
Without break statement, the loop would have printed until i
is 10.
Output
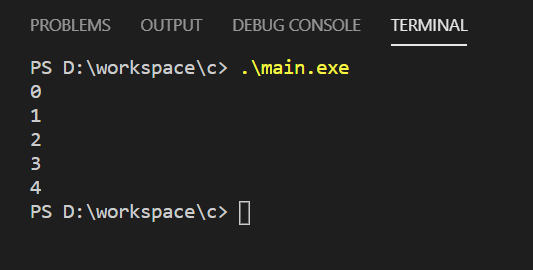
C Break statement in For Loop
Similar to Break statement in While loop, you can have break statement in C For Loop.
All the explanation that we provided in the above section holds for break statement in for loop as well. So, let us dive into an example.
In the following example, we shall try to print i
in for loop, but if i
equals 5
, we would like to break out of the loop.
C Program
#include <stdio.h>
void main() {
for (int i = 0; i <= 10; i++) {
if(i == 5) {
break;
}
printf("%d\n", i);
}
}
Output
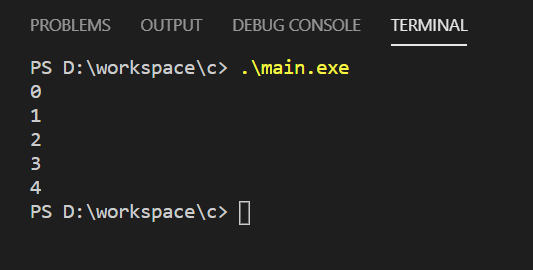
C Break statement inside Switch statement
You may refer C Switch statement to better understand the role of break statement in Switch-Case statement.
In the following example, we will write a switch statement with break inside case blocks.
C Program
#include <stdio.h>
void main() {
int a;
printf("Enter number, a : ");
scanf("%d", &a);
int b;
printf("Enter number, b : ");
scanf("%d", &b);
printf("\n1. Addition");
printf("\n2. Subtraction");
printf("\n3. Multiplication\n\n");
int choice;
printf("Enter choice : ");
scanf("%d", &choice);
switch (choice) {
case 1:
printf("%d + %d = %d", a, b, (a + b));
break;
case 2:
printf("%d - %d = %d", a, b, (a - b));
break;
case 3:
printf("%d * %d = %d", a, b, (a * b));
break;
default:
printf("Invalid Choice.");
}
}
Output
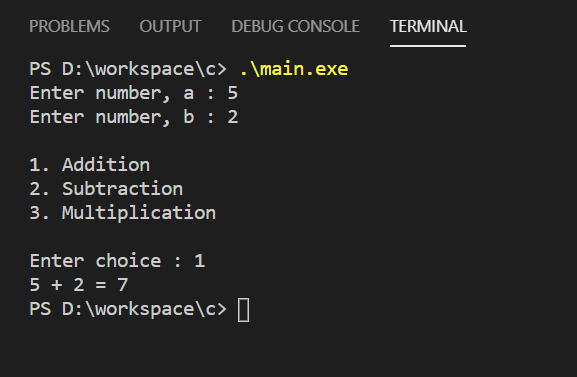
Conclusion
In this C Tutorial, we learned how to use a break statement inside looping statement and switch statement, with example programs.