C If Else Statement
C If Else statement is kind of an extension to C If Statement. In C If statement, we have seen that execution of a block of statements depends on a condition. In If Else statement, we have one more block, called else block, which executes when the condition is false.
So, in C if-else statement, we have an if block followed by else block. If the condition is true, if block is executed, and if the condition is false, else block is executed. Based on the output of condition, only one of the block is executed.
Syntax of C If-Else statement
Following is the syntax of C if else statement.
if (condition) { //if block statement(s) } else { //else block statement(s) }
where
if is C builtin keyword.
condition is a boolean value or an expression that evaluates to boolean value. The condition is generally formed using comparison operators and logical operators.
() parenthesis around the condition is mandatory.
if block statement(s) is a set of statements. You can have none, one or multiple statements inside if block.
{} flower braces wrap around if block statement(s). These are optional only in case that there is only one statement inside if block. Otherwise, these braces are mandatory.
else is C builtin keyword.
else block statement(s) is a set of statements. You can have none, one or multiple statements inside else block.
Flow Diagram of C IF-Else Statement
Following diagram represents the flow of execution for a C if-else statement.
The if-else statement execution starts with evaluating the condition. If the condition evaluates to true, the program executes the if block statement(s) and continues with statements (if any) after the if-else statement.
But, if the condition evaluates to false, the program executes the else block statement(s) and continues with statements (if any) after the if-else statement
Example for C If-Else Statement
In the following example, we have an if-else with condition to check whether the number is even. If the number is even, then we shall print a message to the console that the number is even. This is if block, and have just a single statement. If the number is not even, in the else block, we shall print to the console that number is odd.
C Program
#include <stdio.h> void main() { int a; printf("Enter Number : "); scanf("%d", &a); if (a%2 == 0) { printf("You entered an even number"); } else { printf("You entered an odd number"); } }
Here the condition is a%2 == 0
. It is a boolean expression formed using comparison operator and it evaluates to either true or false.
Output
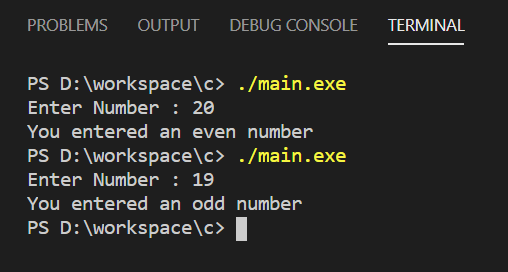
Conclusion
In this C Tutorial, we learned what an If-Else statement is in C programming language, how its execution happens, and how it works with an example program.