C Factorial Program
C Factorial Program – You can find Factorial of a Number in C programming, in many ways. In this tutorial, we will go through following list of processes.
- Factorial using Recursion.
- Factorial using While Loop.
- Factorial using For Loop.
Factorial using Recursion
You can calculate factorial of a given number using recursion technique in C programming.
The steps to find factorial using recursion are:
- Read number from user.
- Write a function called factorial.
- If the argument is less than 2, the function should return 1.
- Else, the function should return the product of argument and factorial(argument – 1).
- Call factorial function with the number, read from user, as argument.
C Program
#include <stdio.h>
int factorial(int n) {
if (n<2) {
return 1;
} else {
return n * factorial(n - 1);
}
}
int main() {
int n;
printf("Enter a number : ");
scanf("%d", &n);
int result = factorial(n);
printf("Factorial of %d is %d", n, result);
}
Output
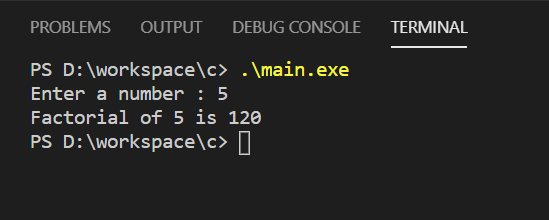
Factorial using While Loop
By looking at the formula of factorial, we can use looping technique to find the result of factorial for a given number.
In the following program, we will use C While Loop to find factorial
The steps to find factorial using while loop are:
- Read number n from user. We shall find factorial for this number.
- Initialize two variables: result to store factorial, and i for loop control variable.
- Write while condition i <= n. We have to iterate the loop from i=1 to i=n.
- Compute result = result * i during each iteration.
- Increment i.
- After while loop execution, result contains the factorial of the number n.
C Program
#include <stdio.h>
int main() {
int n;
printf("Enter a number : ");
scanf("%d", &n);
int result = 1;
int i = 1;
while (i <= n) {
result *= i;
i++;
}
printf("Factorial of %d is %d", n, result);
}
Output
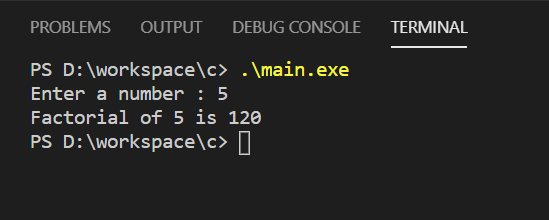
Factorial using For Loop
Similar to that of While loop in the previous example, we can also use C For Loop to find the factorial of a number.
The steps to find factorial using for loop are:
- Read number n from user. We shall find factorial for this number.
- Initialize variable result to store factorial
- In for loop initialization, initialize i=1 for loop control variable. In the update section of for loop, increment i.
- Write for loop condition i <= n.
- Compute result = result * i during each iteration.
- After for loop execution, result contains the factorial of the number n.
C Program
#include <stdio.h>
int main() {
int n;
printf("Enter a number : ");
scanf("%d", &n);
int result = 1;
for (int i = 1; i <= n; i++) {
result *= i;
}
printf("Factorial of %d is %d", n, result);
}
Output
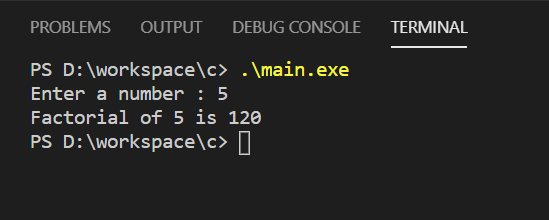
Conclusion
In this C Tutorial, we learned to write C program to compute Factorial of a given number.