fprintf() Function
The fprintf()
function in C writes formatted data to a specified output stream. It allows you to combine static text with variable data by using format specifiers within a format string. This function is extremely useful for logging and file output operations where the formatted presentation of data is essential.
Syntax of fprintf()
int fprintf(FILE *stream, const char *format, ...);
Parameters
Parameter | Description |
---|---|
stream | A pointer to a FILE object that identifies the output stream. |
format | A C string containing the text to be written to the stream. It can include embedded format specifiers that will be replaced by the additional arguments. |
The function processes the format string and replaces any format specifiers with the corresponding additional arguments, formatting the output accordingly. It supports a variety of specifiers such as %d
, %f
, %s
, and more, allowing for flexible output formatting.
Return Value
On success, fprintf()
returns the total number of characters written. If a writing error occurs, it returns a negative number, and the error indicator for the stream is set accordingly.
Examples for fprintf()
Example 1: Writing Simple Formatted Text to a File
This example demonstrates how to use fprintf()
to write a simple formatted message to a file.
Program
#include <stdio.h>
int main() {
FILE *fp = fopen("example1.txt", "w");
if (fp == NULL) {
perror("Error opening file");
return 1;
}
// Write a simple message to the file
fprintf(fp, "Hello, fprintf!\n");
fclose(fp);
return 0;
}
Explanation:
- The file
example1.txt
is opened in write mode. fprintf()
writes the formatted string “Hello, fprintf!\n” to the file.- The file is closed after writing.
Program Output:
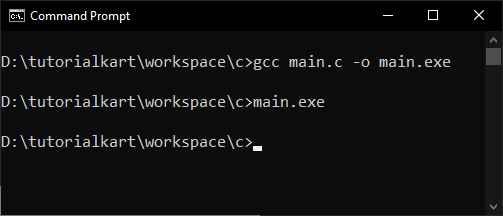
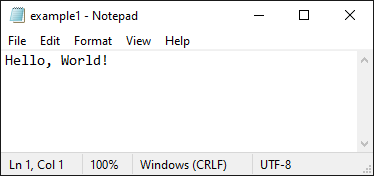
Example 2: Writing Formatted Numeric Data
This example shows how to format and write numeric data to a file using various format specifiers.
Program
#include <stdio.h>
int main() {
FILE *fp = fopen("example2.txt", "w");
if (fp == NULL) {
perror("Error opening file");
return 1;
}
int num = 42;
double pi = 3.14159;
fprintf(fp, "Integer: %d\n", num);
fprintf(fp, "Floating-point: %.2f\n", pi);
fclose(fp);
return 0;
}
Explanation:
- The file
example2.txt
is opened in write mode. - An integer and a floating-point number are defined.
fprintf()
writes the integer using the%d
specifier and the floating-point number using the%.2f
specifier to format the output with two decimal places.- The file is closed after writing.
Program Output:
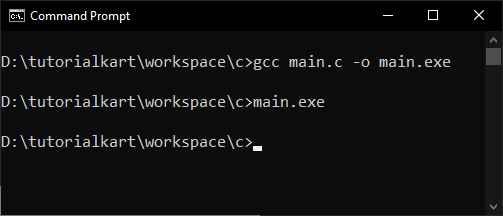
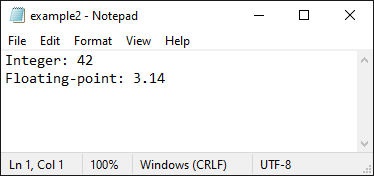
Example 3: Writing Mixed Data Types
This example illustrates how to combine different data types into a single formatted output to a file.
Program
#include <stdio.h>
int main() {
FILE *fp = fopen("example3.txt", "w");
if (fp == NULL) {
perror("Error opening file");
return 1;
}
char name[] = "Arjun";
int age = 30;
double salary = 55000.75;
fprintf(fp, "Name: %s\n", name);
fprintf(fp, "Age: %d years\n", age);
fprintf(fp, "Salary: $%.2f per year\n", salary);
fclose(fp);
return 0;
}
Explanation:
- The file
example3.txt
is opened in write mode. - A string, an integer, and a floating-point number are defined.
fprintf()
is used to write each variable with appropriate format specifiers:%s
for the string,%d
for the integer, and%.2f
for the floating-point number formatted to two decimal places.- The file is closed after writing.
Output:
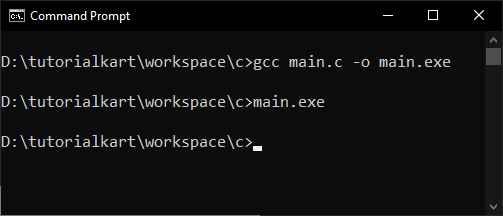
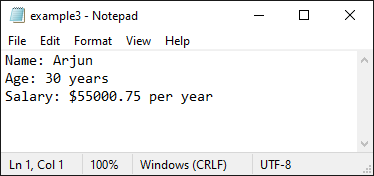
Example 4: Handling File Opening Errors
This example demonstrates how to check for errors when opening a file and using fprintf()
to report the error.
Program
#include <stdio.h>
int main() {
FILE *fp = fopen("nonexistent_directory/example4.txt", "w");
if (fp == NULL) {
fprintf(stderr, "Error: Unable to open file for writing.\n");
return 1;
}
fprintf(fp, "This will not be printed if file open fails.\n");
fclose(fp);
return 0;
}
Explanation:
- The program attempts to open a file in a directory that does not exist.
- If
fopen()
fails, it returnsNULL
and an error message is printed to the standard error stream usingfprintf()
withstderr
. - The program exits with an error code.
Program Output:
Error: Unable to open file for writing.