fputc() Function
The fputc()
function in C writes a single character to an output stream, updating the stream’s internal position as it does so. It is typically used for outputting data one character at a time, which can be useful in various file and console operations.
Syntax of fputc()
int fputc(int character, FILE *stream);
Parameters
Parameter | Description |
---|---|
character | An integer representing the character to be written, which is internally converted to an unsigned char. |
stream | A pointer to a FILE object that identifies the output stream. |
The function writes the given character to the specified stream at the current position and then advances the stream’s position indicator by one. It is essential for the stream to be properly opened for writing before calling fputc()
.
Return Value
On success, fputc()
returns the character written. In the event of a writing error, it returns EOF
and sets the error indicator for the stream.
Examples for fputc()
Example 1: Writing a Single Character to a File
This example demonstrates how to write a single character to a file using fputc()
.
Program
#include <stdio.h>
int main() {
FILE *fp = fopen("output.txt", "w");
if (fp == NULL) {
perror("Error opening file");
return 1;
}
// Write a single character 'A' to the file
fputc('A', fp);
fclose(fp);
return 0;
}
Explanation:
- A file named
output.txt
is opened in write mode. fputc()
writes the character'A'
to the file.- The file is closed to ensure the data is saved properly.
Program Output:
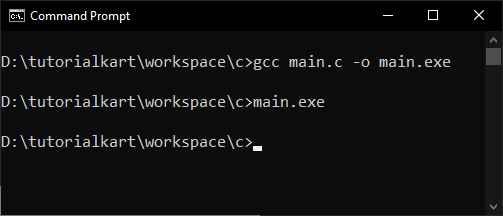
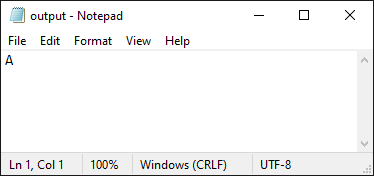
Example 2: Writing a String Character-by-Character to a File
This example illustrates how to write a complete string to a file by using fputc()
to output each character individually.
Program
#include <stdio.h>
int main() {
FILE *fp = fopen("output.txt", "w");
if (fp == NULL) {
perror("Error opening file");
return 1;
}
char str[] = "Hello, World!";
for (int i = 0; str[i] != '\0'; i++) {
fputc(str[i], fp);
}
fclose(fp);
return 0;
}
Explanation:
- A file named
output.txt
is opened in write mode. - A string
"Hello, World!"
is defined. - A loop iterates over the string, writing each character to the file using
fputc()
. - The file is closed after writing all characters.
Program Output:
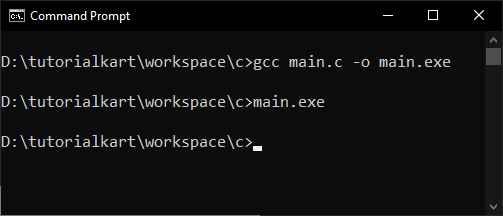
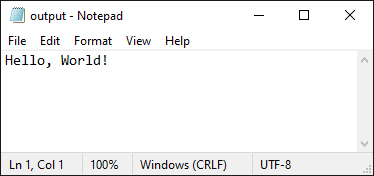
Example 3: Handling Write Errors with fputc()
This example demonstrates error handling by attempting to write to a file that could not be opened.
Program
#include <stdio.h>
int main() {
// Attempt to open a file in a non-existent directory to simulate an error
FILE *fp = fopen("nonexistent_dir/output.txt", "w");
if (fp == NULL) {
perror("Error opening file");
return 1;
}
// Attempt to write a character
if (fputc('B', fp) == EOF) {
perror("Error writing to file");
}
fclose(fp);
return 0;
}
Explanation:
- The program attempts to open a file in a non-existent directory, which results in an error.
fopen()
returnsNULL
, triggering the error message fromperror()
.- The program exits without attempting to write any character.
Program Output:
Error opening file: No such file or directory