fscanf() Function
The fscanf()
function in C reads formatted data from a stream, interpreting input based on a specified format. It stores the extracted values into already allocated memory locations using additional arguments. This function is used for parsing input from files or other input streams and supports a wide range of format specifiers, including those for integers, floating-point numbers, characters, strings, and even pointer addresses.
Syntax of fscanf()
int fscanf(FILE *stream, const char *format, ...);
Parameters
Parameter | Description |
---|---|
stream | Pointer to a FILE object that identifies the input stream to read data from. |
format | A C string containing a sequence of characters that specify how to interpret the input data. This includes literal characters, whitespace, and format specifiers. |
… | A variable number of additional arguments, each a pointer to an allocated storage location where the extracted data is stored. |
Important points to note about fscanf()
include its behavior with whitespace characters and literal characters in the format string. Whitespace in the format string causes the function to skip any whitespace in the input stream. Literal characters must match exactly, or the function fails. The format specifiers determine how the input is interpreted and what type of data is expected, so it is crucial that the additional arguments point to correctly allocated memory of the corresponding types.
Return Value
On success, fscanf()
returns the number of input items successfully matched and assigned. This number may be less than the expected count if a matching failure occurs. If a reading error or end-of-file is encountered before any matching occurs, EOF is returned.
Examples for fscanf()
Example 1: Basic File Reading
This example demonstrates how to use fscanf()
to read formatted data from a file, extracting an integer, a floating-point number, and a string.
data.txt
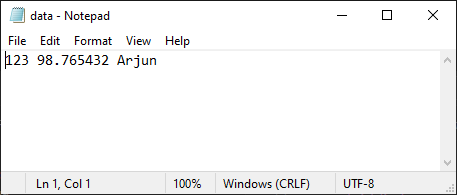
Program
#include <stdio.h>
int main() {
FILE *fp = fopen("data.txt", "r");
if (fp == NULL) {
perror("Error opening file");
return 1;
}
int id;
float score;
char name[50];
// Reading an integer, a float, and a string from the file
if (fscanf(fp, "%d %f %s", &id, &score, name) == 3) {
printf("ID: %d\n", id);
printf("Score: %f\n", score);
printf("Name: %s\n", name);
} else {
printf("Error reading data.\n");
}
fclose(fp);
return 0;
}
Explanation:
- The program opens the file
data.txt
for reading. - It declares variables to store an integer, a float, and a string.
- The
fscanf()
function is used to read these values from the file. - If all three values are successfully read, they are printed to the console.
- If reading fails, an error message is displayed.
Program Output:
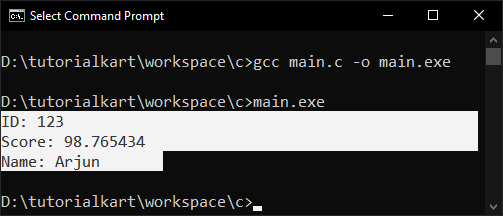
Example 2: Using Scanset with fscanf()
This example uses a scanset in the format string to read a string until a whitespace is encountered, allowing controlled input of non-whitespace characters.
input.txt
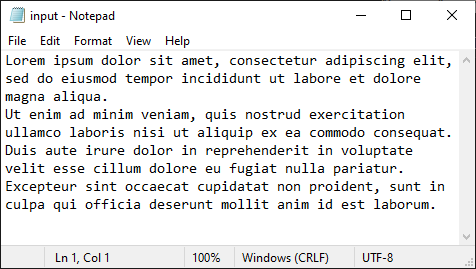
Program
#include <stdio.h>
int main() {
FILE *fp = fopen("input.txt", "r");
if (fp == NULL) {
perror("Error opening file");
return 1;
}
char word[30];
// Reads a sequence of non-whitespace characters from the file
if (fscanf(fp, "%29[^\n]", word) == 1) {
printf("Word read: %s\n", word);
} else {
printf("No valid word found.\n");
}
fclose(fp);
return 0;
}
Explanation:
- The program opens the file
input.txt
for reading. - A character array
word
is defined to store the input string. - The format specifier
%29[^\n]
reads up to 29 characters until a newline is encountered. - The read word is then printed to the console.
Program Output:
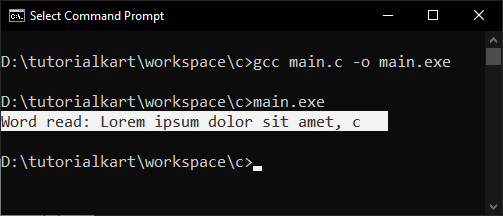
Example 3: Ignoring Input Using the Asterisk Specifier
This example demonstrates how to ignore certain inputs using the asterisk (*) in the format specifier, allowing the program to skip unwanted data.
mixed.txt
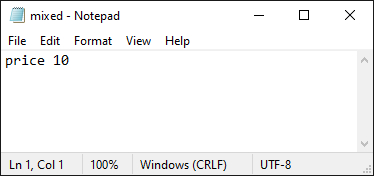
Program
#include <stdio.h>
int main() {
FILE *fp = fopen("mixed.txt", "r");
if (fp == NULL) {
perror("Error opening file");
return 1;
}
int number;
// The %*s specifier reads a string but does not store it
if (fscanf(fp, "%*s %d", &number) == 1) {
printf("Number read: %d\n", number);
} else {
printf("Failed to read the number.\n");
}
fclose(fp);
return 0;
}
Explanation:
- The program opens the file
mixed.txt
for reading. - The format specifier
%*s
is used to read and ignore a string from the file. - The next value, an integer, is read and stored in
number
. - The read number is printed to the console using printf().
Program Output:
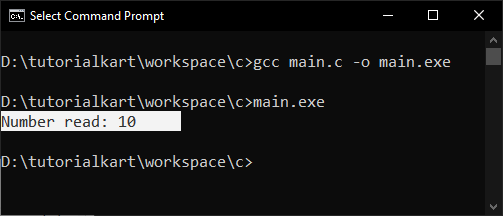