fseek() Function
The fseek()
function in C repositions the stream position indicator for a given file stream. It is used to set the pointer to a specific location in a file, which is particularly useful when you need to navigate through files in both binary and text modes. This function clears the end-of-file indicator and discards any effects from previous ungetc calls, ensuring that subsequent I/O operations work correctly.
Syntax of fseek()
int fseek(FILE *stream, long int offset, int origin);
Parameters
Parameter | Description |
---|---|
stream | A pointer to a FILE object that identifies the stream. |
offset |
For binary files: The number of bytes to offset from the reference position. For text files: Either zero or a value returned by ftell() .
|
origin |
The reference position used for the offset. It can be one of the following constants:
|
It is worth noting that for streams opened in text mode, the offset must either be zero or a value returned by ftell()
, and the origin must be SEEK_SET
. For binary streams, the offset is simply added to the reference position specified by the origin.
Return Value
If fseek()
is successful, it returns zero. Otherwise, it returns a non-zero value, and if an error occurs during reading or writing, the error indicator is set for the stream.
Examples for fseek()
Example 1: Repositioning in a Binary File
This example demonstrates how to use fseek()
to move the file pointer in a binary file to a specified offset from the beginning of the file.
Program
#include <stdio.h>
int main() {
FILE *fp = fopen("example.bin", "rb");
if (fp == NULL) {
perror("Error opening file");
return 1;
}
// Move the file pointer 10 bytes from the beginning (SEEK_SET)
if (fseek(fp, 10L, SEEK_SET) != 0) {
perror("fseek failed");
fclose(fp);
return 1;
}
// Further file operations can be performed here
printf("File pointer repositioned successfully.\n");
fclose(fp);
return 0;
}
Explanation:
- The program opens a binary file named “example.bin” in read mode.
fseek()
is used to move the file pointer 10 bytes from the beginning of the file (SEEK_SET
).- Error checking is performed to ensure the pointer was repositioned successfully.
- A success message is printed if the operation is successful.
Program Output:
File pointer repositioned successfully.
Example 2: Using fseek in a Text File to Reposition for Reading
This example shows how to use fseek()
with a text file example.txt, where the offset is zero or a value obtained from ftell()
, to reposition the file pointer before reading data.
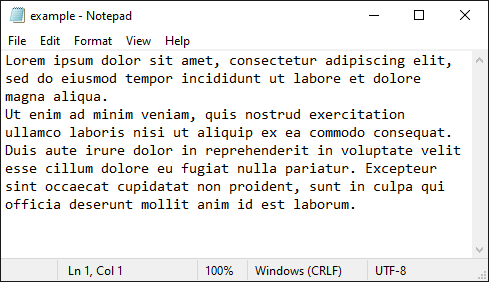
Program
#include <stdio.h>
int main() {
FILE *fp = fopen("example.txt", "r");
if (fp == NULL) {
perror("Error opening file");
return 1;
}
// Read and display first 20 characters
char buffer[21] = {0};
fread(buffer, sizeof(char), 20, fp);
printf("Initial read: %s\n", buffer);
// Reposition to the beginning using ftell value 0 and SEEK_SET
if (fseek(fp, 0L, SEEK_SET) != 0) {
perror("fseek failed");
fclose(fp);
return 1;
}
// Read again from the beginning
fread(buffer, sizeof(char), 20, fp);
printf("Re-read after fseek: %s\n", buffer);
fclose(fp);
return 0;
}
Explanation:
- The program opens a text file “example.txt” in read mode.
- It reads the first 20 characters and prints them.
fseek()
is called with an offset of 0 andSEEK_SET
to reposition the file pointer to the beginning of the file.- The file is read again from the beginning and the output is printed.
Program Output:
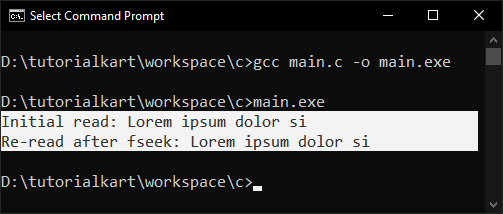
Example 3: Moving to the End of a File and Back to the Beginning
This example demonstrates repositioning the file pointer to the end of the file using SEEK_END
and then moving it back to the beginning with SEEK_SET
, which is useful for determining file size or re-reading a file.
Program
#include <stdio.h>
int main() {
FILE *fp = fopen("example.txt", "r");
if (fp == NULL) {
perror("Error opening file");
return 1;
}
// Move to the end of the file
if (fseek(fp, 0L, SEEK_END) != 0) {
perror("fseek failed");
fclose(fp);
return 1;
}
// Optionally, you can use ftell() here to get the file size
// Reposition back to the beginning
if (fseek(fp, 0L, SEEK_SET) != 0) {
perror("fseek failed");
fclose(fp);
return 1;
}
printf("File pointer repositioned back to the beginning.\n");
fclose(fp);
return 0;
}
Explanation:
- The program opens the text file “example.txt” in read mode.
fseek()
is used withSEEK_END
to move the file pointer to the end of the file.- The pointer is then repositioned to the beginning using
fseek()
with an offset of 0 andSEEK_SET
. - A success message is printed indicating the pointer has been repositioned.
Program Output:
File pointer repositioned back to the beginning.