fsetpos() Function
The fsetpos()
function in C is used to restore the position indicator of a file stream to a previously saved location. This function is particularly useful when you need to return to a specific point in a file after performing some operations. It also clears the end-of-file indicator and discards any effects from previous calls to ungetc()
, ensuring a clean state for subsequent file operations. On streams open for update (read+write), this function helps in switching between reading and writing.
Syntax of fsetpos()
int fsetpos(FILE *stream, const fpos_t *pos);
Parameters
Parameter | Description |
---|---|
stream | Pointer to a FILE object that identifies the stream. |
pos | Pointer to an fpos_t object that contains a file position obtained previously with fgetpos() . |
The fsetpos()
function resets the internal file position indicator to the position stored in the object pointed to by pos
. This function is particularly effective when you need to re-read data or switch between different modes of operation in a file stream.
Return Value
If successful, the fsetpos()
function returns zero. On failure, it returns a nonzero value and sets errno
to a system-specific positive value.
Examples for fsetpos()
Example 1: Restoring File Position After Reading
This example demonstrates how to save and restore the file position using fgetpos()
and fsetpos()
after reading data from a file example.txt.
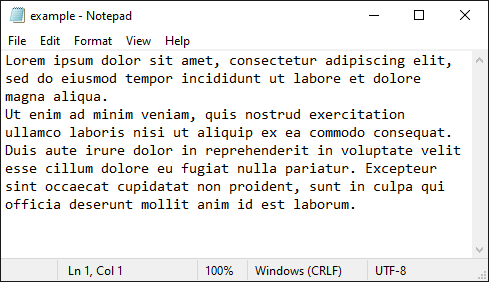
Program
#include <stdio.h>
int main() {
FILE *fp = fopen("example.txt", "r");
if (fp == NULL) {
perror("Error opening file");
return 1;
}
fpos_t pos;
char buffer[100];
// Save the current file position
if (fgetpos(fp, &pos) != 0) {
perror("Error getting file position");
fclose(fp);
return 1;
}
// Read the first line from the file
fgets(buffer, sizeof(buffer), fp);
printf("First read: %s", buffer);
// Restore the file position to the saved state
if (fsetpos(fp, &pos) != 0) {
perror("Error setting file position");
fclose(fp);
return 1;
}
// Read the line again after resetting position
fgets(buffer, sizeof(buffer), fp);
printf("\nSecond read: %s", buffer);
fclose(fp);
return 0;
}
Explanation:
- The file
"example.txt"
is opened in read mode. - The current file position is saved using
fgetpos()
intopos
. - The first line is read from the file and printed.
fsetpos()
restores the file position to the saved state.- The line is read again from the beginning and printed, demonstrating that the position was reset.
Program Output:
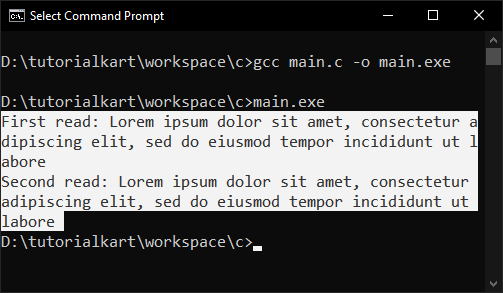
Example 2: Switching Between Reading and Writing in Update Mode
This example illustrates how fsetpos()
is useful in a file data.txt opened in update mode (read+write), allowing the program to switch between reading and writing operations.
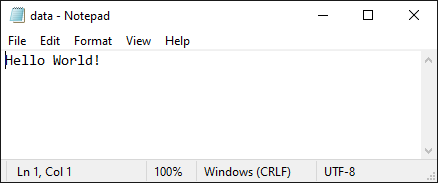
Program
#include <stdio.h>
int main() {
FILE *fp = fopen("data.txt", "r+");
if (fp == NULL) {
perror("Error opening file");
return 1;
}
fpos_t pos;
// Save the current position in the file
if (fgetpos(fp, &pos) != 0) {
perror("Error getting file position");
fclose(fp);
return 1;
}
// Read a line from the file
char buffer[100];
fgets(buffer, sizeof(buffer), fp);
printf("Read: %s", buffer);
// Restore the position to switch to writing mode
if (fsetpos(fp, &pos) != 0) {
perror("Error setting file position");
fclose(fp);
return 1;
}
// Write new data at the restored position
fputs("Hello Arjun!\n", fp);
fclose(fp);
return 0;
}
Explanation:
- The file
"data.txt"
is opened in update mode. - The current file position is saved using
fgetpos()
. - A line is read from the file and printed.
fsetpos()
restores the file position, allowing the program to switch back to writing at the original position.- New data is written into the file, replacing or appending to the original content based on the file mode.
Program Output:
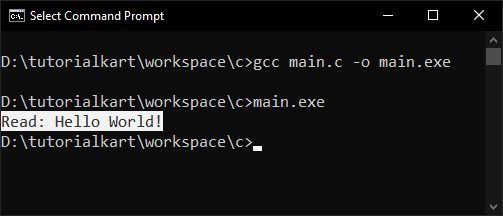
Updated data.txt file:
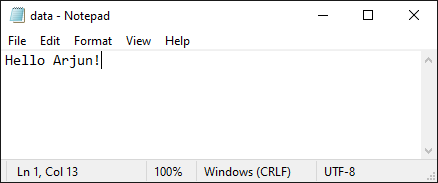
Example 3: Clearing End-of-File Indicator with fsetpos()
This example demonstrates how fsetpos()
clears the end-of-file indicator, allowing further reading from a file after reaching EOF.
Program
#include <stdio.h>
int main() {
FILE *fp = fopen("data.txt", "r");
if (fp == NULL) {
perror("Error opening file");
return 1;
}
fpos_t pos;
// Save the position before reading
if (fgetpos(fp, &pos) != 0) {
perror("Error getting file position");
fclose(fp);
return 1;
}
// Read until EOF
while (!feof(fp)) {
fgetc(fp);
}
printf("Reached EOF.\n");
// Restore position and clear EOF indicator
if (fsetpos(fp, &pos) != 0) {
perror("Error setting file position");
fclose(fp);
return 1;
}
// Read again after clearing EOF
char buffer[100];
fgets(buffer, sizeof(buffer), fp);
printf("After resetting, first line: %s", buffer);
fclose(fp);
return 0;
}
Explanation:
- The file
"log.txt"
is opened in read mode. - The current file position is saved before reading the entire file.
- The program reads characters until the end-of-file is reached.
fsetpos()
restores the file position and clears the EOF indicator.- The program then reads from the restored position, demonstrating that further reading is possible.
Program Output:
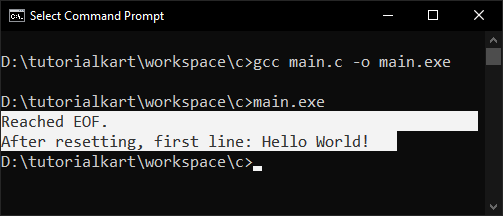