ftell() Function
The ftell()
function in C is used to determine the current position of the file pointer in a stream. It returns the current value of the position indicator, which for binary streams corresponds to the number of bytes from the beginning of the file. For text streams, while the numerical value may not be strictly meaningful, it can still be used to return to the same point later using fseek()
.
Syntax of ftell()
long int ftell(FILE *stream);
Parameters
Parameter | Description |
---|---|
stream | A pointer to a FILE object that identifies the stream. |
It is important to note that when using ftell()
with text streams, the returned value may not represent a byte offset. Nevertheless, it remains useful for saving the position and restoring it later using fseek()
.
Return Value
On success, ftell()
returns the current value of the position indicator. On failure, it returns -1L
and sets errno
to a system-specific positive value.
Examples for ftell()
Example 1: Basic File Position Check After Reading
This example demonstrates how to use ftell()
to check the current position of the file pointer after reading data from a text file example.txt.
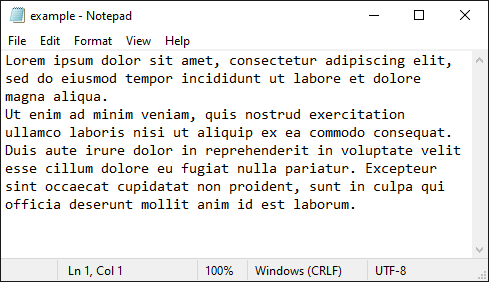
Program
#include <stdio.h>
int main() {
FILE *file = fopen("example.txt", "r");
if (file == NULL) {
perror("Error opening file");
return 1;
}
// Read a character to move the file pointer
fgetc(file);
// Get the current file position
long pos = ftell(file);
if (pos == -1L) {
perror("ftell error");
} else {
printf("Current file position: %ld\n", pos);
}
fclose(file);
return 0;
}
Explanation:
- The program opens a text file named
example.txt
for reading. - A single character is read using
fgetc()
to advance the file pointer. ftell()
is then called to retrieve the current position in the file.- The position is printed, and the file is closed.
Program Output:
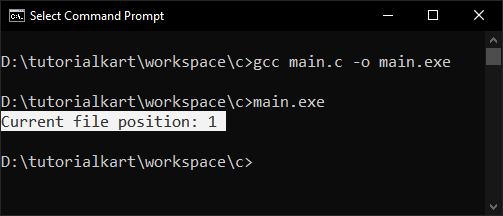
Example 2: Checking File Position in Binary Mode
This example illustrates using ftell()
in binary mode, where the returned position corresponds to the exact byte offset from the beginning of the file.
Program
#include <stdio.h>
int main() {
FILE *file = fopen("binary.dat", "rb");
if (file == NULL) {
perror("Error opening file");
return 1;
}
// Move file pointer to 10 bytes from the beginning
fseek(file, 10, SEEK_SET);
// Get the current file position
long pos = ftell(file);
if (pos == -1L) {
perror("ftell error");
} else {
printf("Current file position: %ld\n", pos);
}
fclose(file);
return 0;
}
Explanation:
- The program opens a binary file named
binary.dat
in read mode. fseek()
is used to set the file pointer 10 bytes from the beginning of the file.ftell()
retrieves the current position, which should be 10.- The position is printed, and the file is closed.
Program Output:
Current file position: 10
Example 3: Saving and Restoring File Position
This example demonstrates how to use ftell()
in conjunction with fseek()
to save the current file position, perform additional operations, and then restore the original position.
Consider the following sample.txt.
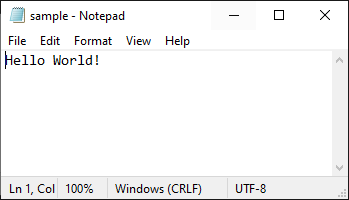
Program
#include <stdio.h>
int main() {
FILE *file = fopen("sample.txt", "r");
if (file == NULL) {
perror("Error opening file");
return 1;
}
// Save the current file position
long initialPos = ftell(file);
if (initialPos == -1L) {
perror("ftell error");
fclose(file);
return 1;
}
// Read some characters
for (int i = 0; i < 5; i++) {
fgetc(file);
}
// Get the new file position after reading
long newPos = ftell(file);
if (newPos == -1L) {
perror("ftell error");
} else {
printf("New file position after reading: %ld\n", newPos);
}
// Restore the original file position
fseek(file, initialPos, SEEK_SET);
long restoredPos = ftell(file);
if (restoredPos == -1L) {
perror("ftell error");
} else {
printf("Restored file position: %ld\n", restoredPos);
}
fclose(file);
return 0;
}
Explanation:
- The program opens a file named
sample.txt
in read mode. ftell()
is used to save the initial file position.- The program reads five characters from the file, moving the file pointer forward.
ftell()
then retrieves the new file position, which is printed.fseek()
restores the file pointer to the initial saved position.ftell()
confirms that the file position has been restored.
Program Output:
New file position after reading: 5
Restored file position: 0