fwrite() Function
The fwrite()
function in C writes a block of data from memory to a file stream. It writes an array of elements from a memory block to the current position in the file and advances the file pointer by the total number of bytes written.
Syntax of fwrite()
size_t fwrite(const void *ptr, size_t size, size_t count, FILE *stream);
Parameters
Parameter | Description |
---|---|
ptr | Pointer to the array of elements to be written, converted to a const void* . |
size | Size in bytes of each element to be written. (size_t is an unsigned integral type.) |
count | Number of elements, each of size bytes, to be written. (size_t is an unsigned integral type.) |
stream | Pointer to a FILE object that specifies an output stream. |
Internally, fwrite()
treats the memory block pointed by ptr
as an array of unsigned char elements and writes them sequentially to the file stream. The stream’s position indicator is advanced by the total number of bytes written.
Return Value
The function returns the total number of elements successfully written. If this number is less than the count
parameter, a writing error occurred, and the error indicator for the stream is set. If either size
or count
is zero, the function returns zero and the error indicator remains unchanged.
Examples for fwrite()
Example 1: Writing a Simple String to a File
This example demonstrates using fwrite()
to write a string to a file.
Program
#include <stdio.h>
#include <string.h>
int main() {
FILE *fp = fopen("output.txt", "w");
if (fp == NULL) {
perror("Error opening file");
return 1;
}
char str[] = "Hello, World!";
size_t elementsWritten = fwrite(str, sizeof(char), strlen(str), fp);
if (elementsWritten != strlen(str)) {
perror("Error writing to file");
}
fclose(fp);
return 0;
}
Explanation:
- The file
output.txt
is opened for writing. - A string
"Hello, World!"
is defined. fwrite()
writes the string to the file, one character at a time.- The program verifies that the number of elements written matches the expected count.
Program Output:
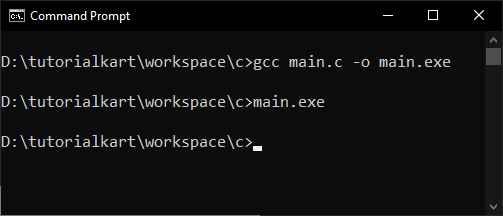
The file “output.txt” will contain:
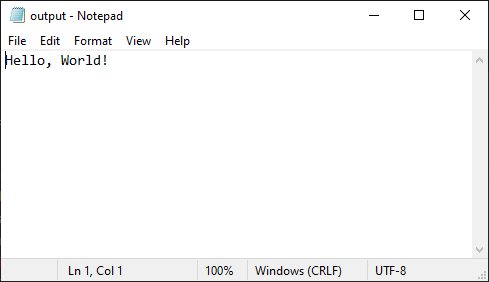
Example 2: Writing an Array of Integers to a Binary File
This example shows how to use fwrite()
to write an array of integers to a binary file.
Program
#include <stdio.h>
int main() {
FILE *fp = fopen("numbers.bin", "wb");
if (fp == NULL) {
perror("Error opening file");
return 1;
}
int numbers[] = {1, 2, 3, 4, 5};
size_t count = sizeof(numbers) / sizeof(numbers[0]);
size_t elementsWritten = fwrite(numbers, sizeof(int), count, fp);
if (elementsWritten != count) {
perror("Error writing to file");
}
fclose(fp);
return 0;
}
Explanation:
- The binary file
numbers.bin
is opened for writing. - An integer array
{1, 2, 3, 4, 5}
is defined. fwrite()
writes the entire array to the file in binary format.- The program checks that the expected number of elements were successfully written.
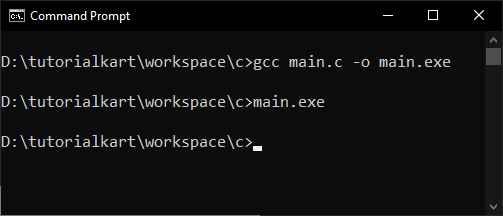
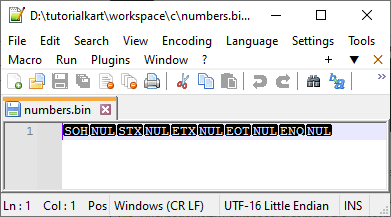
Example 3: Writing an Array of Structures to a Binary File
This example demonstrates how to write an array of structures to a binary file using fwrite()
.
Program
#include <stdio.h>
typedef struct {
int id;
char name[20];
} Record;
int main() {
FILE *fp = fopen("records.bin", "wb");
if (fp == NULL) {
perror("Error opening file");
return 1;
}
Record records[] = {
{1, "Alice"},
{2, "Bob"},
{3, "Charlie"}
};
size_t count = sizeof(records) / sizeof(records[0]);
size_t elementsWritten = fwrite(records, sizeof(Record), count, fp);
if (elementsWritten != count) {
perror("Error writing to file");
}
fclose(fp);
return 0;
}
Explanation:
- The binary file
records.bin
is opened for writing. - A structure
Record
is defined, and an array of such structures is initialized. fwrite()
writes the entire array of structures to the file in binary format.- The program verifies that the number of elements written matches the expected count.
Program Output:
The file “records.bin” will contain the binary representation of the array of structures.
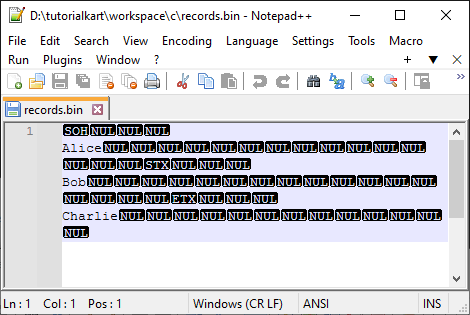