getc() Function
The getc()
function in C is used to read a single character from a given input stream. It reads the character at the stream’s current position and then advances the internal file position indicator. This function can return a valid character (promoted to an int) or the special value EOF
when the end-of-file is reached or an error occurs.
Syntax of getc()
int getc(FILE *stream);
Parameters
Parameter | Description |
---|---|
stream | Pointer to a FILE object that identifies the input stream. |
It is important to note that because getc()
may be implemented as a macro, the stream expression should be free of side effects.
Return Value
On success, getc()
returns the character read from the stream (promoted to an int
). If the end-of-file is reached or a read error occurs, it returns EOF
and sets the appropriate indicator for the stream.
Examples for getc()
Example 1: Reading a Single Character from a File
This example demonstrates how to open a file sample.txt and use getc()
to read its first character.
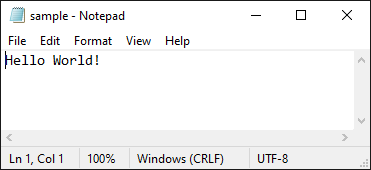
Program
#include <stdio.h>
int main() {
FILE *fp = fopen("sample.txt", "r");
if (fp == NULL) {
printf("Error opening file.\n");
return 1;
}
int ch = getc(fp);
if (ch != EOF) {
printf("First character: %c\n", ch);
} else {
printf("Reached end-of-file or error occurred.\n");
}
fclose(fp);
return 0;
}
Explanation:
- The file
sample.txt
is opened in read mode. getc()
reads the first character from the file.- If a character is read successfully, it is printed; otherwise, an error message is displayed.
- The file is closed after the operation.
Program Output:
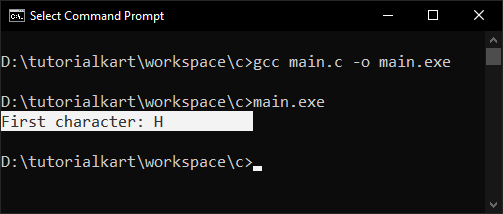
First character: H
Example 2: Reading Characters Until End-of-File
This example illustrates how to continuously read characters from a file using getc()
until the end-of-file is reached.
Program
#include <stdio.h>
int main() {
FILE *fp = fopen("sample.txt", "r");
if (fp == NULL) {
printf("Error opening file.\n");
return 1;
}
int ch;
while ((ch = getc(fp)) != EOF) {
putchar(ch);
}
fclose(fp);
return 0;
}
Explanation:
- The file
sample.txt
is opened in read mode. - A loop uses
getc()
to read each character untilEOF
is encountered. - Each character is printed immediately using
putchar()
. - The file is closed after reaching the end-of-file.
Program Output:
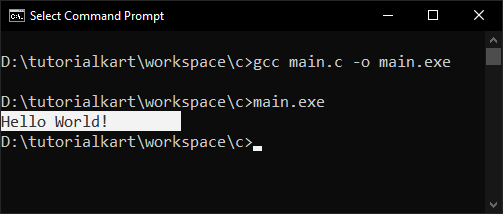
Example 3: Handling End-of-File and Error Conditions
This example demonstrates how to handle cases when a file cannot be opened or when getc()
returns EOF
due to an error or end-of-file condition.
Program
#include <stdio.h>
int main() {
FILE *fp = fopen("nonexistent.txt", "r");
if (fp == NULL) {
printf("Error: Unable to open file.\n");
return 1;
}
int ch = getc(fp);
if (ch == EOF) {
if (feof(fp)) {
printf("End-of-file reached.\n");
} else if (ferror(fp)) {
printf("Read error occurred.\n");
}
}
fclose(fp);
return 0;
}
Explanation:
- An attempt is made to open the file
nonexistent.txt
in read mode. - If the file does not exist, an error message is printed and the program exits.
- If the file were open but
getc()
returnedEOF
, the program would check whether it was due to end-of-file or a read error.
Program Output:
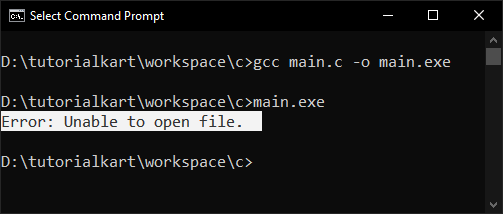
Error: Unable to open file.