C Switch Case Statement
C Switch Case statement is a decision making statement that allows to choose the execution of one of the many case blocks based on the value of switch expression.
C Switch statement is interchangeably used for C Switch Case statement.
In this tutorial, we will learn the syntax of C switch statement, its execution flow using flow diagram, and its usage using example programs.
Syntax of C Switch Statement
The syntax of switch statement in C programming language is given below.
switch (expression) {
case value_1:
// case block 1 statement(s)
break;
case value_2:
// case block 2 statement(s)
break;
default:
// default block statement(s)
}
where
- switch is C keyword.
- expression evaluates to a value.
- case is C keyword.
- value_1, value_2 are the constant values which the expression could evaluate to.
- case block statement(s) are the set of statements that execute when corresponding value matches the expression value.
- default is C keyword.
- case block statement(s) are the set of statements that execute when no case value is matched.
Note: You can have as many case blocks as required.
Flow Diagram of C Switch Case Statement
The execution flow diagram of Switch-Case statement in C is given below.
The step by step process of switch case statement execution is:
- Start switch case statement.
- Evaluate expression’s value.
- Check if expression’s value is equal to value_1. If false go to step 5.
- Execute case block 1 statement(s). Go to step 8.
- Check if expression’s value is equal to value_2. If false go to step 7.
- Execute case block 2 statement(s). Go to step 8.
- Execute default block statement(s).
- End switch-case statement.
As already mentioned, you can have multiple case blocks for your switch statement. The execution flow remains similar to that of how we transitioned from case 1 to case 2.
Example for C Switch Case statement
In the following program, we use switch case statement to implement calculator. We read two numbers and a choice of operation from user. Based on the selected operation, the corresponding case block is executed.
C Program
#include <stdio.h>
void main() {
int a;
printf("Enter number, a : ");
scanf("%d", &a);
int b;
printf("Enter number, b : ");
scanf("%d", &b);
printf("\n1. Addition");
printf("\n2. Subtraction");
printf("\n3. Multiplication\n\n");
int choice;
printf("Enter choice : ");
scanf("%d", &choice);
switch (choice) {
case 1:
printf("%d + %d = %d", a, b, (a + b));
break;
case 2:
printf("%d - %d = %d", a, b, (a - b));
break;
case 3:
printf("%d * %d = %d", a, b, (a * b));
break;
default:
printf("Invalid Choice.");
}
}
Output
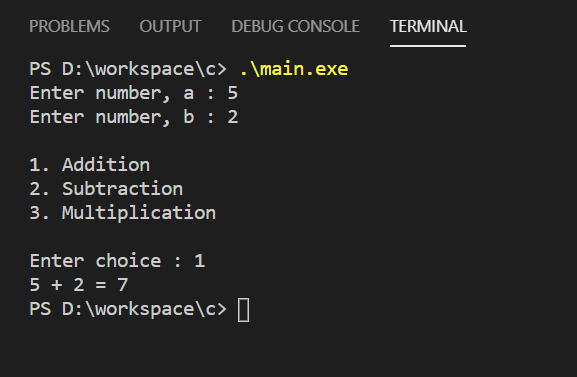
Conclusion
In this C Tutorial, we learned about C Switch-Case statement.