Dart For Loop
Welcome to Dart For Loop tutorial.
In this tutorial, we will learn how to write a for loop and some Dart example programs to understand the usage of Dart For Loop.
Syntax of Dart For Loop
Following is the syntax of For Loop in Dart programming language.
for (initialization; boolean_expression; update) {
//statement(s)
}
initialization
section can contain one or more variables being initialized.
Based on the output of boolean_expression
during each iteration, it is decided whether to execute the statements or not in the for loop. If boolean_expression
evaluates to true
, then the statements inside the for loop are executed. If boolean_expression
evaluates to false
, then the statements inside the for loop are not executed.
update
section can contain update to the variables like increment, decrement or any kind of change in their values.
Working of For Loop
- When the program control comes to a for loop statement, it executes the initialization block.
- And then evaluates the
boolean_expression
.- If
boolean_expression
evaluates totrue
,- then the statements inside the for loop are executed.
- And then the update section is executed.
- Now go to step 2.
- If
boolean_expression
evaluates tofalse
, - Go out of the loop.
- If
Dart For Loop to calculate Factorial of a number
In the following example, we will use Dart For Loop to calculate the factorial of a given number.
Dart program
void main(){
var n = 6;
var factorial = 1;
//for loop to calculate factorial
for(var i=2; i<=n; i++) {
factorial = factorial*i;
}
print('Factorial of ${n} is ${factorial}');
}
Output
Factorial of 6 is 720
Dart Nested For Loop
You can write a For Loop inside another For Loop in Dart. This process is called nesting. Hence Nested For Loop.
Dart For Loop to print * triangle
In the following example, we will use Dart For Loop to *s in the shape of right angle triangle.
Dart program
import 'dart:io';
void main(){
var n = 6;
print('');
for(var i=1; i<=n; i++) {
for(var j=0; j<i; j++) {
stdout.write(' *');
}
print('');
}
}
Output
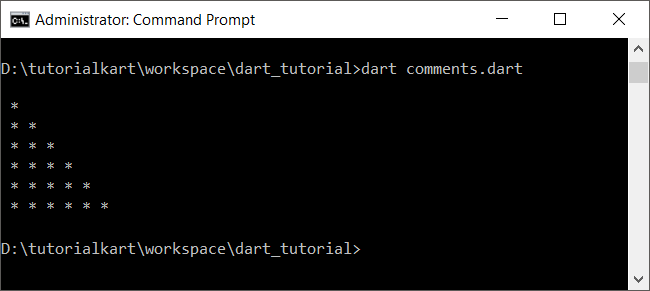
Note: Here we used stdout.write() to write to console without new line at the end, unlike print().
Conclusion
In this Dart Tutorial, we learned the syntax and how to use for loop with the help of example programs.