Java Leap Year Program
An year is said to be leap year if it divisible by 4 and not divisible by 100, with an exception that it is divisible by 400.
In this tutorial, we will write a program to check if given year is leap year.
Algorithm
Following is the algorithm that we shall use to check if given input year is leap year or not.
- Read an integer from user, to year variable.
- Check the condition if year is exactly divisible by 4 and 100, or the year is exactly divisible by 400.
- If the above condition returns true, given year is leap year else it is not leap year.
CheckLeapYear.java
</>
Copy
import java.util.Scanner;
/**
* Java Program - Check Leap Year
*/
public class CheckLeapYear {
public static void main(String[] args) {
//read year from user
Scanner scanner = new Scanner(System.in);
System.out.print("Enter year : ");
int year = scanner.nextInt();
//check if year is leap year
if((year%4 == 0 && year%100 != 0) || (year%400 == 0)) {
System.out.println(year + " is a leap year.");
} else {
System.out.println(year + " is not a leap year.");
}
scanner.close();
}
}
Output
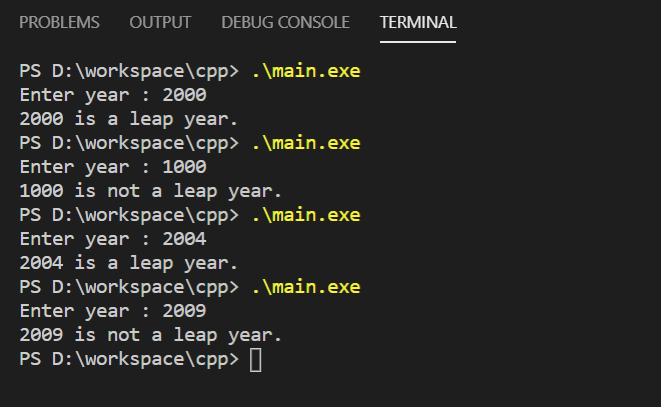
Conclusion
In this Java Tutorial, we learned how to check if given year is leap year or not.