In this Java tutorial, you will learn how to get the list of files and sub-directories in a given folder, with examples.
Java – Filter files or sub-directories in a directory
You can get the list of files and sub-directories in a given folder using Java. We can also filter the list based on extension or a specific condition.
In this Java Tutorial, we shall learn how to perform the following tasks.
- Extract list of files and directories in a folder using java
- Extract list of files belonging to specific file type
- Extract list of files belonging to specific file type, present in the folder and its sub folders/directories
Extract list of files and sub-directories in a directory
Follow these steps to extract the names of files and sub directories in a given directory.
Step 1 : Specify the folder. In this example, “sample” is the folder name placed at the root to the project.
File folder = new File("sample");
Step 2 : Get the list of all items in the folder.
File[] listOfFiles = folder.listFiles();
Step 3 : Check if an item in the folder is a file.
listOfFiles[i].isFile()
Step 4 : Check if an item in the folder is a directory.
listOfFiles[i].isDirectory()
Complete program that lists the files and directories in a folder is given below.
ListOfFilesExample.java
import java.io.File; import java.util.ArrayList; import java.util.List; /** * Program that gives list of files or directories in a folder using Java */ public class ListOfFilesExample { public static void main(String[] args) { List files = new ArrayList<>(); List directories = new ArrayList<>(); File folder = new File("sample"); File[] listOfFiles = folder.listFiles(); for (int i = 0; i < listOfFiles.length; i++) { if (listOfFiles[i].isFile()) { files.add(listOfFiles[i].getName()); } else if (listOfFiles[i].isDirectory()) { directories.add(listOfFiles[i].getName()); } } System.out.println("List of files :\n---------------"); for(String fName: files) System.out.println(fName); System.out.println("\nList of directories :\n---------------------"); for(String dName: directories) System.out.println(dName); } }
The sample folder and its contents are as shown in the below picture :
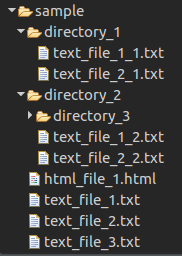
When the program is run, output to the console would be as shown below.
Output
List of files : --------------- html_file_1.html text_file_1.txt text_file_3.txt text_file_2.txt List of directories : --------------------- directory_1 directory_2
Get list of files of specific extension from the directory
Follow these steps.
Step 1 : Specify the folder.
File folder = new File("sample");
Step 2 : Get the list of all items in the folder.
File[] listOfFiles = folder.listFiles();
Step 3 : Check if an item in the folder is a file.
listOfFiles[i].isFile()
Step 4 : Check if the file belong to specified file-type.
Check the extension of the filename with String.endsWith(suffix)
listOfFiles[i].getName().endsWith(fileExtension)
Complete program to get the list of files, that belong to a specified extension type is shown below.
ListOfFilesExample.java
import java.io.File; import java.util.ArrayList; import java.util.List; public class ListOfFilesExample { public static void main(String[] args) { List files = new ArrayList<>(); List directories = new ArrayList<>(); String fileExtension = ".txt"; File folder = new File("sample"); File[] listOfFiles = folder.listFiles(); for (int i = 0; i < listOfFiles.length; i++) { if (listOfFiles[i].isFile()) { if(listOfFiles[i].getName().endsWith(fileExtension)){ files.add(listOfFiles[i].getName()); } } } System.out.println("List of .txt files :\n--------------------"); for(String fName: files) System.out.println(fName); } }
When the program is run, Output to the console would be as shown below.
Output
List of .txt files : -------------------- text_file_1.txt text_file_3.txt text_file_2.txt
Get list of files with specific extension from the directory and its sub-directories
Follow these steps.
Step 1 : Specify the folder.
File folder = new File("D:"+File.separator+"Arjun"+File.separator+"sample");
Step 2 : Get the list of all items in the folder.
File[] listOfFiles = folder.listFiles();
Step 3 : Check if an item in the folder is a file.
listOfFiles[i].isFile()
Step 4 : Check if the file belong to specified file-type.
Check the extension of the filename with String.endsWith(suffix)
listOfFiles[i].getName().endsWith(fileExtension)
Step 5 : Check if an item in the folder is a directory.
listOfFiles[i].isDirectory()
Step 6 : Get the list of files (beloging to specific file-type) recursively from all the sub-directories.
The complete program is given below.
FileListExtractor.java
import java.io.File; import java.util.ArrayList; import java.util.List; public class FileListExtractor { List allTextFiles = new ArrayList(); String fileExtension = ".txt"; public static void main(String[] args) { FileListExtractor folderCrawler = new FileListExtractor(); folderCrawler.crawlFold("sample"); System.out.println("All text files in the folder \"sample\" and its sub directories are :\n---------------------------------------------------------------"); for(String textFileName:folderCrawler.allTextFiles){ System.out.println(textFileName); } } public void crawlFold(String path){ File folder = new File(path); File[] listOfFiles = folder.listFiles(); for (int i = 0; i < listOfFiles.length; i++) { if (listOfFiles[i].isFile()) { if(listOfFiles[i].getName().endsWith(fileExtension)){ allTextFiles.add(listOfFiles[i].getName()); } } else if (listOfFiles[i].isDirectory()) { // if a directory is found, crawl the directory to get text files crawlFold(path+File.separator+listOfFiles[i].getName()); } } } }
When the program is run, output to the console is
Output
All text files in the folder "sample" and its sub directories are : --------------------------------------------------------------- text_file_2_1.txt text_file_1_1.txt text_file_2_1.txt text_file_1_1.txt text_file_2_2.txt text_file_1_2.txt text_file_1.txt text_file_3.txt text_file_2.txt
Conclusion
In this Java Tutorial, we learned how to list all the files and sub-directories in a given folder.