In this tutorial, you will learn how to get the file creation time in Java, using creationTime() method of java.nio.file.attribute.BasicFileAttributes class.
Java – Get file creation time
To get the file creation time of a file in Java, read basic attributes of the file using readAttributes() method of the java.nio.file.Files class, and get the file creation time from the attributes object using creationTime() method.
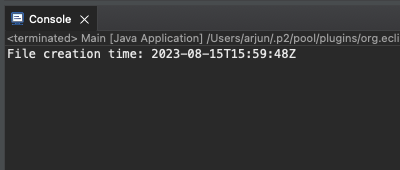
If filePath
is the given file path, then following is the brief code snippet to get the file creation time.
Path filePath = Paths.get("/path/to/file");
BasicFileAttributes attributes = Files.readAttributes(filePath, BasicFileAttributes.class);
FileTime creationTime = attributes.creationTime();
1. Example – Get the file creation time of the file “data.txt” in Java
Let us write a Java program, to get the file creation time of a file at the path "/Users/tutorialkart/data.txt"
, and print it to standard output.
We shall follow these steps.
- Create a Path object filePath from the given file path.
Path filePath = Paths.get("/Users/tutorialkart/data.txt");
- Get the basic attributes of the file using readAttributes() method of the java.nio.file.Files class. Call readAttributes() method and pass the filePath and BasicFileAttributes.class as arguments. The method returns a BasicFileAttributes object. Store the returned value in attributes.
BasicFileAttributes attributes = Files.readAttributes(filePath, BasicFileAttributes.class);
- Get file creation time from the attributes object using creationTime() method of BasicFileAttributes object.
- creationTime() method returns a FileTime object, which represents the file’s time attribute. Store it in creationTime.
FileTime creationTime = attributes.creationTime();
- You may print the creationTime to output using println() method.
- If there is IOException while handling the file, you may address it using a Java Try Catch statement.
The following is a complete working Java program. You may replace the file path, with the file path of your own.
Java Program
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.nio.file.attribute.BasicFileAttributes;
import java.nio.file.attribute.FileTime;
import java.io.IOException;
public class Main {
public static void main(String[] args) {
try {
// Prepare file path
Path filePath = Paths.get("/Users/tutorialkart/data.txt");
// Get file attributes
BasicFileAttributes attributes = Files.readAttributes(filePath, BasicFileAttributes.class);
// Get file creation time
FileTime creationTime = attributes.creationTime();
System.out.println("File creation time: " + creationTime);
} catch (IOException e) {
System.out.println("An error occurred: " + e.getMessage());
}
}
}
Output
File creation time: 2023-08-15T15:59:48Z
Conclusion
In this Java File Operations tutorial, we learned how to get the file creation time of a file using creationTime() method of java.nio.file.attribute.BasicFileAttributes class, with examples.