In this tutorial, you will learn about the structure of a Java program and its key components, illustrated with a practical example.
Structure of a Java Program
A typical Java program is organized into several important elements, including:
- Package Declaration
- Import Statements
- Comments
- Class Definition
- Variables (Class and Local)
- Methods/Routines/Behaviors
The diagram below illustrates these elements, showing how they come together to form the overall structure of a Java program.
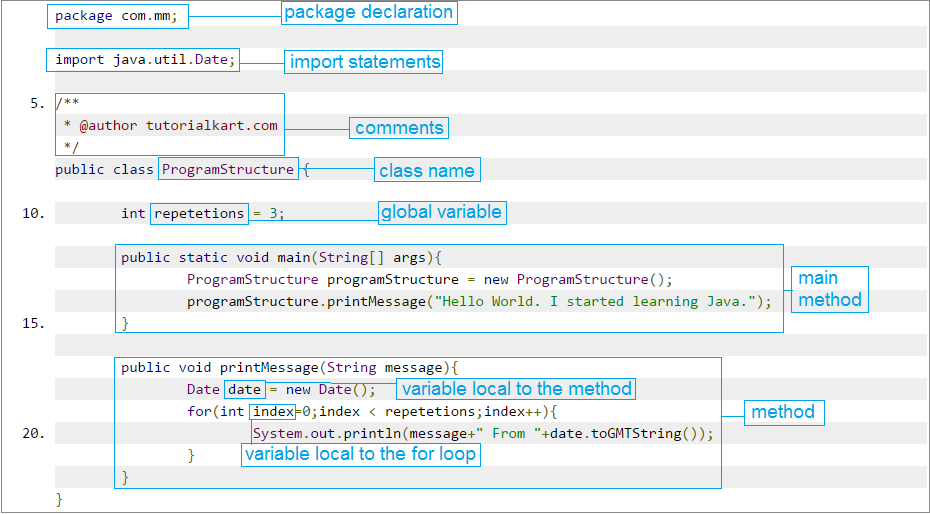
1 Package Declaration
Java classes are often organized into packages, which group related classes together. The package declaration at the top of a Java file defines the directory structure for the classes within a project.
2 Import Statements
Many useful classes reside in different packages or libraries. Import statements allow you to access these external classes in your program. You can include multiple import statements to bring in the functionality you need.
3 Class Definition
Every Java program requires at least one class definition. The class name should match the filename, and it serves as a blueprint for creating objects and defining behavior.
4 Variables
Variables store data values that your program uses during execution. They can be declared at the class level (fields) or within methods (local variables), and their scope determines where they can be accessed. We will explore different types of variables, such as global, local, static, and private, in detail later.
5 Main Method
The main
method is the entry point of any Java application. It is where the program begins execution, loading the bytecode into the Java Runtime Environment (JRE) and running the defined instructions.
6 Methods/Routines/Behaviors
Methods encapsulate a set of instructions that perform specific tasks. By defining methods, you can reuse code and simplify complex operations. Methods may accept parameters (arguments) and return values, allowing you to create modular and maintainable programs.
Conclusion
In this Java Tutorial, we explored the essential components that make up the structure of a Java program. In our next tutorial, we will delve into Object-Oriented Programming concepts, starting with Classes and Objects in Java.