JavaFX Tutorial – We shall build a Basic JavaFX Example Application to understand the basic structure and start working with JavaFX Applications.
Basic JavaFX Example Application
Following is a step by step guide to build Basic JavaFX Example Application.
1. Create a new JavaFX Project.
Follow the clicks :Main Menu ->File ->New ->Project ->JavaFX -> JavaFX Project.
Provide the ‘Project Name‘ – JavaFXExamples and click on Finish.
JavaFXExamples project of type JavaFX would be created.
2. Main Class
By default, Main.java would be created in/src/application/ with necessary code to start the application with an empty window.
Main.java
import javafx.application.Application; import javafx.stage.Stage; import javafx.scene.Scene; import javafx.scene.layout.BorderPane; public class Main extends Application { @Override public void start(Stage primaryStage) { try { BorderPane root = new BorderPane(); Scene scene = new Scene(root,400,400); scene.getStylesheets().add(getClass().getResource("application.css").toExternalForm()); primaryStage.setScene(scene); primaryStage.show(); } catch(Exception e) { e.printStackTrace(); } } public static void main(String[] args) { launch(args); } }
3. Create a Text Message
We shall replace BorderPane with StackPane and add a Text shape to the StackPane. The complete JavaFX Class program is as shown in the following program.
Main.java
import javafx.application.Application; import javafx.stage.Stage; import javafx.scene.Scene; import javafx.scene.layout.StackPane; import javafx.scene.text.Text; public class Main extends Application { @Override public void start(Stage primaryStage) { try { // create a new Text shape Text messageText = new Text("Hello World! Lets learn JavaFX."); // stack page StackPane root = new StackPane(); // add Text shape to Stack Pane root.getChildren().add(messageText); Scene scene = new Scene(root,400,400); scene.getStylesheets().add(getClass().getResource("application.css").toExternalForm()); primaryStage.setScene(scene); primaryStage.show(); } catch(Exception e) { e.printStackTrace(); } } public static void main(String[] args) { launch(args); } }
4. Run the Application
Follow the clicksMain Menu ->Run ->Run.
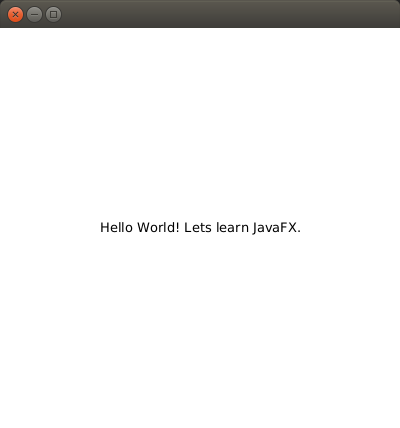
Conclusion
In this JavaFX Tutorial – Basic JavaFX Example Application, we have learnt to create a JavaFX application, display a Text Shape, and run the application.