JavaFX CheckBox
JavaFX CheckBox in GUI allows user to toggle a setting or property or some value.
In this tutorial, we will learn how to initialize a CheckBox control and show it in GUI, then add an action listener to know if the CheckBox is selected or not.
Example 1 – Basic JavaFX CheckBox
To initialize a CheckBox control, you can use CheckBox class as shown below.
CheckBox checkBox = new CheckBox("Some Label");
Following is a basic example to demonstrate how to initialize check box in JavaFX using CheckBox class and show it on a scene.
JavaFxCheckBoxTutorial2.java
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.CheckBox;
import javafx.scene.layout.TilePane;
import javafx.stage.Stage;
public class JavaFxCheckBoxTutorial2 extends Application {
public static void main(String[] args) {
launch(args);
}
@Override
public void start(Stage primaryStage) {
try {
// set title
primaryStage.setTitle("JavaFX Check Box - tutorialkart.com");
//javafx check box
CheckBox checkBox = new CheckBox("TutorialKart");
// tile pane
TilePane tilePane = new TilePane();
// add check box to the tile pane
tilePane.getChildren().add(checkBox);
//set up scene
Scene scene = new Scene(tilePane, 400, 100);
primaryStage.setScene(scene);
primaryStage.show();
} catch(Exception e) {
e.printStackTrace();
}
}
}
Run this Java application and you should see a CheckBox as shown below. You can click on the box or label to toggle the selection.
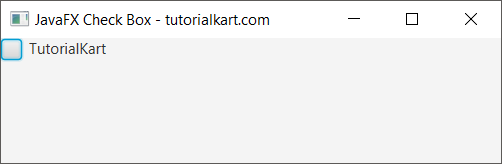
Example 2 – JavaFX CheckBox – Check if Selected
In this example, we will set up an action listener to the CheckBox. The callback function is called when there is a change in the selection (selected or not) for the CheckBox.
JavaFxCheckBoxTutorial.java
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.CheckBox;
import javafx.scene.layout.TilePane;
import javafx.stage.Stage;
public class JavaFxCheckBoxTutorial extends Application {
public static void main(String[] args) {
launch(args);
}
@Override
public void start(Stage primaryStage) {
try {
// set title
primaryStage.setTitle("JavaFX Check Box - tutorialkart.com");
//javafx check box
CheckBox checkBox = new CheckBox("TutorialKart");
//add action listener to check box
checkBox.setOnAction(action -> {
System.out.println("Is CheckBox Selected: "+checkBox.isSelected());
});
// tile pane
TilePane tilePane = new TilePane();
// add check box to the tile pane
tilePane.getChildren().add(checkBox);
//set up scene
Scene scene = new Scene(tilePane, 400, 400);
primaryStage.setScene(scene);
primaryStage.show();
} catch(Exception e) {
e.printStackTrace();
}
}
}
Run this Java application, and click on the check box multiple times. Every time there is a change in the state of the check box, the callback function is called and we are printing if the check box is selected or not.
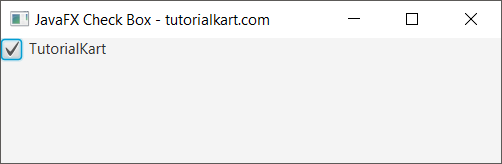
Console output would be as shown below.
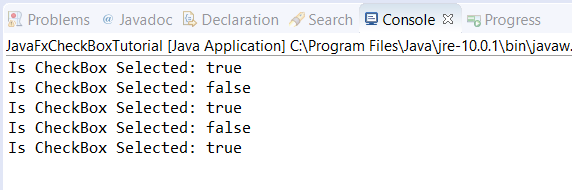
Conclusion
In this JavaFX Tutorial, we learned how to define a JavaFX CheckBox in GUI, and how to set action listener for CheckBox.