Julia Plots
Plots are used to visualize data in a graphical representation. In Julia, Plots can be drawn in different ways.
In this tutorial, we will learn how to draw a Plot in Julia using Plots.jl package.
Install Plots.jl
You have to install Plots.jl package in Julia if you have already not installed.
To install Plots.jl, add “Plots” package using Pkg as shown below:
julia> using Pkg julia> Pkg.add("Plots") Cloning default registries into C:\Users\TutorialKart\.julia\registries Cloning registry General from "https://github.com/JuliaRegistries/General.git" Resolving package versions... Installed Missings ??????????? v0.4.0 Installed PlotThemes ????????? v0.3.0 Installed FixedPointNumbers ?? v0.5.3 . . . Building GR ???? `C:\Users\TutorialKart\.julia\packages\GR\shnUy\deps\build.log` Building Plots ? `C:\Users\TutorialKart\.julia\packages\Plots\qh1wV\deps\build.log`
For the first time, Julia itself compiles the Plots
package for you.
Example – 2D Plot in Julia
In this example, we shall draw a 2D plot in Julia.
Plots package supports multiple backend libraries that actually do the drawing which implement the same API ofcourse. In this example, we will use GR module. GR is essentially based on an implementation of a Graphical Kernel System (GKS) and OpenGL.
script.jl
using Plots #data to plot globaltemperatures = [14.4, 14.5, 14.8, 15.2, 15.5, 15.8]; numindustries = [17, 400, 5000, 15000, 20000, 45000]; #use GR module gr(); #plot plot(numpirates, globaltemperatures, label="line")
Output
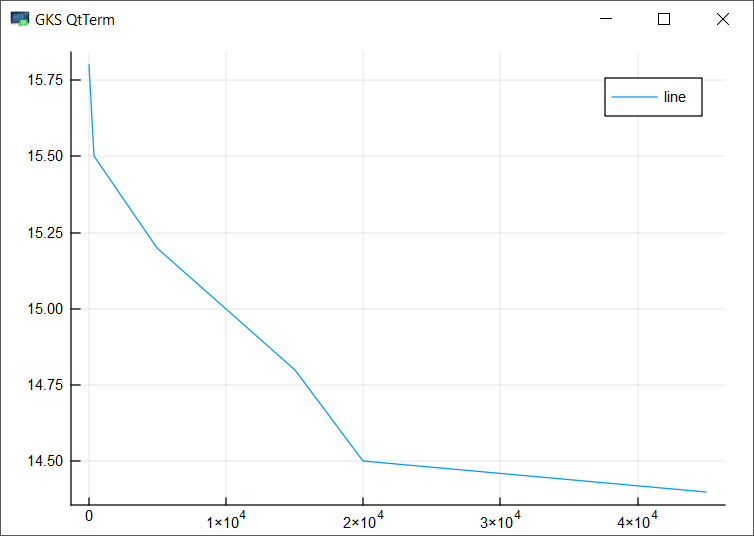
This is just a basic 2D plot. You can add much more information to this plot.
Adding Another Plot to the existing Plot
Let us now add small circles at the points.
We shall use scatter() function. Using ! in scatter!() makes scatter!
a mutating function, indicating that the scattered points will be added onto the pre-existing plot.
script.jl
using Plots #data to plot globaltemperatures = [14.4, 14.5, 14.8, 15.2, 15.5, 15.8]; numindustries = [17, 400, 5000, 15000, 20000, 45000]; #use GR module gr(); #plot plot(numpirates, globaltemperatures, label="line") #add points scatter!(numpirates, globaltemperatures, label="points")
Output
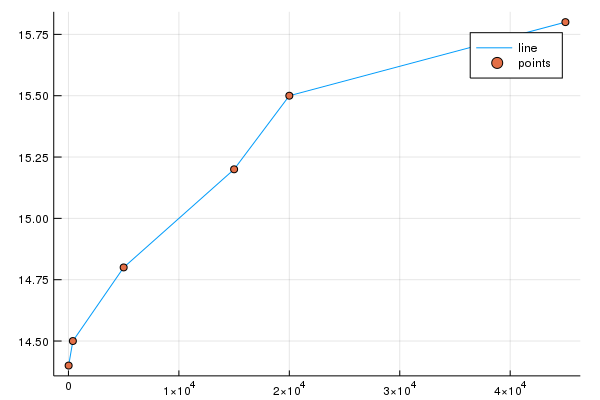
Writing X-label and Y-label
Let us complete this plot with all the basic information. We will add labels to X and Y axes using xlabel() and ylabel() functions.
script.jl
using Plots #data to plot globaltemperatures = [14.4, 14.5, 14.8, 15.2, 15.5, 15.8]; numindustries = [17, 400, 5000, 15000, 20000, 45000]; #use GR module gr(); #plot plot(numindustries, globaltemperatures, label="line") #add points scatter!(numindustries, globaltemperatures, label="points") #adding labels to plot xlabel!("Number of Industries") ylabel!("Global Temperature (°C)") #save plot png("C:\\plot")
X and Y labels are added to the plot.
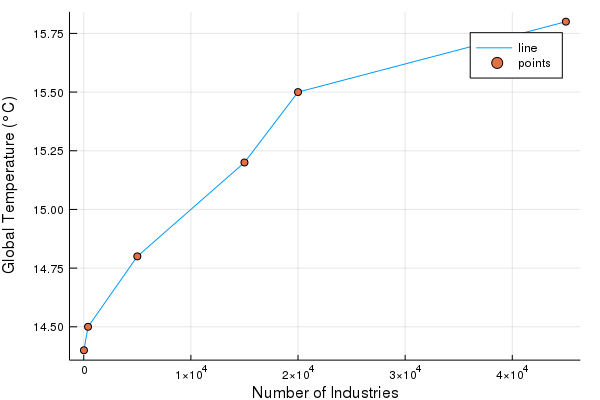
Giving our Plot a Title
To give a title to the plot, use the function title().
script.jl
using Plots #data to plot globaltemperatures = [14.4, 14.5, 14.8, 15.2, 15.5, 15.8]; numindustries = [17, 400, 5000, 15000, 20000, 45000]; #use GR module gr(); #plot plot(numindustries, globaltemperatures, label="line") #add points scatter!(numindustries, globaltemperatures, label="points") #adding labels to plot xlabel!("Number of Industries") ylabel!("Global Temperature (°C)") #title to the plot title!("Influence of Industries on Global Warming") #save plot png("C:\\plot")
Conclusion
In this Julia Tutorial, we learned about Julia Plots.