MongoDB has become one of the must skill set for MEAN stack and backend developers. MongoDB has proven to be the most suited database for the modern applications. Also MongoDB fits well for the Agile methodology. This tutorial presents you with almost all possible MongoDB Interview Questions for basic, intermediate and advanced levels.
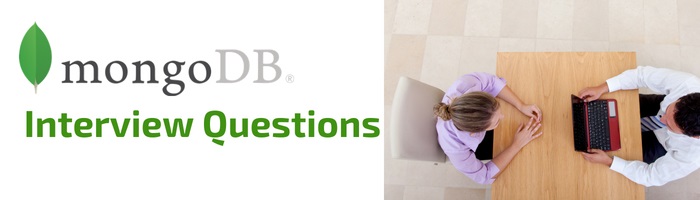
Basic MongoDB Interview Questions
Q1 - What is MongoDB?
MongoDB is a document based NoSQL Database.
Q2 - Why is MongoDB, a NoSQL Database?
A NoSQL Database is one that provides mechanism for storage and retrieval of data that is modeled in means other than tabular relations. Since MongoDB provides a document based (which is again a set of key-value pairs) that is schema-less, MongoDB is a NoSQL Database. And to mention, key-value stores are the simplest NoSQL Databases and MongoDB is popular among them.
Q3 - Could you mention some NoSQL Databases other than MongoDB?
Yes. Of course, there are many. Some of the notable ones are Cassandra, HBASE, CouchDB, IBM Informix, Azure DocumentDB, ToroDB etc.
Q4 - What are the equivalents of Tables and Rows in SQL to that of MongoDB?
MongoDB Collections could be considered as equivalent to Tables and MongoDB Documents could be considered as equivalent to Row of SQL.
Q5 - What specification does MongoDB Document follow?
MongoDB Document follows BSON (Binary JSON) Specification.
Q6 - What are the data types that are allowed for values in key:value pairs of documents?
MongoDB Document follows BSON (Binary JSON) Specification. And the data types allowed are all those that are specified in BSON specification.
Q7 - What should be done when you have to work with large datasets and high data throughput?
MongoDB is horizontally scalable. If the application is expected to handle large datasets and high data throughput, you may add required number of computers (/nodes) to the cluster.
Q8 - Is there something like primary key in MongoDB?
There is no concept of primary key in MongoDB. But there is a key:value pair with key as _id that is used to store a unique value for each Document that is inserted to Collection. By default _id is populated by MongoDB. But _id:value could be provided by user also, as long as the user provides unique value for _id.
Q9 - Coming to Collections, do you think that they can be restricted in size?
Yes. During the creation of collection, we may specify the size limit in Bytes, ie., the number of bytes that can go into that collection. These are also called capped collections. And when we say size, it includes all the data and metadata.
Q10 - Is MongoDB fault tolerant?
Yes. Data Redundancy could be achieved with the help of Replica Set. With MongoDB Replica Set, data could be replicated over specified number of nodes in the cluster. And during that scenario, even if a node fails, there are others with data replicated already. Although, it has to be noted that if a transaction is stored in a DB and just before replicating it to others the node goes down, the transaction data is lost. This is unavoidable with MongoDB.
Q11 - Can MongoDB be used for real time analytics?
Yes. MongoDB’s low latency and ability to analyze semi-structured or unstructured data make it as really a good choice for real time analytics.
Q12 - How is MongoDB better than Relational Databases?
MongoDB has many benefits over Relational Databases, Some of them are :
- MongoDB can handle structured data(like a spread-sheet or table), semi structured data and unstructured data (data with no rules, examples are multimedia content).
- All the NoSQL features of MongoDB makes it a perfect fit for Agile methodology during application development. Modern applications are designed to have fewer development time and quick updates to the ever ending requirements.
- MongoDB is horizontally scalable. Hence it can respond to the increasing application requirements with less hardware cost.
Q13 - What is Replication in MongoDB?
Replication is the process of making copies of data (documents) across multiple MongoDB instances. This helps in failover scenarios. When a node goes down, the data is still present on the other nodes and can be served without any application outage.
Q14 - What is MapReduce in MongoDB?
MapReduce is an algorithm used for deducing some useful information and trends from the available data. It is generally used in data analytics associated with Big Data. MongoDB has the implementation of MapReduce Algorithm and the funcitonality can be accessed through db.collection.mapReduce()
.
Q15 - What are the requirements that ring a bell to use MongoDB for an application?
Try to answer the following questions, and if you get an YES for most of them, choose MongoDB.
- Is your data structured or not ? Structured !
- Does your application need scalability? horizontally in particular !
- Do you want your schema design to be flexible ?
- Does you data objects move around the application as JSON ?
- You do not require JOINS on different collections ?
Q16 - How do you start a Mongo Shell?
Mongo Daemon should be started in prior.
To start Mongo Daemon, run the following command :
~$ sudo service mongod start
Once Mongo Daemon is up and running, we can start a Mongo Shell using mongo
command in terminal.
~$ mongo
Q17 - What is the default port on which Mongo Daemon starts?
By default, unless modified through any means, Mongo Daemon starts on the port 12707
.
Q18 - How do you connect to a Mongo instance running on another computer in the network?
Host and Port options of mongo command can be used to connect to mongod instance running on different node connected to the network.
<pre class="wp-block-syntaxhighlighter-code"> ~$ <span class="crayon-v">mongo</span> <span class="crayon-o">--</span><span class="crayon-v">host</span> <span class="crayon-o"><</span><span class="crayon-v">host</span><span class="crayon-o">></span> <span class="crayon-o">--</span><span class="crayon-v">port</span> <span class="crayon-o"><</span><span class="crayon-v">port_number</span><span class="crayon-o">></span></pre>
Q19 - Can you run multiple Mongo Instances on the same computer?
Yes. Using different port number for each Mongo Instance, multiple instances can be started.
Q20 - Among the multiple Mongo Instances, how do you connect to a specific Mongo Daemon instance?
As Mongo Instances could be differentiated by port number, we can use the same information to start a mongo shell with the --port
option.
<pre class="wp-block-syntaxhighlighter-code"> ~$ <span class="crayon-v">mongo </span><span class="crayon-o">--</span><span class="crayon-v">port</span> <span class="crayon-o"><</span><span class="crayon-v">port_number</span><span class="crayon-o">></span></pre>
Q21 - What is a MongoDB Database?
In MongoDB, Database is a collection of MongoDB Collections.
Tutorial – MongoDB Database.
Q22 - How do you start working on a specific MongoDB Database through mongo shell?
use <database_name>
command in mongo shell can be used to connect to a specific MongoDB Database.
Q23 - What is the command to list available Databases in a MongoDB instance?
To get the list of databases, open Mongo Shell and run the show dbs
command.
Q24 - How do you create a MongoDB Database?
MongoDB Database can be created through Mongo Shell using use <database_name>
command. If the database_name provided to the use command does not exist, a new database is created. Technically, a database is not created until a document is inserted to a collection in the database.
Q25 - How to create a MongoDB Collection?
Once we select a database using use <database_name>
command, to create a collection use db.<collection_name>
to reference a collection. If the collection is already not present, a new Collection is created.
Q26 - How do you explicitly create a MongoDB Collection?
db.createCollection(<collection_name>, [options])
command can be used to create a collection explicitly with the configuration options provided.
Q27 - Which command is used to show all collections in a MongoDB Database?
First we need to select the database. Then we can use show collections
in Mongo Shell to list all MongoDB Collections present in a MongoDB Database.
Q28 - How to delete a MongoDB Collection?
drop()
function can be called on the collection to delete it. Following is the syntax :
db.<collection_name>.drop()
Upon successful deletion, the command echoes back true
to the prompt.
Q29 - What happens when you try delete a Collection that does not exist?
db.<collection_name>.drop()
command returns false
.
Q30 - What is a Document in MongoDB ? Give us an example.
Document is an entity in which zero or more ordered field-value pairs are stored.
Following is a sample MongoDB Document :
{ name: "Robin", age: 23, place: "New York", hobbies: ["Singing", "Reading Books"] }
Q31 - Can Documents be nested ?
Yes. They can. Documents support nesting.