In this tutorial, you shall learn how to define constant properties in a class in PHP, with the help of example programs.
PHP – Class Constants
We can declare constant properties in a class using const keyword.
A property that is declared constant must be initialised in the class definition itself. This constant can never be re-initialised.
In this tutorial, we define a constant in the class and print it to output.
Syntax
The syntax to declare a constant $LEGAL_AGE
, in Person
class is
class Person() {
const $LEGAL_AGE = 18;
}
Please note that we initialised the constant during the declaration.
To access the constant via a class object, use the following syntax.
$classObject::CONSTANT_NAME
For the example of Person
class, the expression would be
$person1::LEGAL_AGE
where $person1
is an object of type Person
.
Examples
1. A simple example for class constant
In this example we take a class Person
and declare a constant $LEGAL_AGE
. We initialise this constant in the declaration itself.
PHP Program
<?php
class Person {
const LEGAL_AGE = 18;
//other properties and methods
}
//create object
$person1 = new Person();
//access constant property
echo $person1::LEGAL_AGE;
?>
Output
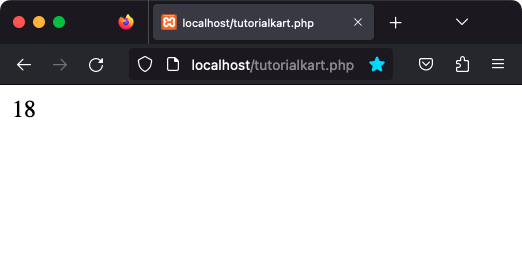
2. Access class constant via class method
In this example we take a class Person
and declare a constant $LEGAL_AGE
. We access this constant from the class method $printLegalAge()
.
PHP Program
<?php
class Person {
const LEGAL_AGE = 18;
public function printLegalAge() {
echo "Legal Age : " . self::LEGAL_AGE;
}
}
//create object
$person1 = new Person();
//access constant property
echo $person1->printLegalAge();
?>
Output
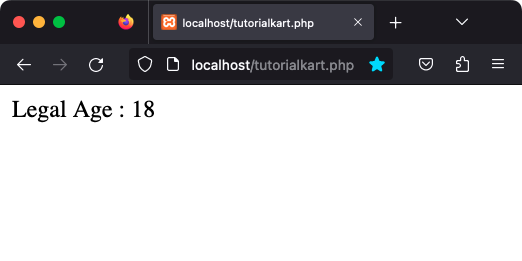
Conclusion
In this PHP Tutorial, we learned how to declare a constant in a class, and how to access it from within the scope, with examples.