In this PHP tutorial, you shall learn how to convert a given string to a float value using typecasting or floatval() function, with example programs.
PHP – Convert string to float
To convert string to float in PHP, you can use Type Casting technique or PHP built-in function floatval().
In this tutorial, we will go through each of these methods and learn how to convert contents of a string to a float value.
Convert String to Float using Type Casting
To convert string to floating point number using Type Casting, provide the literal (float)
along with parenthesis before the string literal. The expression returns float value of the string.
The syntax to type cast string to float number is
$float_value = (float) $string;
Example
In the following example, we will take a string with floating point number content, and convert the string into float using type casting.
PHP Program
<?php $string = "3.14"; $float_value = (float) $string; echo $float_value; ?>
Output
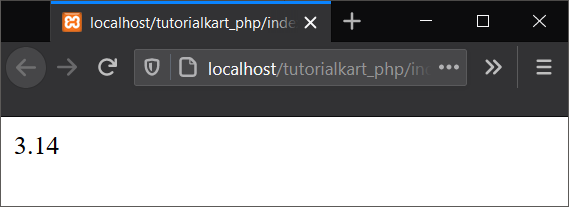
Convert String to Float using floatval()
To convert string to float using PHP built-in function, floatval(), provide the string as argument to the function. The function will return the float value corresponding to the string content.
The syntax to use floatval() to convert string to float is
$float_value = floatval( $string );
Example
In the following example, we will take a string with float content, and convert the string into float value using floatval() function.
PHP Program
<?php $string = "3.14"; $float_value = floatval( $string ); echo $float_value; ?>
Output
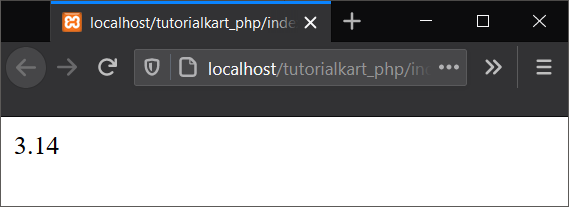
Conclusion
In this PHP Tutorial, we learned how to convert a string to float using typecasting and floatval() function.