In this tutorial, you shall learn how to define a property and a method in a class with the same name in PHP, with the help of example programs.
PHP – Define property and method with the same name in a class
We can define a property and a method in a class with the same name in PHP.
Property or method is a member of class. When a property and a method is defined with the same name, if we access the member as a property then the property is returned, and if we access the member as a method, then the method is called.
The following is a code snippet of a class where we have a property and a method with the same name x
.
class ClassName() {
public $x;
public function x() {
//some code
}
}
Example
In the following program, we take a class Person
and define a property fullname
and method fullname
.
PHP Program
<?php
class Person {
public string $fullname;
public int $age;
public function __construct(string $fullname = null, int $age = null) {
$this->fullname = $fullname;
$this->age = $age;
}
public function fullname() {
echo "<br>Name : " . $this->fullname;
}
}
//create object of class type Person
$person1 = new Person("Mike Turner", 24);
//accessing property of the object
echo $person1->fullname;
//calling method of the object
echo $person1->fullname();
?>
Output
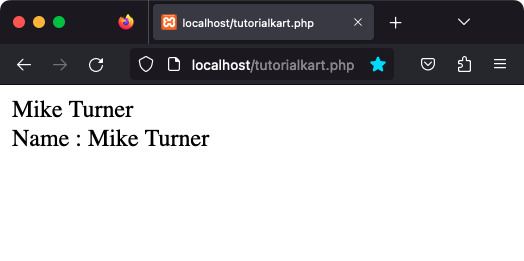
Conclusion
In this PHP Tutorial, we learned how to define a property and a method with the same name in a class, how to access the property or call the method on this class object.