In this tutorial, you shall learn about If-Else conditional statement in PHP, its syntax, and how to use an if-else statement in programs with the help of some examples.
PHP If Else
PHP If Else statement is a conditional statement that can execute one of the two blocks of statements based on the result of an expression.
Syntax
The syntax of PHP If-Else statement is
if (condition) {
// if-block statement(s)
} else {
// else-block statement(s)
}
where
if
is a keyword.condition
is a boolean expression.if-block statement(s)
are a block of statements. if-block can contain none, one or more PHP statements.else
is a keyword.else-block statement(s)
are a block of statements. else-block can contain none, one or more PHP statements.
If the condition evaluates to true, PHP executes if-block statement(s). If the condition is false, PHP executes else-block statement(s).
Example
The following is a simple PHP program to demonstrate the working of If-Else conditional statement.
We take two variables and assign values to each of them. Then we use if-statement to check if the two variables are equal.
Here, the condition is a equals b ?.
PHP Program
<?php
$a = 4;
$b = 4;
if ($a == $b) {
echo "a and b are equal.";
} else {
echo "a and b are not equal.";
}
?>
Output
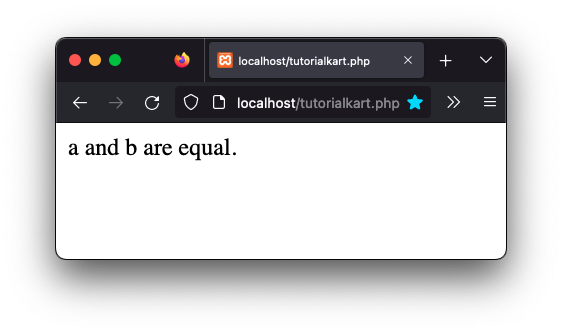
Nested If-Else
if-else itself if a statement just like an echo statement.
Since if-else is a statement, we can write an if-else in the if-block or else-block. If we have an if-else statement inside an if-else statement, it is called nested if-else statement.
In the following PHP program, we write a nested if-else program, to find out the largest of the given three numbers.
PHP Program
<?php
$a = 4;
$b = 1;
$c = 9;
if ($a > $b && $a > $c) {
echo "a is the largest.";
} else {
if ($b > $c) {
echo "b is the largest.";
} else {
echo "c is the largest.";
}
}
?>
Output
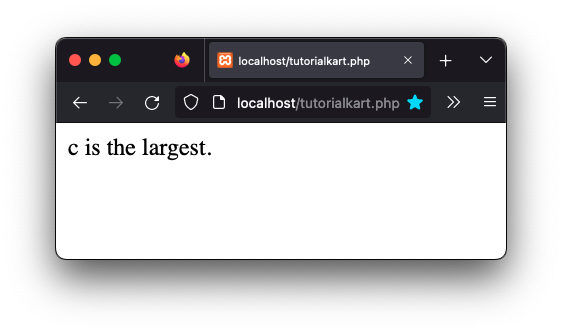
Conclusion
In this PHP Tutorial, we learned about if-else statement, and how to use if-else statement in a PHP program, with the help of examples.