PostgreSQL – ORDER BY Clause
The ORDER BY
clause in PostgreSQL is used to sort the rows of a query result by one or more columns in ascending or descending order.
In this tutorial, we will guide you step-by-step on using the ORDER BY
clause, starting with creating a database and table, inserting data, and then demonstrating various ORDER BY
scenarios.
Step 1: Create a Database
First, create a database named school
to hold the data.
CREATE DATABASE school;
Connect to the database:
\c school;
Step 2: Create a Table
Next, create a table named students
to store information about students.
CREATE TABLE students (
student_id SERIAL PRIMARY KEY,
name VARCHAR(50),
age INT,
grade CHAR(1),
score DECIMAL(5, 2)
);
Step 3: Insert Data
Insert some sample data into the students
table.
INSERT INTO students (name, age, grade, score)
VALUES
('Alice', 14, 'A', 95.5),
('Bob', 15, 'B', 85.3),
('Charlie', 14, 'A', 88.2),
('David', 15, 'C', 72.5),
('Eve', 14, 'B', 91.0);
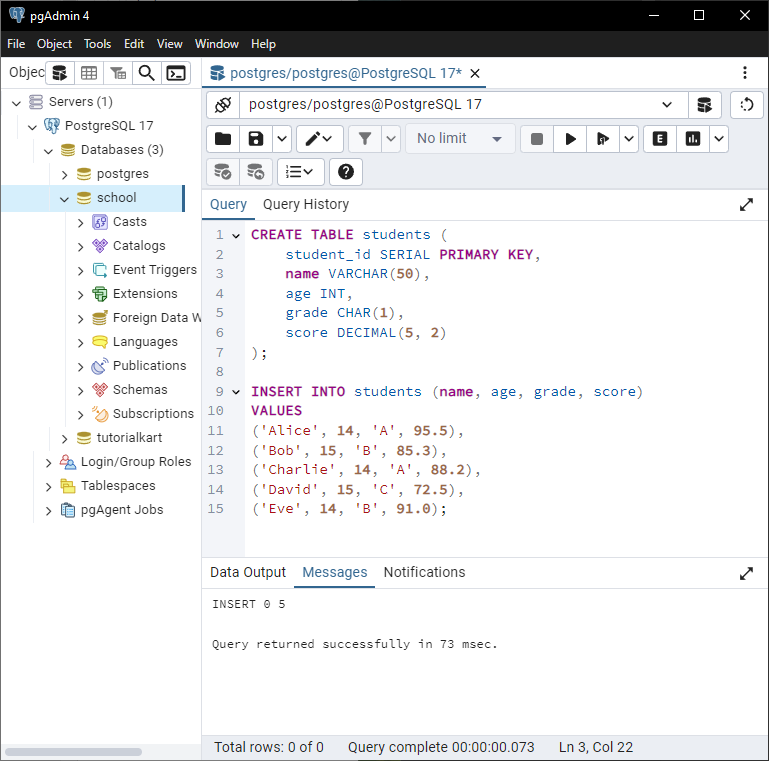
Step 4: Basic Usage of ORDER BY
Retrieve all student records sorted by their scores in ascending order:
SELECT * FROM students
ORDER BY score ASC;
The result will display the students sorted from the lowest to the highest score.
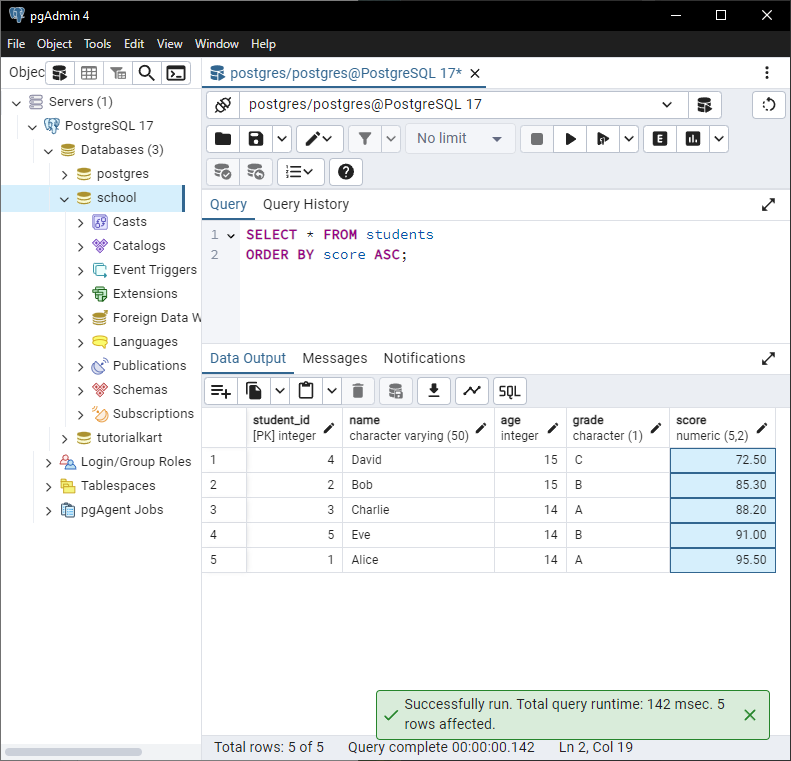
Step 5: Sort in Descending Order
To sort the students by score in descending order:
SELECT * FROM students
ORDER BY score DESC;
This will list the students starting with the highest score.
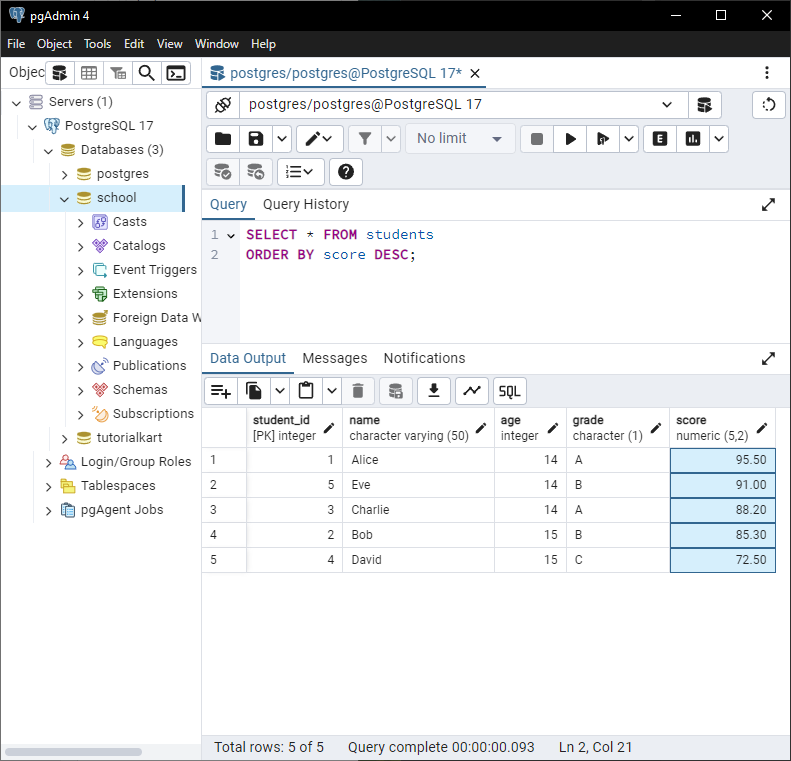
Step 6: Sorting by Multiple Columns
You can sort by multiple columns. For example, to sort by grade
in ascending order and then by score
in descending order:
SELECT * FROM students
ORDER BY grade ASC, score DESC;
This will group students by their grade and within each grade, sort them by their scores in descending order.
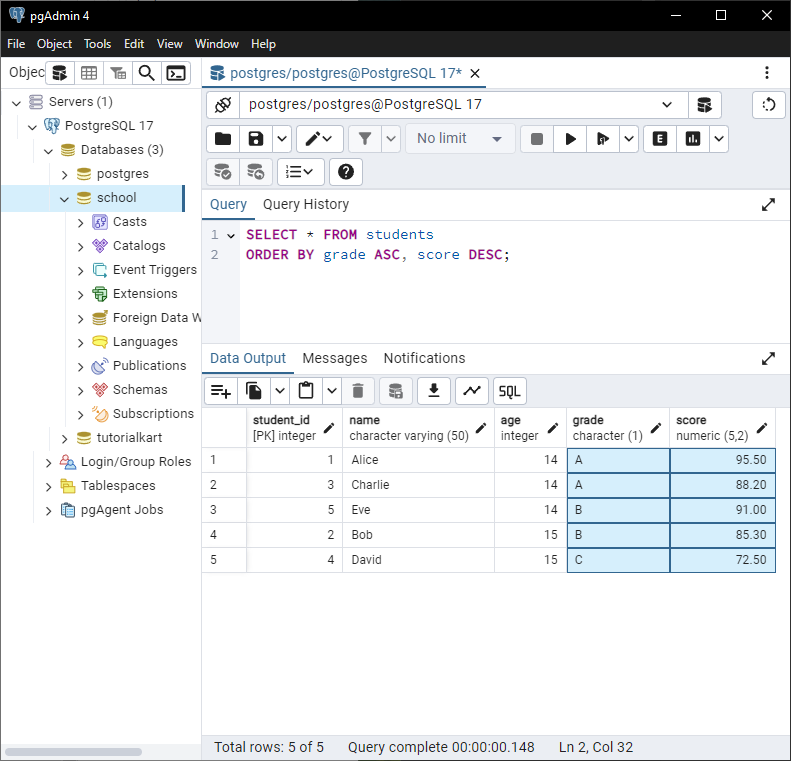
Step 7: Sorting by Alias
Use a calculated column or alias for sorting. For example, sort by score and label it as performance
:
SELECT name, score AS performance
FROM students
ORDER BY performance DESC;
This query sorts the result using the alias performance
.
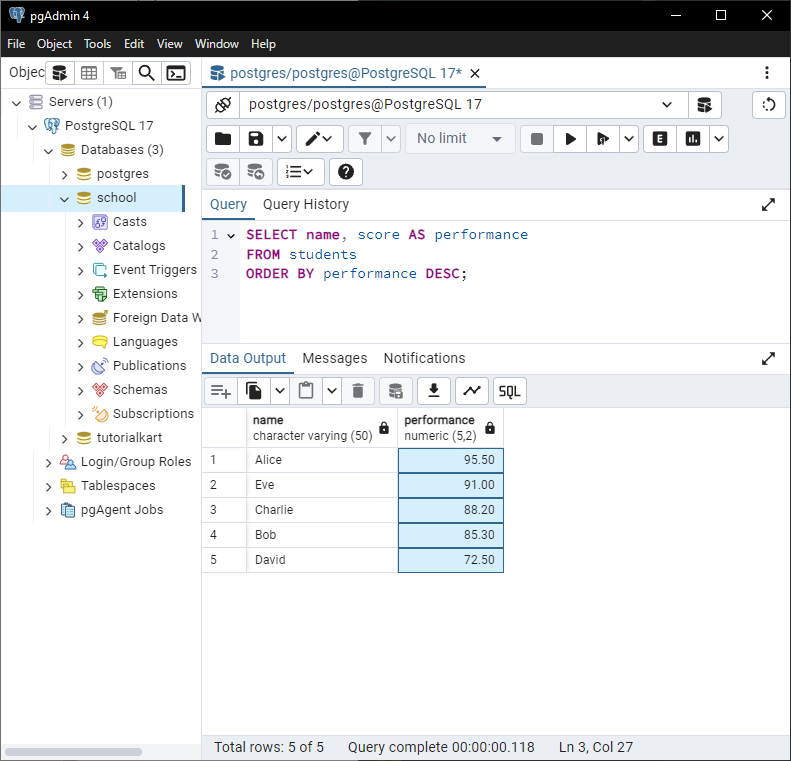
Step 8: NULLS FIRST or NULLS LAST
If the column contains NULL
values, you can specify whether to display them first or last:
SELECT * FROM students
ORDER BY score DESC NULLS LAST;
This ensures that rows with NULL
values in the score
column appear at the end.
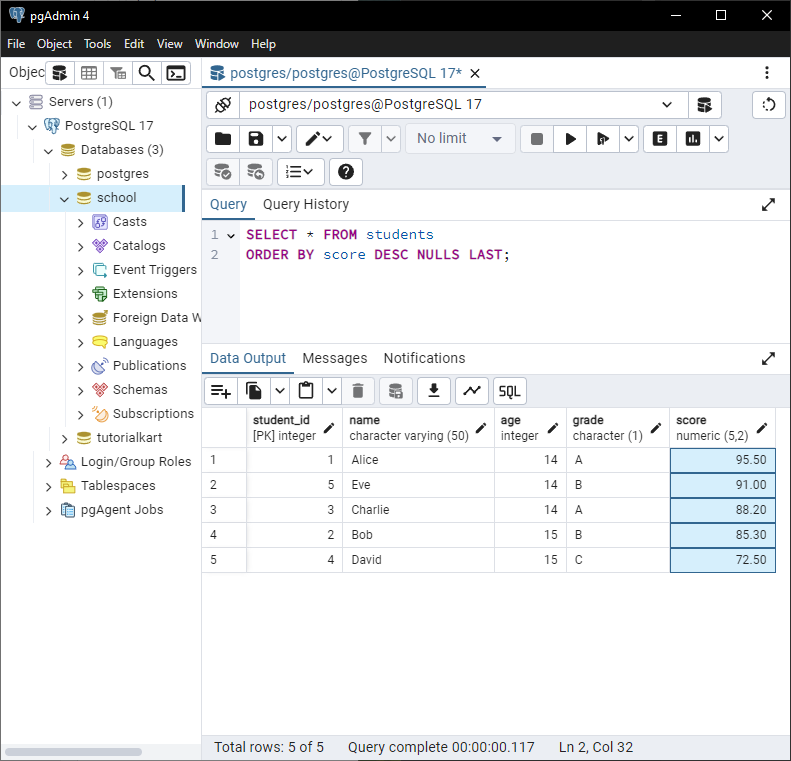
Conclusion
The ORDER BY
clause is a versatile feature in PostgreSQL that allows you to sort query results in various ways. Experiment with these scenarios to better understand its power and flexibility.